F#
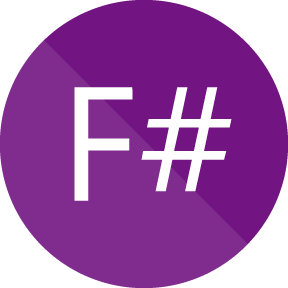
Introduction to F#
F# is a multi-paradigm programming language that encompasses functional, imperative, and object-oriented programming. Originally developed by Microsoft for use in the .NET framework, it is now open source and available on several other platforms. It has a strong focus on productivity and can offer many advantages over other languages, such as a cleaner syntax, higher performance, and lower development time.
History
F# is based on the ML programming language, which was developed at the University of Edinburgh in 1973. ML was a popular academic language throughout the 1980s but eventually lost traction. By the mid-1990s, Don Syme had begun to develop a version of ML for the .NET environment. In 2005 Microsoft released F# as a research project. It gained popularity among software developers, who found its concise syntax and functional-first approach to be very expressive and powerful. The initial releases were closely integrated with the .NET development environment and Microsoft Visual Studio. In 2012, F# 3.0 was released, featuring improved support for asynchronous programming and support for HTML5. This made F# well-suited for web development, which has become one of its major applications. In 2014, Version 4.0 was released, adding additional syntax improvements and language features. This release also added support for Universal Windows Platform apps and .NET Core. In 2018, F# 4.5 was released with further updates to the language and library. This version includes improved support for functional programming, improved type inference, and performance enhancements.
Language Features
F# is a statically typed language which means that all values must have their types explicitly declared in order for the compiler to be able to check them for correctness. This results in programs that are faster and more reliable since the compiler can detect and correct any incorrect usage at compile time.
The language also supports a range of declarative programming paradigms, including:
- Functional Programming: functional programming emphasizes the use of functions to manipulate and transform data. F# features high-level features such as pattern matching and lambda expressions to make this style of programming easier.
- Object-Oriented Programming: F# lets you create classes and objects, making it suitable for building object-oriented applications. Its type system is designed to facilitate composition, allowing you to mix and match different styles of programming.
- Imperative Programming: F# also supports imperative programming, which requires the programmer to explicitly state how each operation should be carried out. This style of programming is useful for implementing algorithms and for creating low-level system software.
Furthermore, F# also provides strong support for distributed computing and parallel programming, making it suitable for a wide range of use cases.
Benefits and Drawbacks
F# offers a number of benefits over other languages, including a robust type system and a functional programming style. Its type system allows for safe and reliable code, while its functional style helps developers produce maintainable and extensible code. F# is also a great tool for rapid prototyping and scripting, due to its concise syntax and interactive command line REPL.
However, there are some drawbacks to using F#, such as its relatively steep learning curve and lack of well-documented tutorials. Additionally, F# might not be suitable for certain problems, such as embedded systems or low-level optimization, due to its high-level abstractions.
Adoption
F# is particularly suited for a wide range of problem domains, including big data analysis, machine learning, artificial intelligence, web development, financial computing, and scientific computing. Here are some examples of how F# can be used:
- Big Data Analysis: F# provides rich support for parallelism and asynchronous programming, allowing developers to quickly and easily process large amounts of data.
- Machine Learning: F# makes it easy to create applications for analyzing and predicting data, such as machine learning models for natural language processing and computer vision.
- Artificial Intelligence: F# can be used to develop applications featuring complex decision-making and problem-solving capabilities.
- Web Development: F# can be used to create web applications using its built-in web development framework, called Suave.
- Financial Computing: F# can be used to create sophisticated financial applications, such as algorithmic trading systems.
- Scientific Computing: F# supports numerical computation and can be used to create scientific applications, such as physical simulations.
Examples
To give an idea of how F# code looks like, here’s a simple example of a function that adds two integers:
let add x y =
x + y
To call the function we simply pass in the two numbers as arguments:
let result = add 5 10
This will assign the value 15
to the variable result
.
Here’s another example that demonstrates the functional programming style of F#. This example uses a pipeline of operations to filter out negative numbers from a list of integers:
let positiveInts =
[ 1; -3; 2; 0; -4 ]
|> List.filter (fun x -> x > 0)
The result of the operation is assigned to the positiveInts
variable, which will contain the list [ 1; 2; 0 ]
.
F# also supports object-oriented programming, and here’s an example of a simple class definition:
type Person =
{ Name: string; Age: int }
This code defines a Person
type, with two members: Name
, which is a string, and Age
, which is an integer. We can
use this type to create instances of Person
:
let person = { Name = "John"; Age = 30 }
Finally, we can demonstrate the imperative programming style of F# with this example of a simple loop:
for i in 1..10 do
printfn "i = %d" i
This example prints the numbers from 1 to 10.
Here’s a simple F# program that prints out “Hello World!”
printfn "Hello World!"
This program uses the printfn
function to print out a string to the console. This example shows how concise F# code
can be.
Here’s another example, which uses F#’s type inference to calculate the Fibonacci sequence:
let fib n =
let mutable a = 0
let mutable b = 1
let mutable i = 0
while i < n do
let temp = a
a <- b
b <- temp + b
i <- i + 1
a
let result = fib 10
printfn "Fibonacci of 10 is %d" result
This program takes an integer argument, n
, and calculates the n
th Fibonacci number. This example demonstrates F#’s
ability to express complex calculations with very minimal code.
Function
// Simple function
let add x y = x + y
List
// Create a simple list
let numbers = [1; 2; 3; 4]
// Map each element in the list
let numbersSquared = List.map (fun num -> num * num) numbers
// Output the result
printfn "%A" numbersSquared
// Output: [1; 4; 9; 16]
Pattern Matching
// Function to calculate area of shapes
let calculateArea shape =
match shape with
| Circle(radius) -> Math.PI * radius * radius
| Rectangle(width, height) -> width * height
// Examples
calculateArea (Circle 3.0) // 28.274333882308138
calculateArea (Rectangle 4.0 5.0) // 20.0
Discriminated Union
// Define a discriminated union
type Employee =
| Manager of string
| Developer of string
// Construct a Developer
let dev = Developer "John Smith"
// Test the value
match dev with
| Manager name -> printfn "Manager: %s" name
| Developer name -> printfn "Developer: %s" name
// Output: Developer: John Smith
Asynchronous Programming
// Use async workflows to query a remote service
async {
let! resp = async {
let client = httpClient()
return! client.GetAsync("http://example.com/api")
}
match resp with
| Ok result -> printfn "Result: %s" result
| Error err -> printfn "Error: %s" err
}
Hello World Example
This example prints “Hello, World!” to the console:
printfn "Hello, World!"
Fibonacci Sequence Example
This example prints the first 10 numbers in the Fibonacci sequence:
let rec fib n =
match n with
| 0 -> 0
| 1 -> 1
| _ -> fib(n -1) + fib(n -2)
[for i in 0..10 do
yield fib i]
|> printfn "%A"
Working with Strings Example
This example shows how to work with strings:
// Declare a string
let myString = "Hello, World!"
// Find the length of the string
let strLength = String.length myString
// Print the result
printfn "The length of the string is %d" strLength
Conclusion
F# is a powerful and expressive language that can be used for a wide variety of programming tasks. From web development to mobile development to game development to machine learning, F# is an excellent choice for developers looking for an easy-to-use language for their projects. Its concise syntax and strong type system make it a great choice for writing idiomatic and maintainable code.