CouchDB
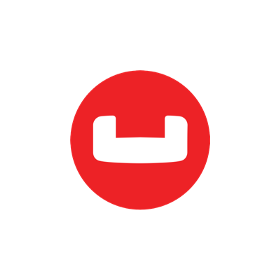
Introduction to CouchDB
CouchDB is an open-source document-oriented database written in the Erlang programming language. It was created by Damien Katz in 2005 and released under the Apache License. CouchDB stores data in JSON documents, and allows users to access, query, and manipulate the data through a RESTful HTTP API. The same API can also be used to view and modify documents directly from any web browser. CouchDB is often used as a tool for large-scale data storage, synchronization, and replication applications. It’s popular among mobile developers and web application developers who need to store and sync their data between different devices, services, or platforms.
History of CouchDB
CouchDB was first released in 2005 by Damien Katz. Initially, the database was known as Lotus Notes Cloud Database, but due to a trademark issue it was changed to Couch. Since its initial release, CouchDB has been adopted by major companies like IBM, Oracle, Google, Microsoft and many more The latest version of CouchDB as of 2020 is version 2.3.1 and it supports multiple OS i.e. Linux, OSX, and Windows.
Features of CouchDB
CouchDB includes several features that make it unique.
• Document Storage: CouchDB stores its data in JSON documents, which makes it easier to access and manipulate data. • Replication: CouchDB supports replication, which allows users to keep multiple copies of their data in different locations. This helps keep data safe in case of disasters or outages. • Data Synchronization: CouchDB includes a feature called “data synchronization” which allows users to keep their data synchronized across multiple devices and services. • HTTP API: CouchDB includes an easy-to-use HTTP API that can be used to access, query, and manipulate documents directly from any web browser. • MapReduce Queries: CouchDB can run MapReduce queries to quickly retrieve and analyze large amounts of data.
Adoption
CouchDB is often used by developers to create web and mobile applications that require large amounts of data storage, synchronization, and replication. Here are some examples of how CouchDB can be used in real-world applications.
• Mobile Apps: CouchDB is popular among mobile developers for synchronizing data between different devices. By using CouchDB, developers can build apps that keep data synchronized across multiple devices. • Web Applications: Web developers often use CouchDB to store and access large amounts of data. The easy-to-use HTTP API allows developers to quickly query and manipulate documents directly from any web browser. • Analytics: MapReduce queries allow developers to quickly analyze large amounts of data stored in CouchDB. This is useful for applications that need to generate reports or aggregate data from multiple sources.
Advantages of CouchDB
Easy to use
CouchDB is easy to use and is a great choice for those who are just starting out with NoSQL databases. Its straightforward API allows users to quickly get up and running. Also, its built-in RESTful web interface makes it even easier to access data.
Great Scalability
CouchDB has great scalability due to its sharding capabilities. It also provides high availability which makes sure that data is always available even when some of the nodes fail.
Good Data Modeling
CouchDB provides good data modeling capabilities. It is schema-free which makes it easy to store different type of data without having to worry about specific schemas. Also, its powerful query system allows users to query data in a much efficient way.
Features of CouchDB
Security
CouchDB offers features like authentication and authorization which makes sure that user’s data is secure. Its Access Control List feature also allows users to control who can access the database and what operations they can perform on the data.
Replication
CouchDB provides replication which enables users to synchronize data between multiple remote databases. This feature is especially helpful for cloud-based applications where data needs to be replicated across multiple locations.
Flexible Data Model
CouchDB provides a very flexible data model. Documents can have different data types and fields can be added or removed as needed. This flexibility allows developers to create applications using a variety of data models.
Event Handler
CouchDB provides an Event Handler feature which allows developers to define some custom logic that will be executed when an event occurs. This feature is useful for implementing data validation, audit logs, and other application specific logic.
Adoption
Content Management System
CouchDB can be used to create content management systems. It provides great scalability and data modeling capabilities which make it a great choice for managing large amounts of data.
Mobile Applications
CouchDB is a great choice for mobile applications due to its replication capabilities. Replication allows data to be synced across multiple devices which makes it ideal for applications that need to be used on multiple devices.
IoT Applications
CouchDB can be used to create real time IoT applications. Its replication capabilities are especially helpful for this use case as it allows data to be synced across multiple devices.
Gaming Applications
CouchDB is a great choice for creating gaming applications due to its scalability, performance and data modeling capabilities. Its replication feature also makes it ideal for creating multiplayer games.
Code Examples
Connecting to a database
The following code example demonstrates how to get a database connection in CouchDB.
// Get the database client
var couch = require('nano')('http://localhost:5984');
// Connect to database
couch.db.get('dbname', function (err, body) {
if (!err) {
console.log('Database connected successfully!');
}
});
Creating a document
The following code example demonstrates how to create a document in CouchDB.
// Create a document
couch.insert({name: 'John Doe'}, 'testdoc', function (err, body) {
if (!err) {
console.log('Document created successfully!');
}
});
Updating a document
The following code example demonstrates how to update a document in CouchDB.
// Update a document
couch.get('testdoc', function (err, body) {
if (!err) {
body.name = 'Jane Doe';
couch.insert(body, 'testdoc', function (err, body) {
if (!err) {
console.log('Document updated successfully!');
}
});
}
});
Deleting a document
The following code example demonstrates how to delete a document in CouchDB.
// Delete a document
couch.destroy('testdoc', 'revision-number', function (err, body) {
if (!err) {
console.log('Document deleted successfully!');
}
});
Here are some examples of how to use CouchDB in code.
To add data to a CouchDB database, you can use the “PUT” command. For example:
curl -X PUT http://url/database/doc_id \
-H "Content-Type: application/json" \
-d '{ "name": "John Doe", "age": 20 }'
To edit data in a CouchDB database, you can use the “POST” command. For example:
curl -X POST http://url/database/doc_id \
-H "Content-Type: application/json" \
-d '{ "name": "Jane Doe", "age": 21 }'
To retrieve data from a CouchDB database, you can use the “GET” command. For example:
curl -X GET http://url/database/doc_id
To delete data from a CouchDB database, you can use the “DELETE” command. For example:
curl -X DELETE http://url/database/doc_id
Creating a Document
This example creates a new document with the specified id, fields, and values:
PUT / database / _id
{
"field1"
:
"value1",
"field2"
:
"value2"
}
Updating a Document
This example updates an existing document with the specified id and fields:
PUT / database / _id
{
"_rev"
:
"1-abcdefghijklmnopqrstuvwxyz",
"field1"
:
"new value1",
"field2"
:
"new value2"
}
Querying a Document
This example queries a document using Lucene Query Syntax:
GET / database / _all_docs ? q = field1 : value1
Deleting a Document
This example deletes a document with the specified ID:
DELETE / database / _id ? rev = 1 - abcdefghijklmnopqrstuvwxyz
Conclusion
CouchDB is an open source NoSQL database designed for scalability and resilience. It uses JSON documents to store data with an HTTP API for easy access and offers built-in replication for both one-to-one and master/slave synchronization of data. It is used by some of the world’s largest companies and open source projects and is an ideal choice for developers looking for an alternative to popular SQL databases.