Exploring the Latest Features in Java
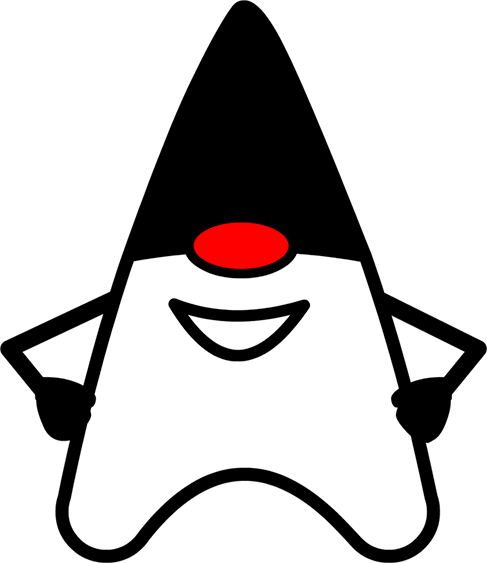
Exploring the Latest Features in Java
Introduction
Java continues to evolve, bringing new features that enhance performance, security, and developer productivity. In this post, we’ll explore some of the most exciting additions to recent Java versions and how they impact modern development.
1. Pattern Matching Enhancements
Pattern matching has been evolving in Java, making code more readable and reducing boilerplate.
Key Updates:
- Pattern Matching for
instanceof
(Introduced in Java 16) - Record Patterns (Preview in Java 19, Improves destructuring of records)
- Switch Pattern Matching (Refined in Java 21)
Example:
if (obj instanceof String s) {
System.out.println(s.toUpperCase());
}
2. Records and Sealed Classes
Records
Records simplify immutable data carriers, reducing boilerplate associated with traditional Java beans.
public record Person(String name, int age) {}
Sealed Classes
Sealed Classes (Introduced in Java 17) allow better control over class hierarchies:
sealed class Shape permits Circle, Rectangle {}
final class Circle extends Shape {}
final class Rectangle extends Shape {}
3. Virtual Threads (Project Loom)
Introduced in Java 21, Virtual Threads revolutionize concurrency by enabling lightweight thread creation, reducing resource consumption.
try (var executor = Executors.newVirtualThreadPerTaskExecutor()) {
executor.submit(() -> System.out.println("Hello from a virtual thread"));
}
4. Foreign Function & Memory API
Java has improved interoperability with native code using the Foreign Function & Memory API.
Use Case:
Interfacing with C libraries without JNI complexity.
try (Arena arena = Arena.ofConfined()) {
MemorySegment segment = arena.allocate(100);
}
5. String Templates (Preview in Java 21)
String Templates simplify concatenation and formatting:
String name = "Alice";
String greeting = STR."Hello, \{name}!";
Java future
Java’s evolution is driven by the Java Enhancement Proposal (JEP) process, which introduces new features and improvements to the language and its runtime environment. As of January 2025, several JEPs are in development, aiming to enhance Java’s performance, security, and developer experience.
Project Valhalla: Value Objects
One of the most anticipated initiatives is Project Valhalla, which focuses on introducing value objects to Java. Value objects are instances that lack identity and can offer improved memory layout and performance benefits. This enhancement aims to provide developers with more efficient data structures and operations.
Project Panama: Foreign Function & Memory API
Project Panama aims to improve Java’s interoperability with native code. The Foreign Function & Memory API, introduced under JEP 454, enables Java programs to call native libraries and handle native memory more efficiently and safely. This feature is expected to be stabilized in Java 22.
Project Loom: Virtual Threads
Project Loom introduces virtual threads to the Java platform, aiming to simplify concurrent programming by providing lightweight threads that can handle numerous tasks with minimal resource overhead. This enhancement is expected to improve the scalability and performance of Java applications.
Project Amber: Pattern Matching and Records
Project Amber focuses on enhancing Java’s syntax and language features to improve developer productivity. Recent JEPs under this project include pattern matching for switch statements and the introduction of records, which provide a compact syntax for declaring classes that are primarily used to store data.
Project Leyden: Reducing Startup Time
Project Leyden aims to address Java’s startup time and performance by introducing static images and ahead-of-time compilation. This project seeks to make Java applications start faster and consume fewer resources, which is particularly beneficial for cloud and microservices architectures.
These ongoing efforts reflect the Java community’s commitment to evolving the platform to meet modern development needs. Developers are encouraged to stay informed about these proposals and participate in discussions to shape the future of Java.
Conclusion
These new features in Java improve performance, readability, and efficiency, making Java more modern and developer-friendly. Keeping up with these updates ensures better coding practices and optimized applications.