NodeJS
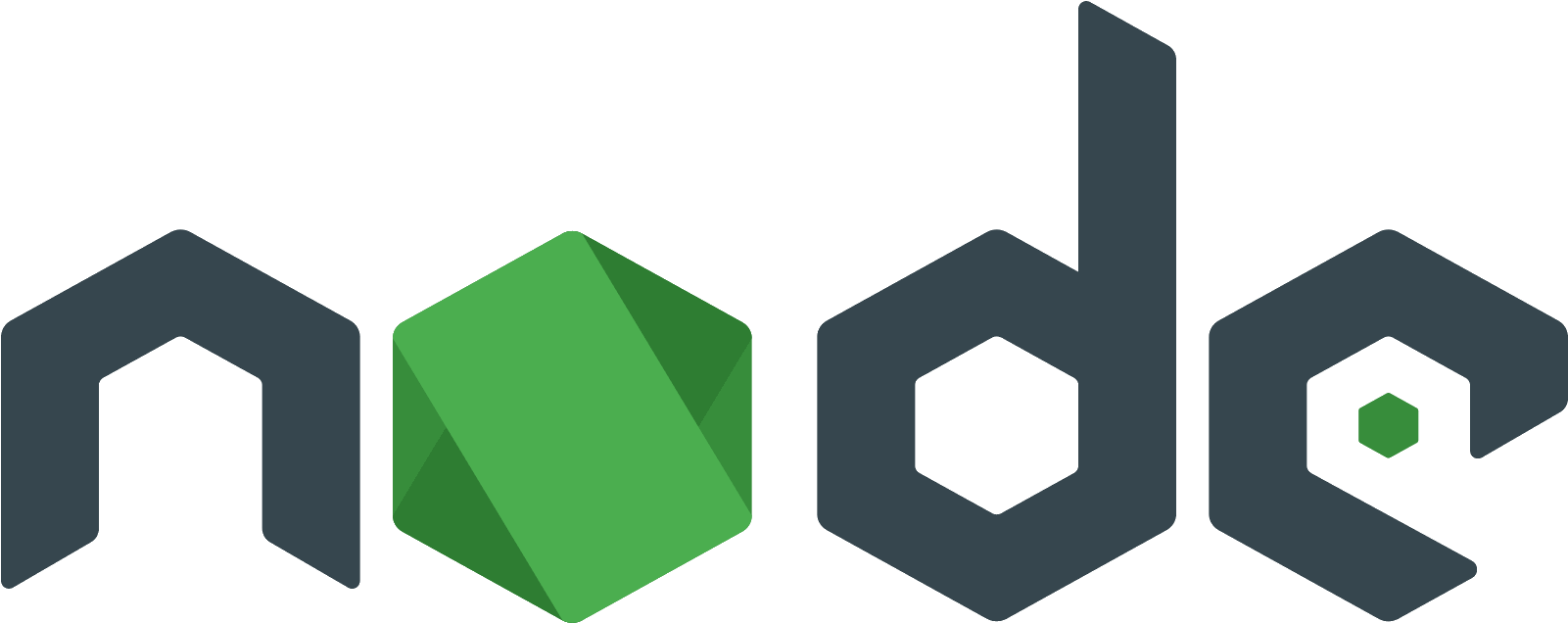
What is Node.js?
Node.js is an open-source, cross-platform JavaScript run-time environment for executing JavaScript code server-side. Node.js is available for desktop and other versions of Windows, macOS, Linux, SmartOS, and Solaris. Node.js is often used for building web applications and APIs, and it’s also popular for its non-blocking, event-driven architecture.
History of Node.js
The first version of Node.js was released in May 2009. According to its creator, Ryan Dahl’s original plan for the project was to build a network application platform similar to Apache HTTP Server. The main goal of Node.js at that time was to provide a way for developers to easily create real-time web applications. In 2010, Node.js added the CommonJS module system which made it easier for developers to share code between browsers and servers. In 2011, Node.js added the Buffer API which allowed developers to store binary data within Node.js applications. The same year, Node.js was used to power a web framework called Express which is still popular today. In 2012, Node.js introduced the REPL (Read-Eval-Print-Loop) feature which allows developers to quickly test out code without needing to create an entire program. In 2013, Node.js moved to the open source project NPM (Node Package Manager) which helps developers quickly and easily install packages from the Node.js repository. From 2014 onwards, Node.js has continued to grow in popularity and has become one of the most popular server-side runtime environments.
Features of Node.js
Node.js allows developers to write server-side code in JavaScript and use popular JavaScript libraries like jQuery and React. Additionally, Node.js offers an event-driven architecture that allows developers to easily create scalable applications.
Node.js also provides a rich set of features including:
- Asynchronous I/O: Node.js can handle multiple concurrent connections much more efficiently than traditional web servers.
- Strong Security: Node.js provides the tools and frameworks necessary to build secure applications.
- Flexibility: Node.js can be used to develop both client-side and server-side applications.
- Cross-Platform Compatibility: Node.js can run on Windows, Mac OS X, and Linux operating systems.
- Scalability: Node.js makes it easy to scale applications horizontally and vertically by using clusters and services like Azure and Amazon Web Services.
Adoption
Node.js is being used to build a wide range of applications. Some popular examples include:
- Real-time Collaborative Applications: Node.js can be used to build collaborative applications such as chat rooms and multi-user drawing programs.
- Online Gaming: Node.js can be used to build online multiplayer games.
- Video Streaming: Node.js can be used to create applications for streaming video, such as Netflix and YouTube.
- Social Networks: Node.js can be used to build social networking sites such as Facebook and Twitter.
- IoT Applications: Node.js can be used to build applications that interact with smart devices, such as home automation systems.
Why is Node.js Popular?
