Perl
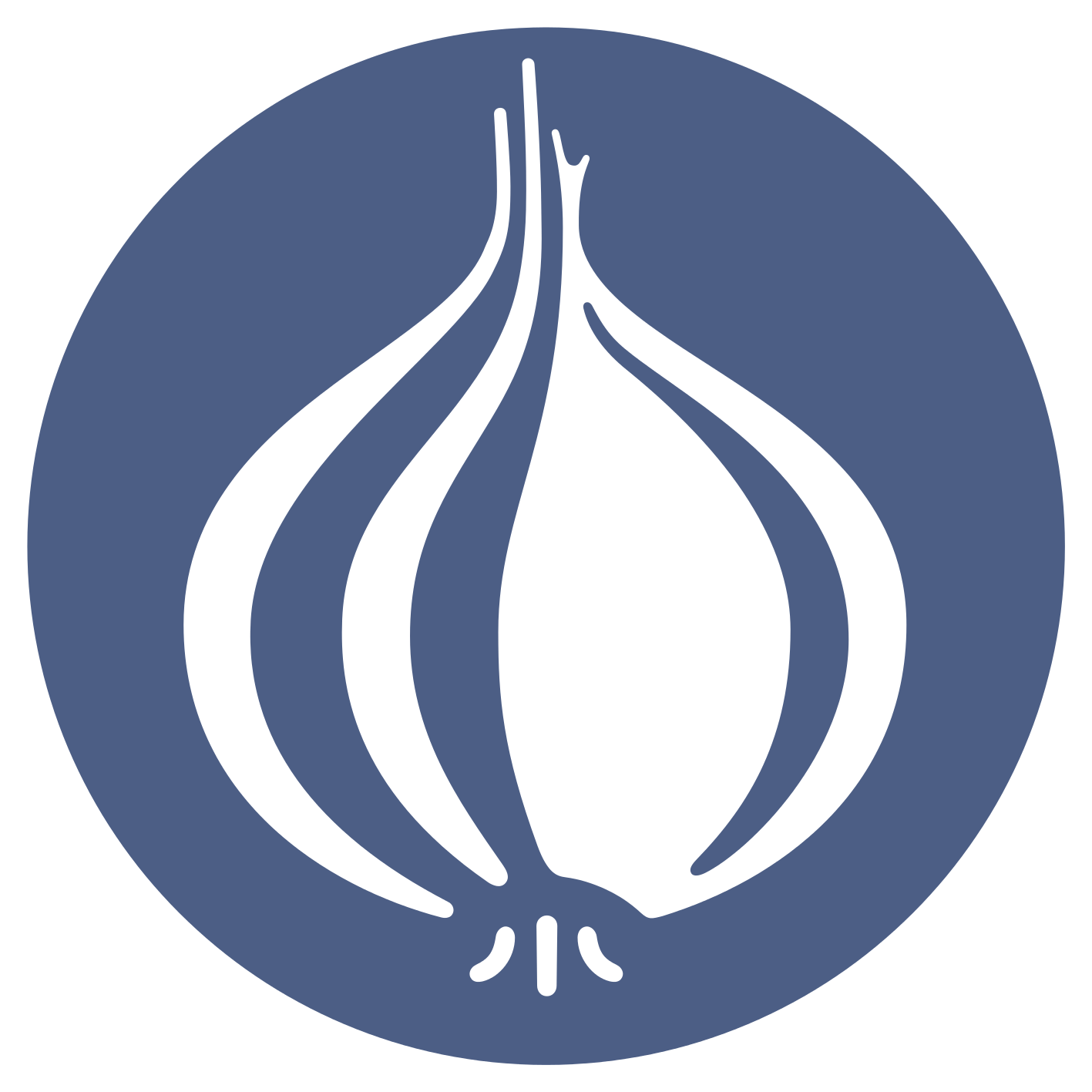
Introduction to Perl
Perl is a powerful and popular programming language that is widely used for web development, system administration, network programming, and other applications. It is an interpreted language, meaning that it does not need to be compiled before being run. Perl comes with a large library of built-in features and functions which make writing programs in Perl easier and faster.
History of Perl
Perl was first released in 1987 by Larry Wall, who had previously worked on the UNIX operating system. Wall then continued to develop and refine the language throughout the 1990s, releasing version 5 in 1994. Version 5 was a major overhaul of the language, introducing many features that are still used today. In 2000, the sixth version of Perl was released and since then each version has included more new features and capabilities. Developed by Larry Wall in 1987, Perl was initially released under the name “Practical Extraction and Report Language” ( or PERL). The language was designed to be a versatile tool that could be used to efficiently manipulate text, automate programs and quickly implement tasks. In 1993, John Ousterhout, creator of the Tcl scripting language, modified Perl to create a more user-friendly version, known as the “Perl 5” release. Perl 5 went through several iterations including “Perl 5.10″ (released 2007), “Perl 5.12″ (released 2010) and “Perl 5.20″ (released 2014). These releases improved upon the language’s features, including optimizations to enhance speed and performance. In 2015, Perl 5.22 was released which added new features such as a Unicode support system, references, memory management and support for 64-bit platforms. Today, Perl continues to be a popular choice for text manipulation, automation and system administration tasks, primarily due to its flexibility, efficiency and cross-platform compatibility.
Examples of Usages
Perl can be used to develop complex applications, including web services, web applications, desktop applications and more. It’s also commonly used for system administration and manipulations tasks, as well as text processing.
Below are some examples of Perl uses:
- Web Automation – Many websites use Perl to automate various tasks such as updating content, retrieving information from databases and sending emails.
