PostgreSQL
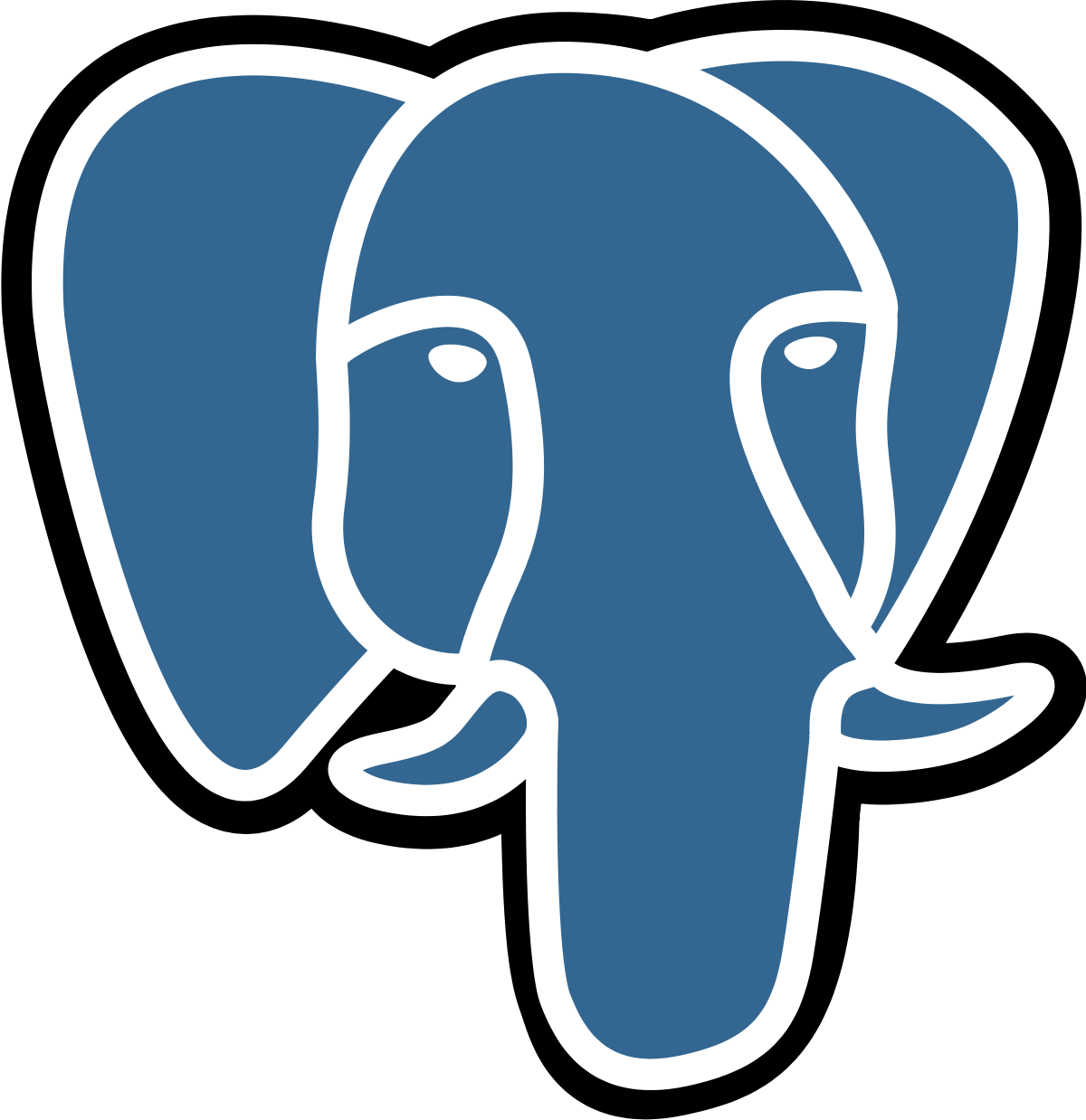
Introduction
PostgreSQL is an open-source object-relational database management system (ORDBMS). It is designed to provide an enterprise-class database solution that can handle the most demanding workloads. PostgreSQL is based on an object-oriented data model, while other popular databases are mostly relational data stores. It provides a wide range of features and capabilities, such as advanced query optimization, stored procedures, triggers, and support for complex data types. It also has the ability to replicate databases across multiple servers and offers a wide variety of APIs and languages for application development.
History
PostgreSQL was created in 1996 as a fork of the Ingres project by a team led by Michael Stonebraker. Initially, the project was called Postgres 95 and was an extended version of the original Postgres project. In 1997, the project was renamed to PostgreSQL and the first major release (version 6.0.2) was released in 1998. The PostgreSQL project has since grown in popularity and is now the most advanced open source database available for download. By 2013, PostgreSQL had become the 4th most popular database in the world, behind Oracle, Microsoft SQL Server, and MySQL, according to DB-Engines.com. PostgreSQL was originally developed at the University of California, Berkeley in the late 1980s. It was then released as an open source project in 1996, and since then has been steadily improved and expanded upon. Today, PostgreSQL is the world’s most advanced open source database, with millions of users around the world relying on it for their data storage and analysis needs.
