Elixir
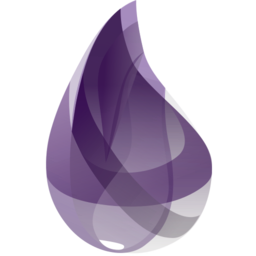
Elixir Library
Elixir is a dynamic, functional programming language that runs on the Erlang virtual machine. The language was developed by José Valim in the early 2010s to provide an extensible and powerful language for managing large data sets, writing distributed applications, and developing concurrent applications. Elixir has since been used in a variety of applications, including Web development, Internet of Things (IoT) applications, and big data processing.
History of Elixir
Elixir’s development began in 2011 when José Valim, an Erlang developer, started to work on an extensible language that ran on the Erlang Virtual Machine (VM). In 2012, the initial version of Elixir was released. Since then, the language has grown in popularity, garnering the attention of major tech companies such as Amazon, Microsoft, and Oracle. Elixir has seen several versions over the years and has been used for a variety of tasks. In 2013, Elixir 1.0 was released. This version included significant improvements in performance, scalability, and error handling. It also included new language features such as pattern-matching and improved string manipulation. In 2016, Elixir 1.3 was released, introducing more efficient code compilation and better garbage collection. Elixir 1.4 was released in 2017, which introduced a new static type system. In 2018, Elixir 1.5 was released, which added support for the Erlang Operating System (EOS). Elixir 1.6 was released in 2019, adding support for metadata and protocols.
Features of Elixir
Elixir is a general-purpose programming language that has many features that make it ideal for developing distributed systems and web applications. Some of the main features of Elixir include:
- Functional programming - Elixir is designed to be a functional programming language, meaning that programs are written using functions rather than objects. This makes Elixir well-suited for large projects and complex calculations.
- Concurrency support - Elixir has support for low-level concurrency primitives such as processes and tasks. This makes it easy to create concurrent applications that can scale easily.
- Fault tolerance - Elixir has support for error-handling and fault-tolerance, making it well-suited for applications with high availability requirements.
- Metaprogramming - Elixir has support for meta-programming, enabling developers to write code that can be extended and modified at run-time. This makes Elixir a great choice for applications that require dynamic behavior.
- Elixir is extensible - Elixir is extensible, allowing developers to create custom data types, data structures, and libraries.
Usage Examples
Elixir has been used in a variety of applications, from web development to IoT applications and big data processing.
Web Development
Elixir has a robust set of libraries that make it well-suited for web development. For example, Phoenix is a web development framework written in Elixir that allows developers to quickly create web applications. Elixir can also be used to create REST APIs, which are commonly used for web applications.
IoT Applications
Elixir has been used to create distributed applications for devices connected to the Internet of Things (IoT). Elixir’s concurrency features make it ideal for creating applications that require high throughput, while its fault-tolerance makes it suitable for applications with high availability requirements.
Big Data Processing
Elixir has been used to develop distributed applications for big data processing. Elixir’s concurrency capabilities make it well-suited for analyzing large amounts of data in parallel. Additionally, Elixir has support for an Erlang compatible distributed database called mnesia, which can be used to store and manage large datasets.
Code Examples
Elixir is a powerful language that can be used to create powerful applications. Here are some examples of basic Elixir code:
# A basic function definition
def greet(name) do
IO.puts “Hello, #{name}!”
end
# Pattern matching example
def sum(a, b) do
a + b
end
sum 1, 2 # 3
sum 1..4 # 10
# Piping expression example
1..4
|> Enum.map(&(&1 * &1))
|> Enum.sum # 30
Here are some simple examples of Elixir code.
A function to add two numbers:
def add(a, b) do
a + b
end
A recursive function to calculate the Fibonacci sequence:
def fibonacci(n) when n < 3 do
1
end
def fibonacci(n) do
fibonacci(n - 1) + fibonacci(n - 2)
end
An example of a task that runs periodically:
defmodule MyModule do
use GenServer
# ...
@impl true
def init(_init_arg) do
Task.start_link(fn -> check_for_updates end)
{:ok, %{}}
end
defp check_for_updates do
# Check for new updates and take action if necessary
end
end
Conclusion
Why Use Elixir?
