GO
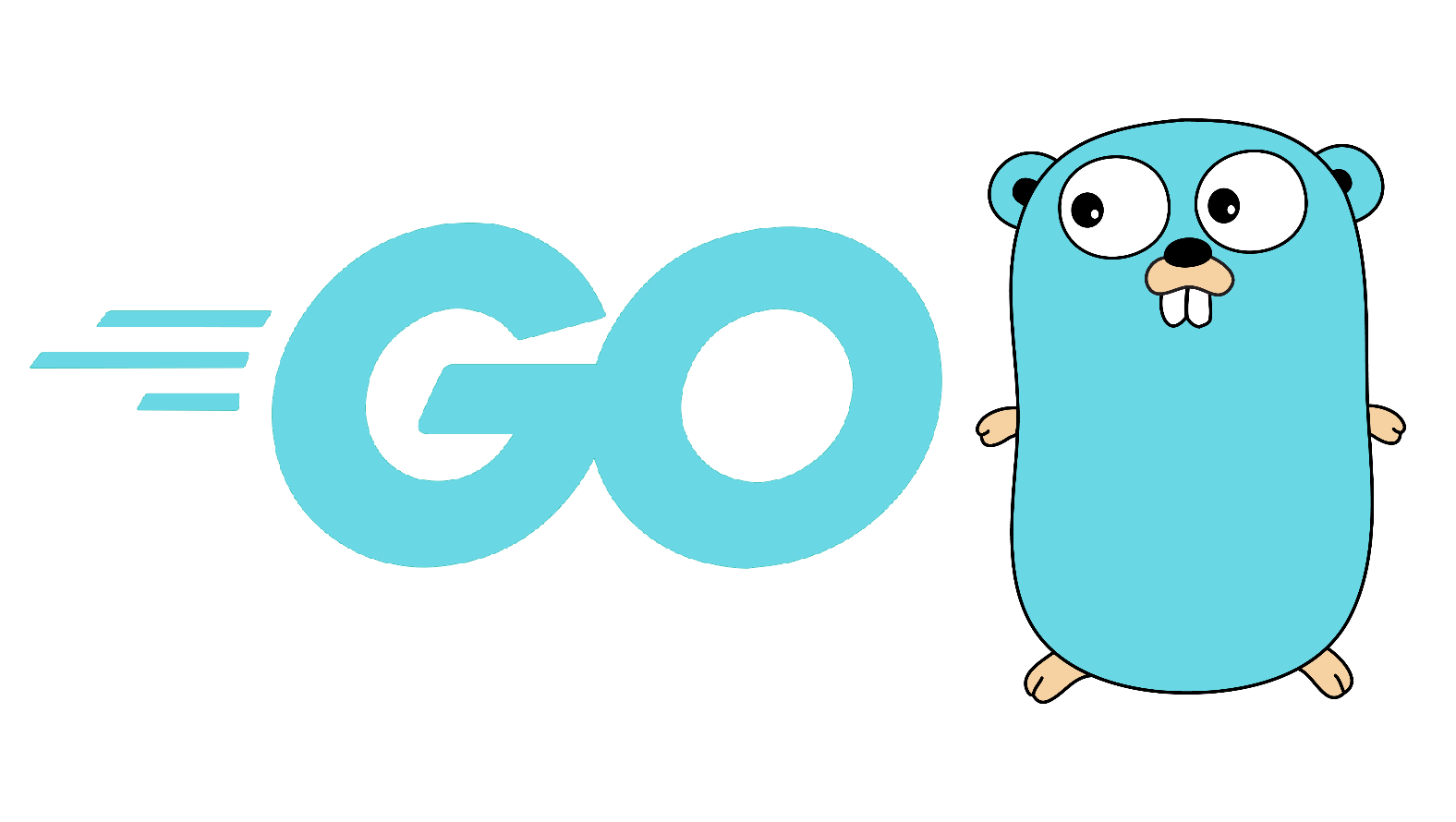
GO
Go is an open source programming language created at Google in 2009. It was designed to be a simple, efficient, and expressive language that would be suitable for writing code on the server side and on distributed systems. Go, also known as golang, is a programming language developed at Google in 2009. It is a compiled, statically-typed language designed with high performance, concurrency and low latency in mind. Go was designed with the goal of providing an alternative to C, a language that is renowned for its power and efficiency but which is considered difficult to learn and master by many. Go is designed to be simpler and easier to read while still offering a great deal of power and flexibility, allowing developers to quickly create efficient and reliable software applications. The official language specification for Go was first published in 2012, and it has since gained traction among developers and programmers looking for a robust language to build applications with. It has been adopted by many well-known companies, such as Dropbox, Netflix, DigitalOcean, and Bitly, and is the language behind popular projects such as Kubernetes, Docker, and Hyperledger Fabric. Go is often referred to as a general-purpose language, as it can be used for a wide variety of programming tasks such as web development, mobile development, system programming, and even data science. Due to its speed and scalability, it is often considered a good choice for large-scale applications and distributed systems. One of the most attractive aspects of Go is its straightforward syntax and clear structuring. As an example, consider the following code snippet:
History
Go was first announced by Google in November 2009, and released in March 2012. Its development was led by Robert Griesemer, Rob Pike, and Ken Thompson, all of whom had previously worked on the Unix operating system. In the years since its release, Go has become increasingly popular, with many large companies such as Uber, Netflix, and Dropbox switching from other languages to Go. It has also been adopted by many open-source projects, such as Kubernetes and Docker.
