Lisp
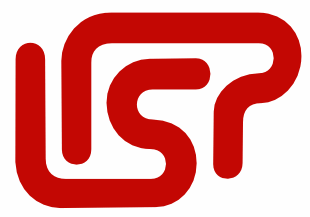
Introduction
Lisp is a programming language developed in 1958 by John McCarthy at the Massachusetts Institute of Technology (MIT). Based on his calculation, it is the second-oldest high-level programming language still in use today. Lisp stands for LISt Processor, and the language is built primarily around list manipulation. It has more data types and more sophisticated functions than most other programming languages, making it suitable for artificial intelligence research and development.
History
Lisp was first presented in a paper by John McCarthy at the ACM Turing Award Lecture in 1962. This paper, entitled “Recursive Functions of Symbolic Expressions and Their Computation by Machine”, introduced the concepts of recursion and symbolic operations, revolutionizing the way programs could interact with data. In 1965, Steve Russell and Timothy Hart wrote the first Lisp interpreter, which was used to run the first Lisp programs. Lisp found early success in the artificial intelligence community, where its ability to manipulate data structures made it well suited for AI applications. The most popular Lisp dialects during this period were MacLisp and I-Lisp. In the 1980s, Lisp’s popularity increased with the advent of Lisp machines, dedicated computers that implemented Lisp as their native language. Several commercial Lisp implementations such as Franz Inc.’s Allegro Common Lisp and Xanalys’ LispWorks were released during this period. In the 1990s, Lisp experienced a revival with the introduction of new Lisp dialects such as Common Lisp and Scheme. Common Lisp was an effort to promote standardization among Lisp implementations, while Scheme was a smaller dialect intended for teaching and experimentation. Common Lisp and Scheme are still in use today, and are popular for tasks ranging from web development to scientific computing.
Early History
Lisp began as an exercise in refinement, by removing unnecessary features from Fortran and using Polish prefix notation. Its architecture of nested expression was subject to continual evolution until it reached its modern goal of list processing. In the 1960s and 1970s, various dialects of Lisp were used in artificial intelligence (AI) research, including the original Maclisp, Interlisp, and Standard Lisp. AI researchers found Lisp to be very useful for their needs, due to its powerful symbolic processing capabilities. In the early 1980s, Lisp suffered a setback when AI research dried up because of funding cutbacks. However, the language continued to be popular among academics and hobbyists. In 1985, an effort was made to standardize Lisp through the Common Lisp standardization process. This effort was successful and the Common Lisp language became a widely used standard for Lisp development and implementation.
Modern Usage
Today, Lisp is still used in research and development of artificial intelligence (AI) systems. Lisp is also used in a variety of other areas such as bioinformatics, machine learning, natural language processing, and graph theory. It is also used in web development, software engineering, and various other fields. Lisp is a dynamically typed, purely functional language. It has a unique macro system, allowing users to create their own syntactic abstractions. This makes Lisp an ideal language for creating applications that require high levels of expressiveness and extensibility. The syntax of Lisp is simple and minimalist, making it easy for novice programmers to learn and use. Lisp programs are also highly portable, allowing them to be deployed on different platforms with relative ease.
Usages
Lisp is widely used in artificial intelligence research and development, where its ability to manipulate data structures gives it an advantage over other programming languages. Lisp’s flexibility also makes it useful for rapid prototyping and software development. Other uses of Lisp include web development, scientific computing, machine learning, image processing, and pattern recognition. Lisp is also used in game development, with several well-known games such as Doom and Quake being written in Lisp. Additionally, Lisp is popular in robotics research, where its ability to easily manipulate data structures is ideal for tasks such as sensor fusion.
Benefits
Lisp is an incredibly powerful language due to its flexibility and strength in manipulating data and AI algorithms. In particular, Lisp has several key benefits that make it ideal for creating AI and machine learning applications. First, Lisp includes several built-in functions that make it easier to write code and manipulate data, such as garbage collection, dynamic typing, and macros. This makes it easier for developers to create complex algorithms and applications quickly and efficiently. Second, Lisp is highly extensible, meaning that developers can add new features or modify existing code without having to rewrite the entire application. This makes it easy for developers to experiment with different ideas and to quickly adjust their code as needed. Finally, Lisp includes many libraries and packages that help developers create powerful AI applications and algorithms more easily. These libraries are often open-source and free to use, making them accessible to everyone.
Adoption
Lisp is used in many different fields and applications, ranging from basic AI research to complex machine learning applications. Some of the most common examples of Lisp applications include:
- Natural language processing: Lisp is often used to create programs that can understand natural language and convert it into a format computers can interpret.
