Ruby
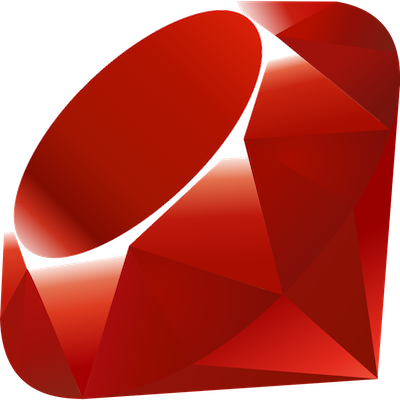
Introduction
Ruby is an open source, interpreted, object-oriented programming language with dynamic semantics that is designed to be not only enjoyable and easy to use, but also powerful. It is an object-oriented scripting language used by developers to create web applications, mobile applications, and data science libraries. It is a highly readable language that was developed by Yukihiro Matsumoto in the mid-1990s and has become one of the most popular languages for web development.
History
In the early 1990s, Yukihiro Matsumoto wanted to create a new language that blended elements from his favorite languages such as Perl, Smalltalk, Eiffel, Ada, and Lisp. This language would be more natural to computer users and thus easier to learn and use. He created the language he called “Ruby,” and it was first released to the public in 1995. Since its release, Ruby has grown in popularity, with many developers finding it to be a more elegant and expressive language than Java or C++. One of its key features is its object orientation, which makes it possible to write code that is both concise and easy to read. In addition, Ruby has a large community of developers who have created numerous libraries, tools, and frameworks, making it easier to develop applications quickly and efficiently. Ruby is a general-purpose programming language that has been around since the mid-1990s. Designed by Yukihiro “Matz” Matsumoto, it was intended to be an object-oriented scripting language. Over the years, Ruby has become popular among developers for its simplicity and flexibility. This article looks at the history of Ruby, its usage, examples of code written in Ruby, and some of the criticisms it has received over the years.
Ruby’s Origins
Ruby began as a personal project of Matsumoto, who wanted a programming language that was more powerful than Perl, but easier to use than Smalltalk. He began working on the language in 1993 and released the first version of the language in 1995. The language has experienced many changes and improvements over the years, including increasing its object-oriented features and optimizing its syntax. The most recent version of the language, Ru 3.1, was released in 2013.
Examples of Usage
Ruby is used to build web applications, mobile apps, data science libraries, and other software tools. For example, Ruby on Rails is a popular web application framework built with Ruby. It makes it easy to create complex, database-backed web applications quickly and efficiently.
Other popular Ruby libraries and frameworks include Sinatra, a lightweight web development library, and React, a library for developing user interfaces. Ruby is also widely used for creating data science projects, such as machine learning algorithms and natural language processing applications.
Code Examples
Here is a simple Hello World program written in Ruby:
puts "Hello, world!"
This code simply prints the string “Hello, world!” to the console.
Another example of Ruby code is a basic web application using the Sinatra framework. Here is the code for a simple ‘ Hello World’ web application:
require 'sinatra'
get '/' do
"Hello, world!"
end
The code above defines a route, which is a web address or path, and defines what will happen when someone visits this address. In this case, the code simply prints the string “Hello, world!” to the browser.
Here is a simple example of code written in Ruby:
def say_hello name
puts "Hello #{name}!"
end
say_hello "World"
This code creates a method called “say_hello” which takes a parameter called “name”, and prints out “Hello
Another example of code written in Ruby is a non-destructive version of the popular array “pop” method. This code checks the last elements of an array and removes it without changing the original array.
def pop arr
arr[-1]
arr
end
a = [1,2,3]
pop(a)
#=> [1,2,3]
The first line of code creates the method “pop” that takes an array as a parameter. The second line creates an array with three elements. The third line calls the method “pop” on the array. The result is that the last element is removed from the array, but the original array remains unchanged.
Popular Libraries for Ruby
There are a number of popular libraries for Ruby that can help make programming easier and more efficient. Some popular libraries include:
-
RSpec – RSpec is a popular library for testing Ruby applications. It provides support for behavior driven development and test driven development, making it easier to create robust code quickly.
-
ActiveRecord – ActiveRecord is an object-relational mapping (ORM) library for Ruby that simplifies the process of interacting with a database. It also supports conventions over configuration, meaning that developers do not have to write complex SQL queries to work with a database.
-
Feathers – Feathers is a library for creating APIs in Ruby. It provides support for the most popular web technologies such as REST, GraphQL and WebSocket, making it easy to create a powerful web application.
-
Rails – Rails is a popular web framework for Ruby. It provides support for models, views, controllers, and more, allowing developers to quickly create web applications without having to write all of the code from scratch.
Using Ruby Libraries
In order to use a Ruby Library, you will first need to install it on your computer. You can install Ruby Libraries using
the gem
command. For example, if you wanted to install the RSpec Library, you would run the following command:
gem install rspec
Once the library has been installed, you can then use it in your code by requiring it at the top of your file. For example, if you were using the RSpec Library, you would include the following line in your file:
require 'rspec'
After that, you can start accessing the various features and functions of the library.
If you are using the Rails Framework, you don’t need to manually install any libraries because it comes with its own set of libraries. In order to use them, you just need to require the necessary files in your code. For example, if you were using the ActiveRecord Library, you would include the following line in your file:
require 'active_record'
RSpec
RSpec is a popular testing library for Ruby. To use it, you first need to require the RSpec library in your file. After that, you can create a specification block and define your tests. For example, the following code defines a test that checks whether a given number is greater than another number:
require 'rspec'
describe "Number comparison" do
it "checks whether the number is greater than 100" do
expect(200).to be > 100
end
end
The above code will produce the following output:
Number comparison
checks whether the number is greater than 100
ActiveRecord
ActiveRecord is an ORM library for Ruby that simplifies the process of interacting with a database. To use it, you first
need to require the ActiveRecord library in your file. After that, you can create a model class that represents a table
in your database. For example, the following code defines a model class called Product
:
require 'active_record'
class Product < ActiveRecord::Base
# Model definition here
end
Once the model has been defined, you can then use it to perform various operations on the database. For example, the following code finds the first product in the database and prints out its name:
product = Product.first
puts product.name
Feathers
Feathers is a library for creating APIs in Ruby. To use it, you first need to require the Feathers library in your file. After that, you can create an endpoint by defining a handler. For example, the following code defines an endpoint that returns the current time:
require 'feathers'
get '/time' do
{ time: Time.now }
end
This code creates a new class called “Animal” and defines two methods:
class Animal
def initialize(name)
@name = name
end
def speak
puts "My name is #{@name}"
end
end
This code iterates over an array and prints out the values:
array = [1,2,3,4,5]
array.each do |n|
puts n
end
Criticisms of Ruby
Despite its popularity and ease of use, Ruby has not been immune to criticism. The main criticism has been around the performance of the language; compared to other languages such as Python and Java, it tends to be slower and heavier. The language has also been criticized for its lack of features and support for new technologies. With the rise of new technologies such as machine learning, many developers have been looking for languages that can offer better support for these technologies—Ruby has not yet been able to provide this kind of support. Finally, Ruby has been criticized for its lack of scalability. While it is well-suited for small projects, its scalability limits its ability to handle larger projects. As a result, many developers choose other languages when the project size increases.
Conclusion
Ruby is a powerful and popular programming language with an intuitive syntax and rich library of features. It is often used for web and application development, scripting, automation, and data analysis. This article has provided an overview of Ruby and examples of how it can be used.