Python
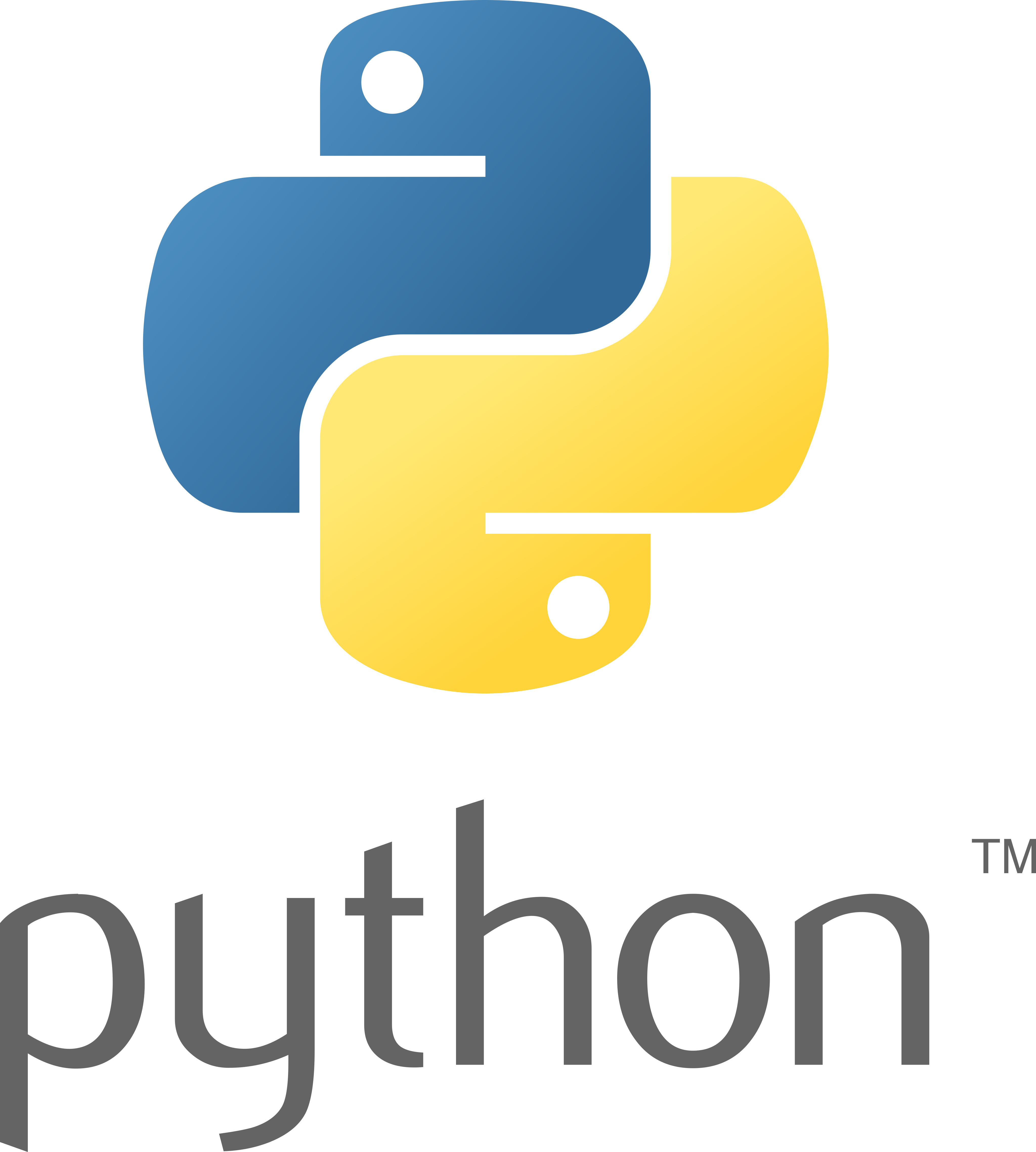
Python
Python is a high-level, interpreted, object-oriented programming language with dynamic semantics and a broad range of applications. It is used for web and app development, data science, machine learning, scripting, automation, and more. Python is an interpreted, high-level, general-purpose programming language. Created by Guido van Rossum and first released in 1991, Python has a design philosophy that stresses code readability, notably using significant whitespace. It provides constructs that enable clear programming on both small and large scales.
Python is a multi–paradigm programming language: object-oriented programming and structured programming are fully supported, and there are a number of language features which support functional programming and aspect-oriented programming (including by metaprogramming and metaobjects (magic methods)). Many other paradigms are supported via extensions, including design by contract and logic programming.
The language’s core philosophy is summarized in the document The Zen of Python, which includes aphorisms such as:
- Beautiful is better than ugly
- Explicit is better than implicit
- Simple is better than complex
Python features a dynamic type system and automatic memory management. It supports multiple programming paradigms, including object-oriented, imperative, functional and procedural, and has a large and comprehensive standard library.
Python interpreters are available for many operating systems. CPython, the reference implementation of Python, is open source software and has a community-based development model, as do nearly all of Python’s other implementations. CPython is managed by the non-profit Python Software Foundation.
History
Python was created by Guido van Rossum in 1989, with the goal of creating an easy to use language that could be read by both humans and machines. The name “Python” was inspired by the British comedy group Monty Python. Since its first release in 1991, Python has become one of the most popular programming languages in the world. Python was developed in the late 1980s, and its first version was released in 1991. Van Rossum continued working on it as a hobby in December 1989, and released it publicly in February 1991, with the name Python due to his love of Monty Python’s Flying Circus.
