Exploring the New Features in Python 3.12
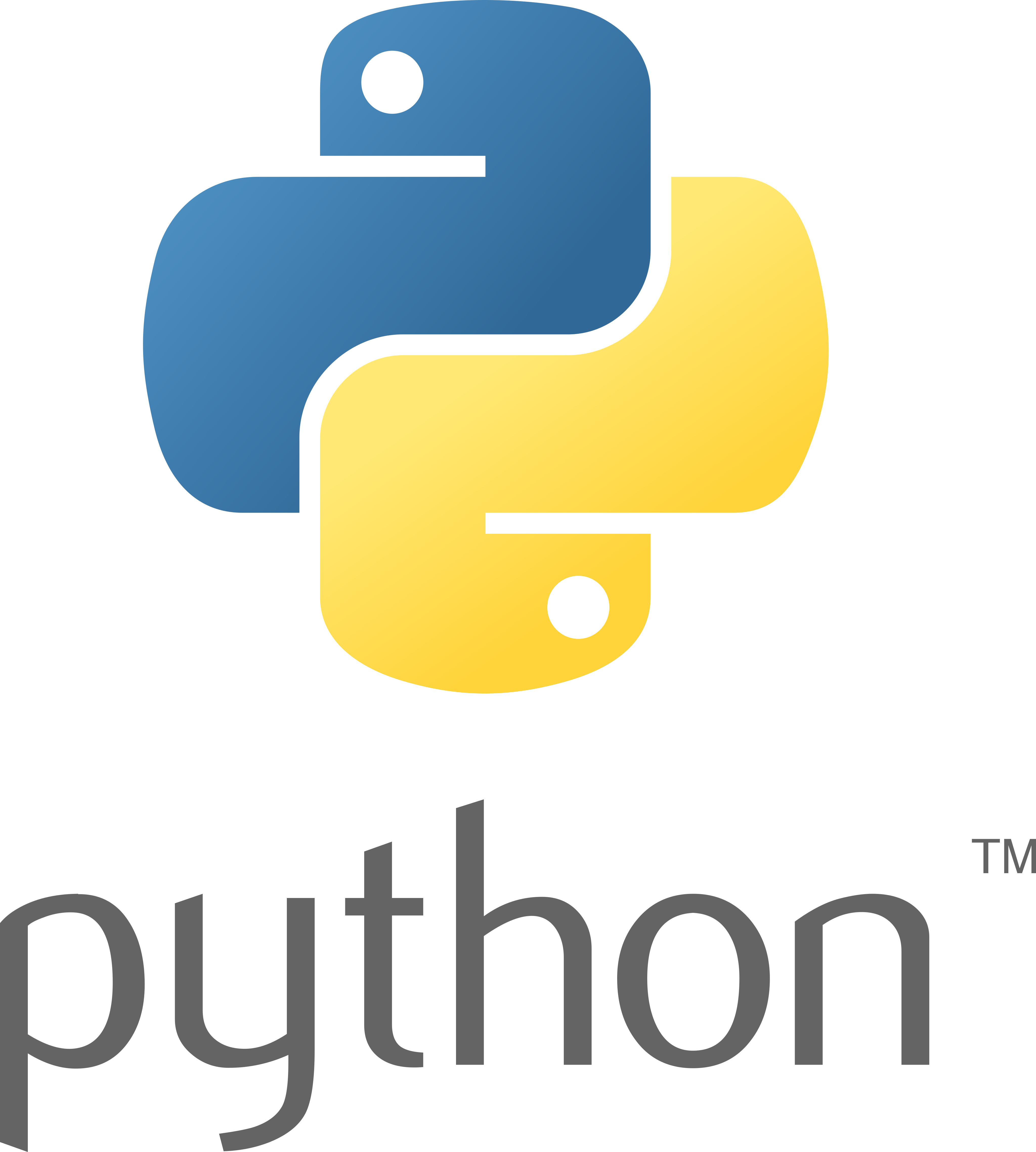
Exploring the New Features in Python 3.12
Python 3.12 introduces a range of enhancements aimed at improving performance, developer experience, and language capabilities. In this post, we’ll delve into some of the most notable features of this release.
Immortal Objects
In Python 3.12, certain immutable singleton objects, such as None
, True
, and False
, are now treated as immortal. This means their reference counts no longer change, leading to better cache behavior and simplifying future interpreter optimizations. For instance:
import sys
print(sys.getrefcount(None)) # Outputs: 4294967295
The reference count for None
is set to a special flag value (4294967295
), indicating its immortality. This change enhances performance and prepares the interpreter for future developments. (realpython.com)
Improved Static Typing Syntax
Python 3.12 introduces a more concise syntax for defining generic classes and functions. You can now declare type variables directly within the class or function definition:
class Queue[T]:
def __init__(self) -> None:
self.elements: list[T] = []
def push(self, element: T) -> None:
self.elements.append(element)
def pop(self) -> T:
return self.elements.pop(0)
This new syntax eliminates the need to import and declare type variables separately, resulting in cleaner and more readable code. (realpython.com)
More Informative Error Messages
Building upon previous improvements, Python 3.12 provides even more descriptive error messages, making debugging more straightforward. For example, a mismatched quotation mark in a print statement will now generate an error message indicating the probable cause and suggesting a correction, helping developers quickly identify and fix simple mistakes. (javacodegeeks.com)
Self
Type Hint in Class Methods
A new addition to the typing
module is the Self
type hint, which allows for more precise type annotations in methods that return an instance of the class:
from typing import Self
class Shape:
def set_sides(self, sides: int) -> Self:
self.sides = sides
return self
This enhancement improves readability and type hinting accuracy, particularly in complex inheritance structures. (javacodegeeks.com)
Enhanced Pattern Matching
The match
statement, introduced in Python 3.10, receives valuable improvements in Python 3.12, making pattern matching more versatile, especially with extended support for custom types and keyword patterns. For example:
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
shape = Rectangle(10, 20)
match shape:
case Rectangle(width=10, height=h):
print(f"Found a rectangle with height {h}")
With enhanced match
and case
support, you can now create more dynamic patterns that match based on attributes, making code more expressive. (javacodegeeks.com)
Performance Improvements
Python 3.12 includes several under-the-hood optimizations:
- Faster Exception Handling: Improved performance in exception-handling code paths leads to quicker error processing.