Examples
Go can be used for a wide variety of tasks, from writing web servers to creating distributed systems. Here are a few examples that demonstrate how powerful the language can be:
- Web applications: Go makes it easy to write web applications, with its built-in http package and powerful templating system.
- Microservices: Go’s lightweight nature makes it ideal for writing microservices. These small services can be spread across multiple machines and still communicate quickly and reliably.
- Network programming: Go’s inbuilt networking libraries make it easy to write network clients and servers. This makes it an ideal language for building distributed systems such as chat bots, search engines, and peer-to-peer networks.
- Machine learning: Go has excellent support for scientific computing and machine learning. The gonum library provides powerful data structures and algorithms for numeric computing, while golearn and Gorgonia provide tools for deep learning.
History
Go was first announced by Google in November 2009 and released as an open source project in March 2012. It was developed by Robert Griesemer, Rob Pike, and Ken Thompson. The language was designed to combine the performance of low-level languages like C and C++ with the safety and reliability of high-level languages like Java and Python. Go has been increasingly adopted by the software development community over the past decade. Many popular web frameworks have been written in Go, including Rails, Django, and Express. The language has also been used to build powerful applications such as Kubernetes and Docker.
Features
Go has several features that make it appealing for developers. These include:
- Static typing: Go is statically typed, meaning that the type of a variable is determined when it is declared rather than at runtime. This improves the readability and maintainability of code.
- Concurrency: Go makes it easy to write code that can execute multiple tasks simultaneously, which is known as concurrency. This is done with goroutines and channels, which allow multiple tasks to communicate and share data without contention.
- Garbage collection: Go has a garbage collector that runs in the background to clean up unused memory, so developers don’t have to worry about manually managing memory.
- Rich standard library: Go comes with a rich standard library, which provides basic functions such as network communication, file manipulation, and testing tools.
Advantages
GO has many advantages over other languages, including:
- Simplicity: GO is easy to learn, read, and maintain;
- Speed: GO programs compile and run quickly, making it ideal for building high-performance applications;
- Cross-platform support: GO can be used to create programs that can run on multiple platforms;
- Scalability: GO makes it easy to scale applications without making significant changes to the code.
Usage examples
Go can be used for a variety of tasks, from web development to DevOps. Here are some examples:
- Web Development: Go provides a robust web development framework for creating full-stack web applications. Examples include the popular web frameworks Rails, Django, and Express.
- DevOps: Go can be used to automate and streamline DevOps tasks, such as infrastructure provisioning and application deployment.
- Data Science: Go can be used to develop data-driven applications, such as machine learning models and natural language processing tools.
- Microservices: Go is well-suited for building microservices, which are small, self-contained applications that run in the cloud.
Code Examples
Here are some examples of Go code:
Hello World
package main
import "fmt"
func main() {
fmt.Println("Hello World!")
}
Goroutine
package main
import (
"fmt"
"time"
)
func say(msg string) {
for i := 0; i < 3; i++ {
fmt.Println(msg)
time.Sleep(time.Millisecond * 100)
}
}
func main() {
go say("hey")
go say("there")
time.Sleep(time.Millisecond * 200)
}
HTTP Server
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprint(w, "Hello World!")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
Hello world
package main
import "fmt"
func main() {
fmt.Println("Hello, world!")
}
This code will print out the string Hello, world!
. As you can see, this program is very easy to understand and is
properly structured. This makes it a great language for beginners and experienced programmers alike.
Additionally, Go provides built-in support for error handling, allowing developers to easily write robust and reliable programs. Here’s an example:
func main() {
value, err := someFunction()
if err != nil {
// Handle the error here
} else {
// Do something with 'value'
}
}
Finally, Go comes with excellent tooling. For example, the go command line tool offers a number of useful commands that allow developers to quickly and easily manage their projects. Additionally, it offers interactive debugging and profiling tools and integrates with popular testing frameworks.
In summary, Go is a powerful, statically-typed language designed for high performance and scalability. It offers a clean, intuitive syntax and excellent tooling, making it a great choice for developers of all levels. Its growing popularity and widespread adoption make it a great choice for many types of projects.
The following code shows a basic HTTP server written in Go:
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello World!")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This code will create an HTTP server that listens on port 8080 and responds to requests with the string “Hello World!”.
The following code shows a simple function that adds two numbers together:
package main
func add(a, b int) int {
return a + b
}
func main() {
sum := add(1, 2)
fmt.Println(sum) // prints 3
}
This code adds two numbers together and prints the result.
Here is an example of a basic GO program:
package main
import "fmt"
func main() {
fmt.Println("Hello World!")
}
This program will print “Hello World!” when run.
Summary
GO is an open-source programming language created in 2009 by Google. It is known for its scalability, high performance, and flexibility. It is primarily used for creating distributed applications and web services. GO has become a popular language choice for many software development tasks due to its simplicity, speed, and cross-platform support. GO is used for many tasks, including web development, data analysis, machine learning, and game development.