- Text Processing – Perl is often used to read, parse and filter data files, and manipulate large amounts of text quickly and efficiently.
- Network Applications – Perl offers an array of networking features, making it an excellent choice for developing network applications.
- System Administration – Perl makes it easy to automate system administration tasks such as account creation and deleting, file transfers, emailing, backups, etc.
- Web Development – Perl can be used to create dynamic websites using CGI, mod_perl, or other Perl-based frameworks such as Catalyst and Mojolicious.
Features of Perl
Perl is known for its wide range of features, allowing developers to quickly create powerful and flexible programs. Some of the most commonly used features include:
- A rich set of built-in data types, including scalars, arrays, hashes, and objects
- A large selection of powerful operators and control structures
- Easy access to system resources, such as files and sockets
- Built-in support for regular expressions
- An extensive library of modules available from the CPAN
- Comprehensive support for object-oriented programming
- Integrated debugging tools
- Comprehensive documentation
How to Use Perl
Perl code is written in plain text files and saved with the suffix “.pl”. To run a Perl program, you can use the perl interpreter, usually accessed on the command line by typing “perl” or “perl my_program.pl”. Perl programs can also be embedded in HTML files and executed by a web server.
Examples of Perl Code
We will now take a look at a few examples of Perl code. These are taken from the Perl Tutorial on the official Perl website:
Hello World Program:
#!/usr/bin/perl
print "Hello, world!\n";
The first line identifies the location of the Perl interpreter. The second line prints “Hello, world!” on the screen.
Looping over an Array:
my @array = (1, 2, 3);
foreach my $item (@array) {
print $item . "\n";
}
This code creates an array with three elements and then loops over each element in the array, printing them out one by one.
Checking if a Variable is Defined:
if (defined $var) {
print "Variable is defined.\n";
} else {
print "Variable is undefined.\n";
}
This code checks whether a variable is defined and prints out a message accordingly.
Working with Regular Expressions:
if ($string =~ /(foo.*bar)/) {
print "Matched: $1\n";
}
This code uses a regular expression to check whether a string matches a certain pattern and prints out the part of the string that matched.
Hello World
Here’s a basic Perl program to print ‘Hello World’ to the console:
#!/usr/bin/perl
print "Hello, World!\n";
File I/O
The following code demonstrates how to read and write data to a file in Perl:
#!/usr/bin/perl
# Open a file handle
open my $fh, '<', 'myfile.txt' or die "Can't open file: $!";
# Read the file line by line
while (my $line = <$fh>) {
chomp $line;
# Do something with $line here
}
close $fh;
# Open a file handle
open my $fh, '>', 'myfile.txt' or die "Can't open file: $!";
# Write data to the file
print $fh "Hello, World!\n";
close $fh;
Regex
The following code demonstrates how to match a regular expression in Perl (this example matches a US telephone number):
#!/usr/bin/perl
use strict;
use warnings;
if ( $text =~ m/^(\d{3})-(\d{3})-(\d{4})$/ ) {
print "Matched \n";
}
else {
print "No match. \n";
}
The following code example shows how Perl can be used to create a dynamic web page using Apache and CGI scripts:
#!/usr/bin/perl
use CGI qw(:standard);
print "Content-type: text/html
<html>
<head><title>My first Perl program</title></head>
<body>
<h1>Hello, world!</h1>
</body>
</html>
System Administration
The following code example shows how Perl can be used to automate the management of a network using Net::Telnet:
#!/usr/bin/perl
use Net::Telnet;
my $telnet = Net::Telnet->new( Host => '10.0.0.1' );
$telnet->login('username','password');
$telnet->cmd('configure terminal');
$telnet->cmd('interface eth 0/0');
$telnet->cmd('ip address 192.168.1.1 255.255.255.0');
$telnet->cmd('exit');
$telnet->close;
Database Management
The following code example shows how Perl can be used to query and manipulate a database using DBI and DBD::mysql:
#!/usr/bin/perl
use DBI;
# Connect to the database
my $dbh = DBI->connect('dbi:mysql:dbname', 'username', 'password')
or die "Unable to connect: $DBI::errstr\n";
# Perform a simple query
my $stmt = "SELECT * FROM table_name";
my $sth = $dbh->prepare($stmt);
$sth->execute();
while (my $row = $sth->fetchrow_hashref()) {
print "Name: $row->{name}\n";
}
$dbh->disconnect;
Text Processing
The following code example shows how Perl can be used to parse and manipulate text using Text::CSV:
#!/usr/bin/perl
use Text::CSV;
my $csv = Text::CSV->new({ binary => 1 });
open( my $fh, "<", "file.csv" ) or die "Can't open file.csv: $!";
while (my $row = $csv->getline( $fh )) {
print "Name: $row->[0]\n";
}
close $fh;
The following is an example of a simple Perl program that prints out “Hello, World!”
#!/usr/bin/perl
print "Hello, World!\n";
The following is an example of a Perl script that reads a text file line by line, reverses each line, and then prints out the reversed lines:
#!/usr/bin/perl
open(FILE, "<sample.txt");
while ($line = <FILE>) {
chomp($line);
$rev_line = reverse($line);
print "$rev_line\n";
}
close(FILE);
Conclusion
In conclusion, Perl is a powerful and popular programming language that is used for many different tasks. It comes with many useful features, including built-in data types, operators, and libraries, making it easy to create powerful programs quickly and efficiently. We have examined some examples of Perl code, showing how easy it is to use the language to accomplish common programming tasks.