Rust
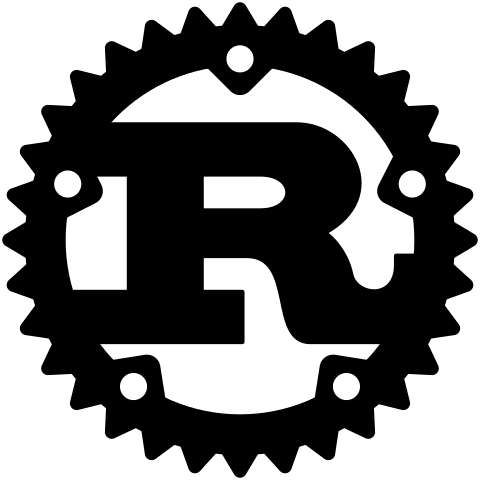
Rust
Rust is a systems programming language that focuses on performance, reliability, and security. It is designed to be fast, memory-efficient, and provide memory safety guarantees without using garbage collection or other runtime components. It is also designed to work well with existing C and C++ libraries and codebases.
History
The development of Rust began in 2006 at a small research project at Mozilla Research and has been sponsored by the Mozilla Corporation since 2009. In 2010, Rust was announced to the public and has since grown in popularity and use.
The language saw a major release every 6 months from version 0.6 in 2012 to version 1.0 in 2015. Since then, new features and syntax changes have been added gradually with version updates every 6 weeks.
Use Cases
Rust is used for a variety of applications ranging from embedded devices, web services, game development, operating systems, and more. Some notable users of Rust include Dropbox, Amazon Web Services, npm, Canonical (Ubuntu), Google, Microsoft, and Docker.
Some popular projects built with Rust include Firefox, Servo, Redox OS, QuestDB, and WebRender.
Syntax
Rust uses a mix of imperative and functional programming paradigms. The language is statically typed and supports type inference. It uses early error checking to ensure that programs are free of crashing bugs and memory safety issues.
Rust supports features such as generics, traits, macros, pattern matching, type aliasing, enums, and more. The language also has an extensive standard library for common tasks such as data structures, I/O, and concurrency.
Below is a Hello World program written in Rust:
fn main() {
println!("Hello, world!");
}
This program is a basic “Hello, World!” program. It uses the println!
macro to print the string “Hello, World!” to the
console.
Features of Rust
Rust is a statically-typed language, meaning that type checking is done at compile time rather than at run-time. This allows for greater code efficiency and accuracy. Rust also features powerful abstractions that allow developers to avoid dealing with the underlying system details and work with higher level abstractions and concepts instead. Other features of Rust include:
- Memory Safety - Rust guarantees memory safety without relying on garbage collection.
- Concurrency - Rust provides support for multi-threaded programming, allowing developers to write highly concurrent applications.
- Performance - Rust provides excellent performance, often beating out C/C++ code in terms of speed, which makes it suitable for situations where performance is of utmost importance.
- Toolchain - Rust comes with a comprehensive set of tools to help developers build robust and reliable applications.
Memory Safety
Rust uses a combination of static typing and ownership rules to ensure memory safety. The idea behind this is that Rust’s ownership system prevents memory issues such as data races, use after free, and memory leaks.
Every variable in Rust is tracked for its lifetime. This means that once a variable has gone out of scope, its memory is automatically freed. This ensures that the user doesn’t have to manually manage the memory of their programs.
Concurrency
Rust supports concurrent programming through its built-in support for asynchronous programming. Asynchronous functions allow for different parts of a program to run concurrently and communicate through channels.
Rust also includes support for parallelism, which allows multiple functions to execute on multiple threads at the same time. This allows for faster execution of tasks.
Community
Rust has an established and active community. The official website hosts discussion forums and provides tutorials and examples to help new users get started.
There are also many online communities such as Reddit and Stack Overflow where users can ask questions and share code snippets. These forums are a great way to find answers to any questions you may have about Rust.
The official Rust book, “The Rust Programming Language” is available online and provides a detailed overview of the language, its features, and examples of how to use it.
Libraries
There is a large and growing ecosystem of Rust libraries that support various tasks and operations. These libraries are maintained by the Rust community, and most are available on crates.io.
Some of the most popular Rust libraries include Tokio (async runtime), Diesel (database access), Actix (web server), Serde (serialization/deserialization), Hyper (HTTP client/server), and Rocket (web framework).
Conclusion
Rust is a modern systems programming language that emphasizes safety, reliability, and performance. With its focus on memory safety, Rust ensures that programs are free of crashing bugs and memory leaks. It also supports a variety of programming paradigms, provides powerful abstraction capabilities, and has an extensive Standard Library.
Rust is used in a variety of applications, ranging from back-end web services to embedded devices and game development. It also has an active community that supports and maintains open source libraries and tools.
If you’re looking for a modern language to power your applications, Rust is worth considering.
Adoption
Rust is regularly used to build web servers, games, operating systems, and even compilers. It has been used to create mission-critical applications ranging from security-critical software such as PasswordSafe to graphics-intensive applications such as the Unity game engine.
Rust is also popular for IoT devices due to its small memory footprint and fast execution speed. It is well-suited for embedded applications such as robotics and drones due to its ability to manage resources safely and efficiently.
In addition, Rust provides great concurrency support, making it ideal for distributed systems and cloud computing. This has spurred the development of a number of frameworks that make it easier to develop distributed applications.
Examples of Rust Code
The following code is written in Rust and illustrates a basic Hello World program:
fn main() {
println!("Hello, world!");
}
The following example demonstrates a basic example of Rust’s ownership system:
fn main() {
let mut x = 5;
let y = &mut x;
*y += 1;
println!("x: {}", x);
}
The following example shows Rust’s powerful pattern matching feature:
fn main() {
let mut x = 1;
match x {
0 => println!("x is zero"),
1 | 2 => println!("x is one or two"),
3..=10 => println!("x is in range 3..=10"),
_ => println!("x is something else"),
}
}
The following example demonstrates Rust’s asynchronous programming capabilities:
use std::future::Future;
use std::time::Duration;
async fn task() -> u32 {
// Do some CPU intensive work
42
}
async fn long_running_task() -> i32 {
// Sleep for 3 seconds
tokio::time::delay_for(Duration::from_secs(3)).await;
// Return a result
1
}
fn main() {
let future_1 = task();
let future_2 = long_running_task();
// Use `join` to wait for both futures to complete
let (result_1, result_2) = futures::join!(future_1, future_2);
println!("Result of first future: {}", result_1);
println!("Result of second future: {}", result_2);
}
Here is a simple “Hello World” example written in Rust:
fn main() {
println!("Hello World!");
}
Here is an example of a simple program that adds two numbers:
fn main() {
let x = 10;
let y = 20;
let sum = x + y;
println!("The sum of {} and {} is {}", x, y, sum);
}
Here is an example of a program that implements concurrency using Rust’s threading feature:
use std::thread;
fn main() {
let handle = thread::spawn(move || {
println!("This is the child thread");
});
println!("This is the main thread");
handle.join().unwrap();
}
Let’s take a look at a simple example of Rust code. This program prints “Hello, World!” to the console:
fn main() {
println!("Hello, World!");
}
When compiled and run, this program prints “Hello, World!” to the console. As you can see, Rust code is easy to read and understand.
Conclusion
Rust is a powerful, open-source systems programming language that offers memory safety, scalability, and performance benefits. It is being increasingly adopted for a variety of tasks, from embedded systems programming to resource-intensive server backends. With its growing popularity and community, Rust looks set to continue its rise in the coming years.