Elasticsearch
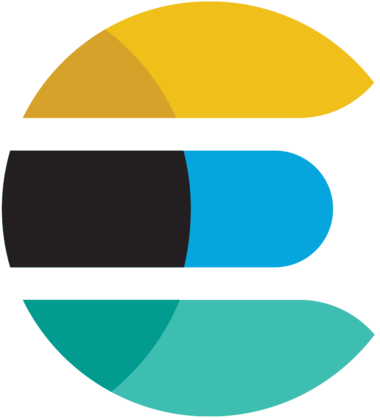
Introduction
Elasticsearch is an open source, distributed, NoSQL search engine and analytics platform. It provides a powerful search engine that enables users to quickly find the most relevant documents from their collections. Elasticsearch is based on Apache Lucene, a well-known search library written in Java. Elasticsearch was created in 2010 by Shay Banon and has since become one of the most popular open source projects in the world. It is used by companies like Netflix, eBay, and Stack Overflow for data analysis and retrieval.
History
The original developers of Elasticsearch, Shay Banon and Lee Hinman, began working on the project in 2003 as an extension of their open-source search engine, Compass. The two were initially working on a standard Apache Lucene-based search engine, but eventually decided to build a new system from scratch. The first version of Elasticsearch was released in February 2010, and it quickly gained popularity among developers due to its simple API and high performance. Since then, the project has grown rapidly, with a thriving open-source community and a “plugable” architecture that allows users to customize the system to their own needs. In 2015, Elasticsearch joined forces with Logstash and Kibana to form the Elastic Stack, a comprehensive suite of open-source data tools. This created an end-to-end search and analytics platform that made it easier than ever before to gain insights from data. Today, Elasticsearch is one of the most popular open-source search and analytics engines in the world. It is used by organizations of all sizes, from startups to Fortune 100 companies, to store and search data.
Features of Elasticsearch
Elasticsearch provides a range of features that enable developers to quickly and easily build search experiences. These include:
- Automatic Clustering: Elasticsearch provides automatic clustering capabilities, which allow the search engine to scale horizontally and dynamically adjust to changes in data and usage.
- Full-Text Search: Elasticsearch uses Lucene-based full-text search capabilities, allowing users to search for words or phrases within documents.
- Aggregations: Aggregations are used to group and summarize data for further analysis.
- Geo Search: Elasticsearch provides geo search capabilities, which allow users to search for locations and distances from an origin point.
- Analyzers: Analyzers are used to transform data before it is indexed, and can be used to identify synonyms, stop words, and more.
- Search Relevance: Elasticsearch provides relevance tuning capabilities, which allow users to adjust how relevant search results should be.
Benefits of Elasticsearch
Elasticsearch provides a number of benefits to developers, including:
- Scalability: Elasticsearch is highly scalable and can be used to support applications with large volumes of data.
- Performance: Its distributed architecture enables it to handle high query rates and quickly return search results.
- Security: Elasticsearch provides tools to protect sensitive data, such as the ability to encrypt traffic and data at rest.
- Flexibility: The search engine allows users to customize their search experience with query parameters, filter options, and other capabilities.
- Open-source: Elasticsearch is an open-source project, which means developers can access the source code and modify it to meet their needs.
Adoption
Log Analysis
Elasticsearch is an ideal solution for log analysis. It provides efficient querying and analysis of large amounts of machine data, such as website visitor logs, system performance logs, and networking device data. By identifying patterns in the data, Elasticsearch can help businesses understand user behavior trends and improve their products.
Text Analysis
Elasticsearch is also a great tool for text analysis. The search engine’s built-in stemming capabilities allow developers to quickly analyze and extract meaning from natural language text, enabling applications such as natural language processing or sentiment analysis.
Business Intelligence
Elasticsearch can be used for business intelligence purposes. Business intelligence involves gathering data from multiple sources, analyzing it, and then taking action that results in positive outcomes for the organization. Elasticsearch helps businesses transform their data into meaningful and actionable insights, allowing them to make smarter decisions.
Code Examples
Below are some example code snippets to get you started with Elasticsearch.
Installation
Install Elasticsearch using the package installer on the official website.
$ wget https://artifacts.elastic.co/downloads/elasticsearch/elasticsearch-7.10.2-linux-x86_64.tar.gz
$ tar -xvf elasticsearch-7.10.2-linux-x86_64.tar.gz
$ cd elasticsearch-7.10.2/bin/
$ ./elasticsearch
Indexing
To index a document, first we need to create an index in Elasticsearch:
PUT /myindex
Then we can index a document:
PUT /myindex/_doc/1
{
"title": "My Document",
"content": "This is my document!"
}
Search
To search an index, we use the _search
API:
POST /myindex/_search
{
"query": {
"match": {
"content": "document"
}
}
}
Below is an example of how to index a document in Elasticsearch using the Java API:
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.client.RestHighLevelClient;
public void indexDocument(RestHighLevelClient client) throws IOException {
IndexRequest request = new IndexRequest("index-name")
.id("document-id")
.source("field1", "value1",
"field2", "value2",
...);
client.index(request);
}
Below is an example of how to execute a search query in Elasticsearch using the Java API:
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.client.RestHighLevelClient;
public void search(RestHighLevelClient client) throws IOException {
SearchRequest request = new SearchRequest("index-name");
request.source("query": {
"match": {
"field1": "value1"
}
});
SearchResponse response = client.search(request);
// process response
}
Below is an example of how to use Elasticsearch to perform a simple full-text search:
GET /products/_search
{
"query": {
"multi_match" : {
"query": "search term",
"fields": ["name", "description"]
}
}
}
This query searches the products
index for documents with a name or description containing the search term.
Elasticsearch also supports advanced search capabilities such as relevance scoring, fuzzy matching, and structured queries. For more examples, see the Elasticsearch documentation.
The following is an example of how to use the Elasticsearch Java API to index a document:
import org.elasticsearch.action.index.IndexResponse;
import org.elasticsearch.client.Client;
import org.elasticsearch.client.transport.TransportClient;
import org.elasticsearch.common.settings.Settings;
import org.elasticsearch.common.transport.InetSocketTransportAddress;
public class IndexingExample {
public static void main(String[] args) throws Exception {
// Setup the client
Settings settings = Settings.settingsBuilder()
.put("cluster.name", "elasticsearch").build();
Client client = TransportClient.builder().settings(settings).build()
.addTransportAddress(new InetSocketTransportAddress(
InetAddress.getByName("localhost"), 9300));
// Index a document
Map<String, Object> data = new HashMap<>();
data.put("title", "Elasticsearch Tutorial");
data.put("content", "This is test content...");
IndexResponse response = client.prepareIndex("wiki", "doc")
.setSource(data).get();
// Cleanup
client.close();
}
}
Conclusion
Elasticsearch is one of the most popular open source search engine projects available today, and it is used in a variety of different contexts. It is easy to use because of its Java API, and the Elastic Stack offers features such as Logstash, Kibana, Beats, X-Pack and Graph Extension. With its powerful capabilities, it is no wonder why Elasticsearch is becoming ever more popular.