Exploring the Latest Features in C#
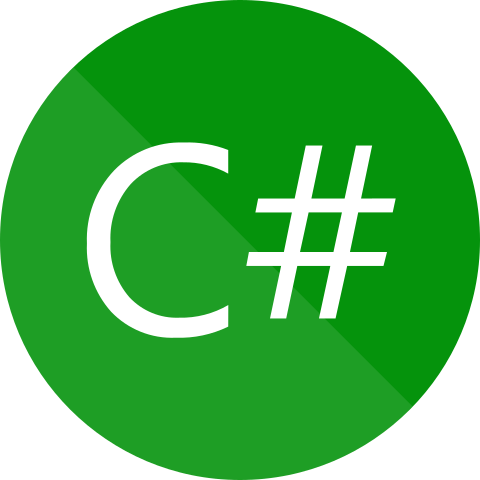
Exploring the Latest Features in C#
Introduction
C# continues to evolve, bringing new features that enhance performance, security, and developer productivity. In this post, we’ll explore some of the most exciting additions to recent C# versions and how they impact modern development.
Past two years
Over the past two years, the C# programming language has seen significant advancements with the release of versions 12 and 13, each introducing features aimed at enhancing developer productivity and code efficiency.
C# 12 (November 2023):
Released in conjunction with .NET 8, C# 12 brought several notable features:
-
Primary Constructors: This feature allows developers to define constructor parameters directly within the class declaration, streamlining the initialization process.
-
Collection Expressions: Simplifying the creation and manipulation of collections, this addition enhances code readability and reduces boilerplate code.
-
Inline Arrays: Developers can now define arrays directly within expressions, leading to more concise and readable code.
-
Optional Parameters in Lambdas: This enhancement allows lambda expressions to have optional parameters, increasing their flexibility and usability.
C# 13 (November 2024):
Building upon its predecessors, C# 13, released alongside .NET 9, introduced the following features:
-
Params Collections: The
params
modifier is no longer limited to array types; it can now be used with any recognized collection type, includingSpan<T>
and interface types, providing greater flexibility in method parameter definitions. -
New Lock Type and Semantics: If the target of a
lock
statement is aSystem.Threading.Lock
, the compiler generates code to use theLock.EnterScope()
method to enter an exclusive scope, offering more granular control over synchronization. -
Field Keyword (Preview Feature): The introduction of the
field
keyword allows for more explicit references to backing fields within property accessors. It’s important to note that this is a preview feature in C# 13 and requires enabling the preview language version in your project settings. (learn.microsoft.com)
C# enhancements
These enhancements reflect Microsoft’s commitment to evolving C# in ways that address modern development needs, emphasizing simplicity, performance, and developer productivity.
1. Record Types
C# introduced record types to simplify immutable data structures and reduce boilerplate code.
public record Person(string Name, int Age);
Records provide built-in value-based equality and with-expressions for easy modifications.
2. Pattern Matching Enhancements
Pattern matching has been significantly improved in recent C# versions.
Key Updates:
- Relational Patterns
- Logical Patterns (and, or, not)
- Type Patterns with
switch
Expressions
Example:
int number = 42;
string result = number switch
{
> 0 => "Positive",
< 0 => "Negative",
_ => "Zero"
};
3. File-Scoped Namespaces
A cleaner way to define namespaces without unnecessary indentation.
namespace MyApp;
class Program
{
static void Main() => Console.WriteLine("Hello, C#");
}
4. Required Properties
Introduced in C# 11, required properties enforce initialization of certain fields.
public class User
{
public required string Name { get; init; }
}
5. Null Parameter Checking
C# 11 introduces a concise way to enforce non-null parameters.
public void PrintMessage(string message!!)
{
Console.WriteLine(message);
}
6. Raw String Literals
C# 11 adds raw string literals, making it easier to define multi-line strings.
string json = """
{
"name": "Alice",
"age": 30
}
""";
7. Performance Improvements with Span<T>
C# continues to improve memory efficiency with Span<T> and Memory<T>.
Span<int> numbers = stackalloc int[] { 1, 2, 3, 4, 5 };
Conclusion
These new features in C# improve performance, readability, and efficiency, making C# more modern and developer-friendly. Keeping up with these updates ensures better coding practices and optimized applications.