Swift
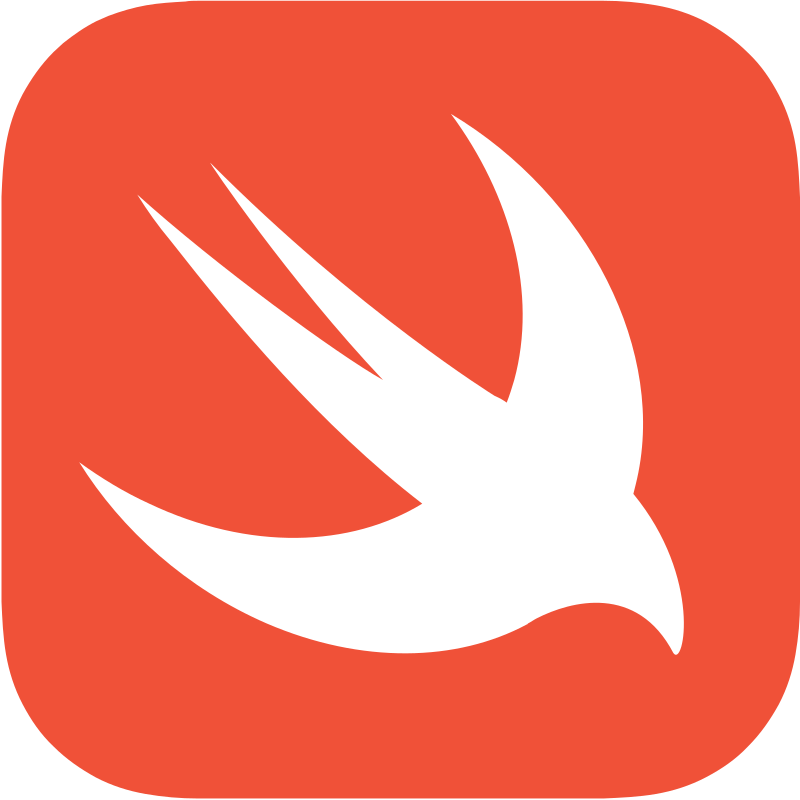
Swift
Swift is an open source programming language developed by Apple Inc. for the development of iOS, macOS, watchOS, tvOS, and Linux applications. It was first released in 2014 and is currently used for everything from web applications to server-side programming.
History
Originally created by Apple in 2009 as a replacement for Objective-C and the NeXTStep platform, Apple debuted Swift as its official programming language during WWDC 2014 and made it available for public use in 2015. Since its launch, Swift has gone on to become one of the most popular programming languages in the world, boasting over 130 million downloads in its first five years. Currently, Swift is a top 10 language based on the TIOBE Index and is the fastest growing language in history.
Origins
The origins of Swift can be traced back to 2010 when Apple started working on a new language for its development platforms. The language was initially codenamed “Project Benson” and it was envisioned as a modern and powerful language to replace Objective-C. The language was officially announced at WWDC 2014 with the goal of making programming easier and more concise than Objective-C.
Features
Swift is a multi-paradigm language that supports object-oriented, procedural, and functional programming paradigms. It is strongly typed and provides static typing which helps to improve code readability and allows for greater error-checking. It also supports dynamic libraries which makes development faster and more efficient. It also has a built-in garbage collector which automatically manages memory by freeing up unused memory resources.
Some of the features that make Swift particularly appealing include:
- Closures: Closures are functions that can be passed as parameters and preserve their context. This allows developers to write very concise code that is easier to read.
- Optionals: Optionals are a type of variable that can hold two different values, either a value or nothing. Optionals are an important feature of Swift because they allow for safe handling of data that may or may not exist.
- Pattern matching: Pattern matching is a way to switch over multiple values. It allows for more concise and powerful code.
- Generics: Generics are a way of writing code that is both type-safe and reusable. Generics allow developers to write code that can work with any type of data.
Applications
Swift is used to develop applications for Apple’s operating systems. It can be used to create both native apps as well as cross-platform apps that can be deployed to multiple platforms. Many popular apps have been built with Swift, including AirBnB, Uber, Instagram, and Twitter. It has also been used for game development. Popular games such as Firewatch and Monument Valley were built with Swift.
Advantages
Swift is a powerful and versatile language that offers many benefits to developers. Some of its advantages include:
- It’s fast: Swift is designed to be fast and efficient. This makes it a great choice for applications that need to perform tasks quickly.
