Java
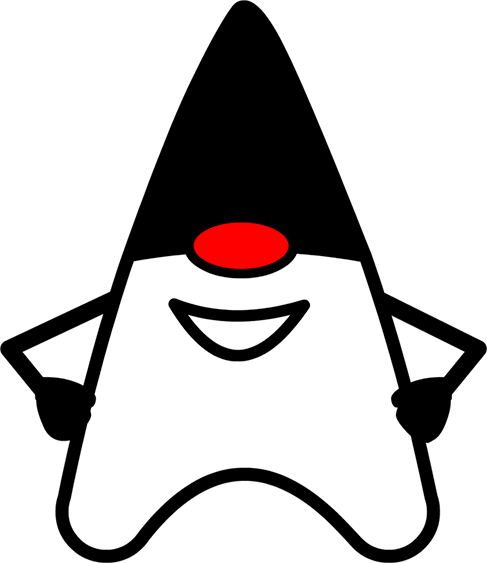
Introduction to Java
Java was created in 1995 by James Gosling at Sun Microsystems. Its initial release came with the Java 1.0 compiler shortly afterwards. The language was quickly adopted and became the de-facto standard for building web applications. In 1997, Sun announced the “Java Community Process” which allowed anyone to contribute ideas to the language, and eventually led to the creation of the Java Standard Edition (SE). In 2001, the language was rebranded as “Java 2 Platform, Standard Edition (J2SE)” and became the language of choice for developers. Since then, newer versions of the language have been released, with each version introducing features and improvements that make it more powerful and secure.
Java is a programming language developed by Sun Microsystems in 1995. It was designed to be simple yet powerful, with the goal of being portable across multiple platforms. It has since become one of the most popular programming languages, used for everything from web applications to mobile development. In this article, we will explore the fundamentals of Java, its history, and some examples of how it can be used. Java is a programming language and computing platform that was released by Sun Microsystems in 1995. It is one of the most popular programming languages in use today and has been used to develop countless applications, websites, games, and software. Java is an object-oriented language, which means it works by creating objects, or data structures, and relating them to each other in order to accomplish tasks or build complex systems or applications. The Java is a collection of pre-built classes and other tools that are provided by the Java development environment. These classes provide functionality such as input/output (I/O), collections, networking, and windowing. This makes it possible for developers to quickly create programs and applications in Java without having to write all of the code from scratch. In this article, we’ll discuss the basics of the Java, its history, and examples of how it can be used. We’ll also take a look at some code examples to illustrate how the works.
History of the Java
The Java was first released with Java 1.0 in 1996. It was created to simplify the development of programs and applications in Java. At the time of its release, Java was a relatively new language and the Java was seen as an essential component for developing in Java due to its range of features and pre-defined classes. Since its initial release, the Java has been refined and enhanced to include a larger selection of classes, tools, and features. The release of Java 5 in 2004 saw the introduction of generics, which allowed for the use of more abstracted data types, such as “list” or “map”. Generics revolutionized the way objects were created and stored, and made it easier to manage data structures. In 2009, Oracle acquired Sun Microsystems, the original creators of Java, and the Java has seen continuous updates and improvements ever since. Today, the Java includes over three thousand API packages, which can be used to develop a wide range of applications and programs.
Adoption
The Java has a wide range of uses, and can be used to create anything from simple programs to complete applications. Here are some examples of how the can be used:
- Networking - The Java provides classes and tools to help with creating networked applications. Its networking capabilities allow developers to create applications that can communicate between separate clients or servers.
- Data Storage and Management - The Java provides classes that make it easy to store and manage data. These classes can be used to store information in databases, access files, and manage memory.
- GUI Development - The Java includes several classes for developing graphical user interfaces (GUI) for applications. These classes provide developers with tools for creating menus, windows, dialog boxes, and other graphical elements.
- Image Processing - The Java includes several classes for manipulating images. These classes provide developers with the ability to resize, crop, and otherwise alter image files.
- Games Development - The Java includes several classes for developing video games. These classes can be used to create 3D graphics, animations, and provide other game development tools.
Examples of Usage
Web Development
The Java can be used to create robust web applications. As web development has become increasingly complex and feature-rich, the Java has evolved to support these needs. The Java contains a number of packages specifically designed for web development, such as servlets, Java Server Pages (JSPs), tag libraries, and more. Developers can utilize these packages to create dynamic web applications with Java.