Node.js has become popular due to its ability to handle large volumes of data in an efficient manner. Node.js is also highly extensible, allowing developers to easily build new modules and applications. The ability to quickly develop and deploy applications makes Node.js a great language for prototyping, as well as for developing complex, cloud-based applications.
Node.js is also efficient and lightweight, making it ideal for applications that require low latency or intensive computations. Because of its low memory footprint, Node.js is also suited for applications that involve microservice architectures. Additionally, the asynchronous nature of Node.js provides developers with better scalability and performance, making it a great choice for applications that require real-time communication and streaming.
Advantages of Node.js
Node.js is an excellent choice for building web applications and services because of its high performance and scalability. Node.js is also lightweight, easy to learn, and has a vibrant community of developers. Here are some of the advantages of using Node.js:
- High performance: Node.js applications are highly efficient and can handle thousands of concurrent connections with low latency.
- Scalability: Node.js is highly scalable, making it easy to build large and complex web applications.
- Lightweight: Node.js is lightweight and does not require a lot of resources to run.
- Easy to learn: Node.js is easy to learn, and there are many tutorials and example code available to help developers get started quickly.
- Vibrant community: Node.js has an active and vibrant community of developers who share tips and advice, and help out fellow developers.
Usage examples
Node.js can be used to build a wide variety of applications, from simple web apps to complex enterprise systems. Some popular uses for Node.js include:
- Web applications & APIs: Node.js is a popular choice for developing web applications and APIs, thanks to its scalable and high-performance capabilities.
- Mobile applications: Node.js can be used to develop native mobile applications and hybrid mobile applications.
- Real-time applications: Node.js is well-suited for applications that require real-time communication and streaming.
- Database management: Node.js can be used to manage, query, and interact with databases, such as MongoDB and SQL.
- Internet of Things (IoT) applications: Node.js is often used to build IoT applications thanks to its scalability and efficiency.
Code Examples
Below are some examples of Node.js code.
Hello World
The following code prints “Hello World!” to the console.
console.log("Hello World!");
HTTP Server
The following code creates a simple HTTP server that listens on port 3000
and prints “Hello World!” when a request is received.
const http = require('http');
const hostname = '127.0.0.1';
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World!\n');
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
File System
The following code prints the contents of a file to the console.
const fs = require('fs');
fs.readFileSync('filename.txt', 'utf-8', (err, content) => {
if (err) throw err;
console.log(content);
});
Below is a simple example of a Node.js application that uses Express to respond to an incoming HTTP request.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => {
console.log('Example app listening on port 3000!');
});
This code will create a web server that listens on port 3000 and responds with “Hello World!” when a request is sent to the /
endpoint.
##Examples of NodeJS Usage
NodeJS can be used to create powerful applications, both on the client-side and the server-side. Here are some examples of NodeJS usage:
Web Development
NodeJS is often used for web development, as it enables developers to create highly scalable, performant web applications. With NodeJS, developers can use JavaScript for both the front-end and the back-end of their web application. This makes it easier to create applications that are maintainable and extensible. NodeJS also provides features such as routing, templating, and others which make it easier to create dynamic web applications.
Server-Side Development
NodeJS can be used for server-side development, allowing developers to create powerful applications on the server-side. NodeJS offers a number of features such as asynchronous I/O, event-driven programming, and more, which makes it ideal for creating scalable applications. NodeJS can be used to create web services, APIs, microservices, and more.
Real-Time Applications
NodeJS can be used to create real-time applications, such as chat applications, gaming applications, and more. NodeJS provides features such as event-driven programming and real-time communication protocols, making it ideal for creating real-time applications.
Data Streaming Applications
NodeJS can be used to create data streaming applications, such as video streaming applications and audio streaming applications. NodeJS provides features such as event-driven programming, real-time communication protocols, and more, making it ideal for creating streaming applications.
IoT Applications
NodeJS can be used to create Internet of Things (IoT) applications, such as sensors, home automation systems, and more. NodeJS provides features such as asynchronous I/O, real-time communication protocols, and more, making it ideal for creating IoT applications.
Mobile Applications
NodeJS can be used to create mobile applications, by using popular tools such as React Native and Expo. This enables developers to create mobile applications using the same language, making it easier to maintain the codebase.
NodeJS Code Examples
Here are some simple NodeJS code examples to illustrate its usage:
Creating a HTTP Server
The following code example shows how to create a basic HTTP server using NodeJS:
const http = require('http');
const hostname = '127.0.0.1';
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World!');
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
Creating an Express App
The following code example shows how to create an Express application using NodeJS:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => {
console.log('Example app listening on port 3000!');
});
Creating a Socket.io Server
The following code example shows how to create a Socket.io server using NodeJS:
const io = require('socket.io')();
io.on('connect', (socket) => {
socket.on('message', (message) => {
io.emit('message', message);
});
});
io.listen(3000);
The following code example creates a simple web server using Node.js.
const http = require('http');
const port = 3000;
const requestHandler = (request, response) => {
console.log(request.url);
response.end('Hello Node.js Server!')
};
const server = http.createServer(requestHandler);
server.listen(port, (err) => {
if (err) {
return console.log('something bad happened', err);
}
console.log(`server is listening on ${port}`);
});
This example creates a basic web server using Node.js. The code starts by importing the http
module, then creates a server using http.createServer()
. Finally, the server will listen on port 3000 and log any requests.
Conclusion
Node.js is a powerful and versatile platform for developing web applications and services. Node.js is lightweight, efficient, and easy-to-learn, making it a great choice for developers. Node.js is used by many major companies and its vibrant community provides support and resources for developers. If you’re looking for a platform for developing fast, scalable and efficient web applications, you should definitely give Node.js a try.