Origins
The original Postgres project was created at the University of California, Berkeley in 1986. Computer science Professor Michael Stonebraker partnered with a few graduate students to develop the project. The aim was to develop a relational database system that could handle complex queries. The project’s first version, known as Postgres, was released in 1989. This version used a query language called POSTQUEL. The name “Postgres” is a combination of the words “post-structured query language.” In 1994, Stonebraker and others released the first version of PostgresSQL, which was based on an improved query language called SQL. The team continued to refine and develop PostgresSQL over the years, introducing new features such as transactions, stored procedures, and user-defined types. In 1996, PostgresSQL became a wholly-owned subsidiary of Enterprise DB, Inc., a company dedicated to developing software solutions based on PostgresSQL.
Adoption and Popularity
Since its inception, PostgresSQL has grown in popularity and is now one of the most widely used relational databases in the world. It is used in a variety of industries, ranging from finance to web development. Over the years, PostgresSQL has become increasingly popular due to its robustness, scalability, and ease of use. It offers an extensive range of features, including support for multiple languages, advanced security measures, and high availability solutions. PostgresSQL is also highly extensible and can be extended with custom functions, procedures, and data types. It supports a wide range of programming languages, including Java, Python, and PHP.
Uses of PostgreSQL
PostgreSQL is widely used in many different situations. It is often used as a replacement for commercial databases such as Oracle or Microsoft SQL Server. It is also used in web applications, data warehousing, geographic information systems, and data analysis. PostgreSQL is particularly well suited for large-scale data warehousing and analytics applications. It is highly scalable, supports very large volumes of data, and can be used for both online transaction processing (OLTP) and OLAP applications. PostgreSQL is also used in various scientific, engineering, and financial applications. It is popular in bioinformatics, physics, and astrophysics, as well as in finance and banking.
Features of PostgreSQL
PostgreSQL has a number of features that make it a powerful and flexible database. These include:
- Advanced query optimization and parallel query execution
- Extensible type system
- Support for data types, including JSON and custom types
- Full-text search capabilities
- Triggers and stored procedures
- Transaction isolation levels
- Materialized views
- Foreign data wrappers
- Multi-version concurrency control
Usage Examples
Web Applications
PostgreSQL can be used as the backend for web applications, allowing developers to store and manage data and build dynamic web experiences. Popular frameworks like Django and Ruby on Rails make use of PostgreSQL’s features to provide developers with powerful tools for building modern web apps.
Data Warehousing
PostgreSQL can also be used as a data warehouse solution. It offers easy scalability and the ability to query large datasets quickly and efficiently. With its range of data types and powerful indexing capabilities, PostgreSQL can be used to effectively mine and analyze massive datasets.
Analytics
PostgreSQL is an ideal platform for analytics and data science, as it offers a wide range of analytic functions such as aggregations, window functions, and statistical operations. It provides developers with the flexibility to perform complex queries and analyses on large datasets.
Geospatial
PostgreSQL is well-known for its powerful geospatial features, which allow users to store, query, and visualize geographic data with ease. It supports various data formats, from shapefiles to vector tiles, and has several built-in geospatial functions to simplify your queries.
Code Examples
Below are some examples of how to use PostgreSQL in your applications.
Creating a Table
To create a table in PostgreSQL, you use the CREATE TABLE
statement. For example, to create a table named users
with
three columns id
, name
, and email
, you can use the following code:
CREATE TABLE users
(
id serial PRIMARY KEY,
name varchar(50) NOT NULL,
email varchar(50) NOT NULL
);
Querying Data
To query data from a table in PostgreSQL, you use the SELECT
statement. For example, to select all records from
the users
table, you can use the following code:
SELECT *
FROM users;
Updating Data
To update existing data in a table in PostgreSQL, you use the UPDATE
statement. For example, to update the name
column of the record with ID 2 in the users
table, you can use the following code:
UPDATE users
SET name = 'John'
WHERE id = 2;
Inserting Data
To insert new data into a table in PostgreSQL, you use the INSERT
statement. For example, to add a new record to
the users
table, you can use the following code:
INSERT INTO users (name, email)
VALUES ('Jane', 'jane@example.com');
Deleting Data
To delete data from a table in PostgreSQL, you use the DELETE
statement. For example, to delete the record with ID 3
from the users
table, you can use the following code:
DELETE
FROM users
WHERE id = 3;
Here is a simple code example of how to connect to a PostgresSQL database:
import psycopg2
conn = psycopg2.connect(host='localhost', dbname='mydb', user='postgres', password='pass')
cur = conn.cursor()
cur.execute('SELECT * FROM mytable')
data = cur.fetchall()
for row in data:
print(row)
conn.close()
Create a Table
This example creates a table called students
with the following fields:
CREATE TABLE students
(
id serial primary key,
name varchar(50),
email varchar(50)
);
Insert a Record
This example inserts a new record into the students
table:
INSERT INTO students (name, email)
VALUES ('John Doe', 'john@example.com');
Select a Record
This example selects all records from the students
table:
SELECT *
FROM students;
Update a Record
This example updates the email
field for a record in the students
table:
UPDATE students
SET email = 'john.doe@example.com'
WHERE id = 1;
Delete a Record
This example deletes a record from the students
table:
DELETE
FROM students
WHERE id = 1;
Conclusion
PostgreSQL is a powerful and feature-rich RDBMS that is widely used in many areas. It is highly extensible, scalable, and fault tolerant, making it ideal for large-scale data processing and analysis. In addition, PostgreSQL supports a wide variety of programming languages, making it easy to integrate into existing applications. Finally, PostgreSQL provides comprehensive security features, making it a secure and reliable choice for data storage and processing.