- Robotics: Lisp is used to create programs for controlling robots, allowing them to navigate and interact with their environment.
- Image recognition: Lisp is used to create programs that can identify objects in images and videos, such as faces, letters, and numbers.
- Machine learning: Lisp can be used to create algorithms that can learn from data and improve over time.
Code Examples
Here is an example of a simple program written in Common Lisp:
(defun hello-world ()
(print "Hello, world!"))
(hello-world)
This program will print “Hello, world!” when it is executed. Here is an example of a more complex program written in Scheme:
(define (factorial n)
(if (= n 0)
1
(* n (factorial (- n 1)))))
(display (factorial 4))
This program calculates the factorial of 4 and prints the result on the screen (24).
#Data Structures
One of the strengths of Lisp is its rich set of data structures. Lisp provides support for lists, vectors, hash tables, trees, and other abstract data types. Lisp also supports a wide range of functions built-in to the language, allowing programmers to quickly manipulate data structures.
In Lisp, lists are the primary data structure. Lists can contain any type of Lisp object, including numbers, strings, symbols, and other lists. Lists can be constructed using the cons
special operator. For example, the following code creates a list containing the numbers 1, 2, and 3:
> (cons 1 (cons 2 (cons 3 nil)))
(1 2 3)
Lists can then be manipulated using a variety of functions, such as map
, filter
, and reduce
. For example, the following code applies the function square
to each element in the list:
> (map square '(1 2 3))
(1 4 9)
Vectors are another type of data structure supported by Lisp. Vectors are similar to lists, but they contain elements of a fixed size. Vectors can be created using the vector
special operator. For example, the following code creates a vector containing the numbers 1, 2, and 3:
> (vector 1 2 3)
#(1 2 3)
Additionally, Lisp provides support for associative data structures, such as hash tables and trees. Hash tables are key-value stores that allow efficient lookup of values based on keys. Trees are hierarchical data structures that can be used to represent large amounts of data in an organized manner.
Below are some examples of code written in Lisp:
Example 1: ```(defun my-func () (princ “Hello World!”))
(my-func)
Output:
Hello World!
Example 2:
```(defun summation (list)
(if (null list)
0
(+ (car list)
(summation (cdr list)))))
(summation '(1 2 3 4 5))
Output:
15
Lisp is widely used in AI, particularly in the creation of expert systems and prediction algorithms. Lisp is also used in natural language processing, where it is used to create programs that can understand and generate human language.
In web development, Lisp is used to create programs that optimize HTML and JavaScript. It is also used in databases, where it is used to create sophisticated queries.
Finally, Lisp is used in computer graphics for creating sophisticated graphics algorithms. Since Lisp is a functional language, it is well-suited for the task of manipulating data structures and images in a variety of ways.
Code Examples
Here is an example of a simple Lisp program that prints out the numbers from one to ten:
(defun print-nums (n)
(if (= n 10)
(print 'done)
(progn
(print n)
(print-nums (+ 1 n)))))
(print-nums 1)
This program defines a function called print-nums
, which takes a number n
as an argument. The function then prints the number n
and calls itself recursively with the argument incremented by one. Once the argument reaches 10
, done
is printed.
The following Lisp code snippet implements a simple factorial function:
(defun factorial (n)
(if (= n 0)
1
(* n (factorial (- n 1)))))
(print (factorial 5))
This code defines a function factorial
which takes a number n
as an argument and returns the factorial of n
. The function uses a recursive algorithm to calculate the factorial of n
. If n
is zero, then the function returns 1
, otherwise it multiplies n
by the factorial of n-1
.
Below is a simple example of a Lisp program that can print out each item in a list:
(let ((my-list '(1 2 3 4 5)))
(dolist (item my-list)
(print item)))
The code above will print out each item in the list, one at a time. This code shows how Lisp can be used to easily manipulate lists and iterate over them.
Conclusion
Lisp is a powerful and flexible programming language with a long history of successful applications in artificial intelligence and robotics research. Its ability to manipulate data structures makes it well-suited for tasks such as machine learning, image processing, and pattern recognition. Additionally, its wide range of data structures and powerful built-in functions make it an ideal choice for rapid prototyping and software development. With its wide array of features, Lisp is an excellent choice for programming projects in any domain. Lisp is an incredibly powerful programming language with a long and rich history. Its unique features make it ideal for creating AI and machine learning applications, and its extensibility allows developers to quickly and easily experiment with new ideas. With its wide range of applications and libraries, Lisp is an essential programming language for any aspiring AI developer.