GUI Development
The Java can also be used to create graphical user interfaces (GUIs). This means that developers can create desktop applications with attractive user interfaces and rich functionality with the help of the Java . The contains a number of packages specifically geared towards GUI development, including the Swing and AWT packages.
Database Access
The Java also provides access to database systems. Developers can utilize the JDBC API to access relational databases such as Oracle, MySQL, and others. The JDBC API provides developers with an easy way to connect to database systems and execute queries in an efficient manner.
XML Processing
The Java contains a number of packages for working with extensible markup language (XML). Developers can use the Java API for XML Processing (JAXP) to parse, manipulate, and generate XML documents. The Java XML parsers can be used to read and write XML documents.
Networking
The Java contains a number of packages for working with network protocols. The java.net package provides developers with a set of classes and interfaces for accessing the Internet. The package includes classes for working with URLs, HTTP connections, TCP/IP sockets, IP multicast groups, and more.
Security
The Java also includes a number of security-related packages. The java.security package is especially useful for developers who need to create applications that are secure. The package includes classes for working with digital signatures, message digests, key management, and encryption.
Code Examples
Here is a code example of how to use the Java to read from an XML document:
Here are some code examples that demonstrate how the Java can be used.
Print Output:
public class Test {
public static void main(String[] args) {
System.out.println("Hello World!");
}
}
The above code will print out the string “Hello World!” to the console. The System.out.println() method is used to print output to the console, and is part of the Java .
Network Connection:
import java.net.*;
public class Test {
public static void main(String[] args) {
try {
URL url = new URL("http://www.example.com");
HttpURLConnection connection = (HttpURLConnection)url.openConnection();
connection.connect();
System.out.println("Connected!");
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
}
The above code will attempt to open a connection to the specified URL. The URL and HttpURLConnection classes are both part of the Java and are used to establish a network connection. If the connection is successful, the program will print out “Connected!” to the console.
A basic code example in Java is as follows:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello World!");
}
}
This code will print “Hello World!” to the console.
To demonstrate a more advanced example, consider the following code that creates a basic window application using the Swing toolkit:
import javax.swing.*;
public class MyWindowApp {
public static void main(String[] args) {
JFrame frame = new JFrame("My Window Application");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300,200);
frame.setVisible(true);
}
}
When executed, this code will open a window with the title “My Window Application” and size 300 by 200 pixels.
// import the necessary classes
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.DocumentBuilder;
import org.w3c.dom.Document;
import org.w3c.dom.NodeList;
import org.w3c.dom.Node;
import org.w3c.dom.Element;
// create a DocumentBuilderFactory
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
// create a DocumentBuilder object and parse the file
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(new File("data.xml"));
// get the root element
Element root = document.getDocumentElement();
// get all the nodes in the document
NodeList nodeList = root.getChildNodes();
// loop through the nodes
for (int i = 0; i < nodeList.getLength(); i++) {
// get the node
Node node = nodeList.item(i);
// if it is an element then process it
if (node.getNodeType() == Node.ELEMENT_NODE) {
// print out the element name
System.out.println("Element Name: " + node.getNodeName());
// get the element's attributes
NamedNodeMap attributes = node.getAttributes();
// loop through the attributes
for (int j = 0; j < attributes.getLength(); j++) {
// get the attribute
Attr attribute = (Attr) attributes.item(j);
// print out the attribute name and value
System.out.println("Attribute Name: " + attribute.getName());
System.out.println("Attribute Value: " + attribute.getValue());
}
}
}
Java is a versatile language, and can be used to create a wide range of software applications. It is the preferred language for developing web applications as it is more secure than other languages. Additionally, it is also used for mobile applications, server-side applications, scientific software, and game development.
Java has also become popular for creating artificial intelligence (AI) and machine learning (ML) applications. Many major companies such as Google and Facebook use Java for their AI projects.
Below is a basic “Hello World” example written in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
This example will output the text “Hello, World!” when it is run.
To execute this code, you must compile it into an executable format. You can do this by using the command-line tool javac
. Once it has been compiled, you can then run the executable file using the command java
.
Conclusion
Java has become one of the world’s most popular programming languages due to its versatility and portability. It is used for everything from web applications to artificial intelligence projects, and is a powerful and secure language for developing software. We hope that this introduction has given you a better idea of what Java is and how it can be used.