Van Rossum’s primary goal was to make a powerful, yet easy-to-use scripting language. His key design goals included:
- It should be easy to learn and use
- It should support multiple programming paradigms, such as object-oriented, procedural, and functional programming
- It should have a wide range of libraries for various tasks
- It should be extensible
Python was created in the late 1980s, and first released in February 1991, by Guido van Rossum, a Dutch programmer at CWI in the Netherlands, as a successor to the ABC programming language, which was inspired by SETL. Van Rossum’s guiding principle for Python’s development was “code readability”. His decision to make the language available as open source has had a great impact on its suitability for many types of projects. On October 16th 2000, Van Rossum announced he would step down as the leader in the Python community. In July 2018, Van Rossum stepped down as the Benevolent Dictator For Life, passing leadership of the project to a five-person steering council.
Features
Python has an extensive standard library that provides developers with tools, modules, and packages for a range of tasks, such as mathematical operations, web services, and database access. There are also many third-party libraries available for Python developers to use.
Python has excellent support for object-oriented programming, functional programming, and procedural programming. It also supports automatic type inference and garbage collection.
Python is a high-performance language, with powerful built-in data structures and dynamic typing. It has excellent readability and thus makes it easier for developers to debug and maintain their code.
Python has a wide range of uses, from web development and desktop applications to scientific applications, artificial intelligence, machine learning, and data analysis.
Usage
Web Development
Python can be used for server-side web development, with frameworks such as Django and Flask. These frameworks provide developers with tools for creating dynamic websites and applications that can be scaled and tailored to suit the requirements of any project.
Desktop Applications
Python is also used for developing desktop GUI applications. The most popular GUI frameworks for Python include PyQt, Tkinter, and wxPython. These frameworks provide a range of widgets, such as buttons, combo boxes, check boxes, and toolbars, that can be used to create a user interface.
Scientific Applications
Python is widely used in scientific computing, with libraries such as NumPy, SciPy, and matplotlib providing tools for numerical computation and data visualization. It can also be used for statistical analysis and machine learning.
Artificial Intelligence
Python is also used in artificial intelligence (AI) development, with libraries such as Keras, TensorFlow, and Scikit-learn providing tools for deep learning, natural language processing, and other AI tasks.
Data Analysis
Python is a popular choice for data analysis, with libraries such as pandas, scikit-learn, and matplotlib providing tools for exploratory data analysis and predictive modeling.
Code Examples
Here is an example of a basic “Hello World” program written in Python:
print("Hello World")
Here is an example of a simple calculator program written in Python:
# Take input from the user
num1 = int(input("Enter first number: "))
num2 = int(input("Enter second number: "))
# Choose operation
print("Choose operation")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
# Take input from the user
choice = int(input("Enter choice(1/2/3/4): "))
if choice == 1:
print(num1+num2)
elif choice == 2:
print(num1-num2)
elif choice == 3:
print(num1*num2)
elif choice == 4:
print(num1/num2)
else:
print("Invalid input")
Below are some code examples to demonstrate how Python can be utilized in various ways.
Web Development
# Import necessary modules
from flask import Flask
from flask import request
# Create a new Flask application
app = Flask(__name__)
# Define the “Hello World” route
@app.route('/hello')
def hello_world():
return 'Hello World!'
# Start the application
if __name__ == '__main__':
app.run()
Software Development
# Import necessary modules
from tkinter import *
# Create the main window
root = Tk()
# Add a button
button = Button(root, text="Click Me!", command=lambda: print("Hello World!"))
button.pack()
# Run the main loop
root.mainloop()
Scientific Computing
import numpy as np
# Create an array
arr = np.array([1, 2, 3, 4, 5])
# Perform a mathematical operation
result = arr * 2
# Print the result
print(result)
Server-side Scripting
# Import necessary modules
import cgi
import cgitb
from http import cookies
# Get the HTTP cookie value
cookie = cookies.SimpleCookie(os.environ.get("HTTP_COOKIE"))
user_id = cookie.get('user_id').value
# Print the result
print("You are currently logged in as ", user_id)
Game Development
# Import necessary modules
import pygame
# Initialize pygame
pygame.init()
# Create a new surface
surface = pygame.display.set_mode((800, 600))
# Main loop
while True:
# Handle events
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Update the display
pygame.display.update()
Here are some examples of basic Python code:
Hello World
print("Hello World!")
Variable
message = "Hello World!"
print(message)
Loop
for i in range(10):
print(i)
Function
def say_hello(name):
print("Hello, {}!".format(name))
say_hello("John")
Popular Python Libraries
NumPy: NumPy is a library for scientific computing in Python. It contains functions for manipulating multi-dimensional arrays, performing mathematical operations, and linear algebra. Additionally, NumPy is used for manipulating images, numerical integration, and random number generation. Matplotlib: Matplotlib is a plotting library for Python that enables data visualization. It is used to create interactive figures and plots, including histograms, line plots, and bar graphs. Pandas: Pandas is a library for data manipulation and analysis in Python. It provides powerful data structures and tools for working with relational, tabular, and multidimensional datasets. It is often used for data cleaning and wrangling, data exploration, and analysis. Scikit-Learn: Scikit-Learn is a library for machine learning in Python. It provides tools for regression, classification, clustering, and dimensionality reduction. It is used for building models from data and making predictions. TensorFlow: TensorFlow is an open-source library for machine learning. It enables developers to build and train deep learning models with high accuracy and efficiency. It is used for tasks such as image classifications, natural language processing, and automatic speech recognition.
Conclusion
Python has become one of the most popular programming languages due to its versatile applications and easy-to-learn syntax. It is widely used in web development, data science, machine learning, and artificial intelligence, and its vibrant library ecosystem makes it easy to implement complex algorithms and tasks.