Elixir has many benefits for developers. It’s easy to learn, due to the syntax being based on the Ruby programming language. This makes transitioning from Ruby much easier. It is also very fast and efficient, since it runs on the Erlang virtual machine, making it suitable for real-time applications. Finally, Elixir offers great scalability and fault tolerance.
History
Elixir is actually a relatively new programming language, only introduced in 2012 by José Valim. It was created as a tool to make more productive use of Elixir and the Erlang platform. Since then, it has gained popularity among developers due to its elegant syntax and powerful features.
Features
Elixir has many features that make it an attractive language for development. It is a functional language and comes with great pattern matching capabilities. It also has a process-oriented approach which allows for great concurrency. Additionally, Elixir has a built-in macro system and extensibility, and comes with a wide range of libraries like OTP, mnesia, and Ecto.
Usage Examples
Elixir can be used for a wide range of development projects, ranging from web and mobile applications to embedded systems and real-time data processing.
Web Applications
Elixir is well-suited for developing web applications. It can be used with frameworks such as Phoenix to build fast and efficient web apps. Additionally, Elixir has great scalability and fault tolerance, meaning it can easily handle large amounts of traffic.
Mobile Applications
Elixir can also be used to create mobile applications. It can be used along with the React Native framework to develop cross-platform mobile applications. This can make it easier to deploy on both Android and iOS platforms. It is also suitable for testing, due to its agile nature.
Embedded Systems
Elixir is becoming increasingly popular for its use in embedded systems. It can be used in combination with the Nerves framework to create robust and reliable embedded systems. Additionally, Nerves makes it easy to integrate hardware components into an Elixir application.
Real-Time Data Processing
Elixir is well-suited for real-time data processing due to its high performance, scalability, and fault tolerance. It can be used with frameworks such as GenStage to handle streaming data and process events as they occur. This makes it perfect for applications that require near-instant responses.
Code Examples
Here are a few examples of how to use Elixir:
Hello World
The following code will print “Hello world!” to the console:
IO.puts "Hello world!"
Pattern Matching
Elixir’s powerful pattern matching can be used for efficient data extraction:
[x, y, z] = [1, 2, 3]
IO.puts "#{x}, #{y}, #{z}"
This code will output “1, 2, 3” to the console.
Concurrency
Elixir’s process-oriented approach makes writing concurrent programs easy. Here is an example of spawning two processes and sending a message between them:
parent_pid = self()
spawn fn -> send(parent_pid, {:msg, "hello"}) end
receive do
{:msg, body} -> IO.puts body
end
This code will print “hello” to the console.
Hello World
IO.puts("Hello, World!")
This code will print “Hello, World!” to the terminal.
String Interpolation
Elixir allows you to interpolate variables into strings using the #{}
syntax. For example:
name = "John"
IO.puts("Hello, #{name}!")
This will print “Hello, John!” to the terminal.
Pattern Matching
Elixir also supports pattern matching for assigning values to variables. For example:
[first, second, _] = ["a", "b", "c"]
IO.puts(first)
# Output: "a"
IO.puts(second)
# Output: "b"
This code assigns the first two elements of the list to first
and second
, and then prints them to the terminal.
Elixir encourages functional programming, meaning you can use functions as arguments to other functions. For example:
def multiply(a, b) do
a * b
end
def double(a) do
multiply(a, 2)
end
double(2)
# Output: 4
This code defines the multiply
and double
functions, which are then used to calculate a value.
Conclusion
Elixir is a powerful language that is well suited for developing highly concurrent and distributed applications. Its robust libraries and low-level code performance make it ideal for tasks such as web development, data processing and machine learning. While it may take some time to learn, Elixir can provide excellent performance and scalability for your applications.