- Memory Efficiency: Enhancements in memory usage benefit applications dealing with large datasets.
The Future of Python: What’s Next for the World’s Favorite Language?
Python has remained one of the most popular programming languages for years, powering everything from web development to data science and AI. With Python 3.x now well-established, the Python community continues to push forward with innovations aimed at improving performance, security, and developer experience. In this post, we’ll explore what’s next for Python, including ongoing projects, upcoming PEPs (Python Enhancement Proposals), and the broader direction of the language.
1. Performance Enhancements with CPython
Python’s performance has often been criticized compared to lower-level languages like C++ or Rust. However, with the Shannon Plan, spearheaded by Guido van Rossum and the Python Steering Council, significant optimizations are underway to make Python faster.
Key Initiatives:
- Faster CPython (PEP 659): The goal is to improve Python’s speed by at least 2x in future releases.
- Adaptive Specialization: More efficient bytecode execution by optimizing common code paths.
- Efficient Memory Management: Reducing memory overhead in object allocations.
Expect these improvements to make Python even more suitable for high-performance applications.
2. Pattern Matching Evolution
Introduced in Python 3.10, Structural Pattern Matching (PEP 634) was a major language addition, allowing developers to write more readable and expressive code.
Future Enhancements:
- Support for richer data structures in match statements.
- More efficient compilers to optimize pattern matching performance.
- Expansion of match-case syntax to cover additional scenarios.
This feature is still evolving, and upcoming versions may further refine its capabilities.
3. Improvements in Type Hinting and Static Typing
With large-scale applications, type safety and static analysis have become more critical. Python’s typing module is evolving rapidly to enhance maintainability and developer productivity.
Future Plans:
- Better Type Inference: Reducing the need for explicit annotations.
- Refinement of
TypedDict
and Protocols for more expressive type hints. - Performance Boost for Type Checking with Just-in-Time (JIT) compilation for type analysis.
These improvements make Python more appealing for enterprise-scale applications.
4. The GIL Removal Initiative
Python’s Global Interpreter Lock (GIL) has long been a bottleneck for multi-threaded performance. PEP 703 proposes making Python GIL-free, unlocking true multi-core parallelism.
Expected Benefits:
- Improved multi-threading for CPU-bound tasks.
- Enhanced support for parallel processing.
- More efficient execution of AI/ML workloads.
If successful, this could mark one of the most significant changes in Python’s history.
5. Better Support for WebAssembly and Mobile Development
Python’s ecosystem is expanding beyond traditional server-side applications, with efforts to improve WebAssembly (WASM) and mobile support.
Key Developments:
- Python on WebAssembly (Pyodide): Running Python directly in the browser.
- Mobile Optimizations: Efforts to make Python more efficient on iOS and Android.
- Lightweight Runtimes: Creating smaller, more portable Python distributions.
This shift could bring Python to new domains, including mobile apps and browser-based applications.
6. Enhanced AI and Data Science Capabilities
With Python’s dominance in AI and data science, expect further optimizations and integrations.
Future Trends:
- Better NumPy/Pandas Performance through native accelerators.
- Integration with Rust and C++ for high-speed computing.
- Optimized Machine Learning Libraries such as TensorFlow and PyTorch with deeper Python integration.
These enhancements will solidify Python’s role in AI and big data processing.
7. Expanding Python’s Ecosystem
Beyond the core language, Python’s packaging and dependency management tools are improving.
Upcoming Enhancements:
- PEP 704: Simplifying Python packaging with better dependency resolution.
pip
andvenv
Improvements for faster and more reliable package management.- Containerized Python Environments for better reproducibility in development and deployment.
These features will make Python development smoother, especially for teams and large projects.
Conclusion
Python 3.12 brings significant advancements that enhance performance, readability, and developer productivity. By adopting these new features, developers can write more efficient and maintainable code, further solidifying Python’s position as a versatile and powerful programming language.
Python’s future is incredibly exciting, with performance optimizations, new language features, and expanded use cases. From GIL removal to enhanced AI capabilities, the language is evolving to meet the demands of modern software development. As Python continues to grow, developers can look forward to a faster, more scalable, and more versatile language in the coming years.
Stay tuned for Python’s next major releases and keep experimenting with the latest features to stay ahead in the world of programming!