- Safe and secure: Swift is type-safe and provides static typing which makes it easier to read, debug, and maintain.
- Easy to learn: The syntax of Swift is easy to understand and it is designed to be intuitive and approachable.
- Open source: Swift is open source and freely available to anyone.
Usages
Swift can be used for a variety of development tasks, from web programming to mobile app development to enterprise software development. It is the primary language used for developing iOS and macOS apps and is widely used for WatchOS and tvOS app development as well.
Swift is also a great choice for building server-side applications, as it can be used to create server APIs, manage databases, and host websites. It is becoming increasingly popular for game development as well, with Unity and Unreal Engine both supporting Swift as a development language.
Code Examples
Here are some examples of simple Swift code.
let foo = "Hello, world!"
print(foo)
This simple code will print out the string Hello, world!
in the console.
func addTwoNumbers (a: Int, b: Int) -> Int {
return a + b
}
let result = addTwoNumbers(a: 4, b: 6)
print(result)
This function adds two numbers and prints the result in the console. It will print out 10
.
struct Point {
var x: Int
var y: Int
}
let point = Point(x: 1, y: 2)
print(point)
This code defines a Point
struct and creates an instance of it. It will print out Point(x: 1, y: 2)
.
Here is an example of Swift code that prints out “Hello, World!”
print("Hello, World!")
This code creates a string object with the text “Hello, World!” and then prints it out to the console.
Creating Strings
Strings are a common data structure used to store textual data. It can be created with either the mutable or immutable types. Here is an example of a string created with the immutable type:
let textString = "This is a string"
Accessing and Modifying Strings
To access a particular character in a string, use the subscript notation. To modify a string, use the append() method, as shown below:
var myName = "John Smith"
let thirdCharacter = myName[2] //Will return "h"
myName.append(" Sr") //Will modify the string to "John Smith Sr"
Using Arrays
Arrays are used to store collections of values. Here is an example to create an array of strings:
let myFavorites = ["Apple", "Banana", "Strawberry"]
Accessing and Modifying Arrays
To access a particular element in an array, use the subscript notation. To modify an array, use the append() method, as shown below:
var myFruits = ["Apple", "Banana", "Strawberry"]
let secondFruit = myFruits[1] //Will return "Banana"
myFruits.append("Orange") //Will modify the array to ["Apple", "Banana", "Strawberry", "Orange"]
Working with Dictionaries
Dictionaries are used to store key-value pairs. Here is an example to create a dictionary of strings:
let myFamily = ["Father": "John", "Mother": "Jane", "Brother": "Jim"]
Accessing and Modifying Dictionaries
To access a particular value in a dictionary, use the subscript notation. To add a new key-value pair, use the updateValue(_:forKey:) method, as shown below:
var myFriends = ["Friend 1": "Jake", "Friend 2": "Jack"]
let friend1 = myFriends["Friend 1"] //Will return "Jake"
myFriends.updateValue("Jill", forKey: "Friend 3") //Will add a new key-value pair, modifying the dictionary to ["Friend 1": "Jake", "Friend 2": "Jack", "Friend 3": "Jill"]
Performing Loops
Loops are used to execute a set of statements multiple times. Here is an example of a loop while loop in Swift:
var i = 0
while i < 10 {
print(i)
i += 1
}
The code above will print out the numbers 0 through 9.
Working with Classes and Objects
Classes and objects are used to create and manage complex data structures. Here is an example to create a class and an object in Swift:
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
}
let jane = Student(name: "Jane Doe", age: 18)
The code above will create a class called Student, which has two properties: name and age. It also creates an object called jane of type Student, with the specified name and age.
Here is an example of a simple Swift program that prints “Hello World” to the console:
// Program to print "Hello World"
import Foundation
print("Hello World!")
This code will compile and run, printing “Hello World!” to the console.
Conclusion
Swift is a powerful programming language used to develop iOS, macOS, watchOS and tvOS applications. It is a modern language that is designed to be easier to work with and more efficient than other languages. As such, it has become increasingly popular among developers seeking to create powerful applications. Swift offers numerous libraries and frameworks that can be used to create powerful applications with fewer lines of code. Additionally, there are many third-party libraries that can be used together with Swift, making it an even more powerful language. With the help of Swift, developers can create robust and efficient applications for Apple products in less time than ever before.