R
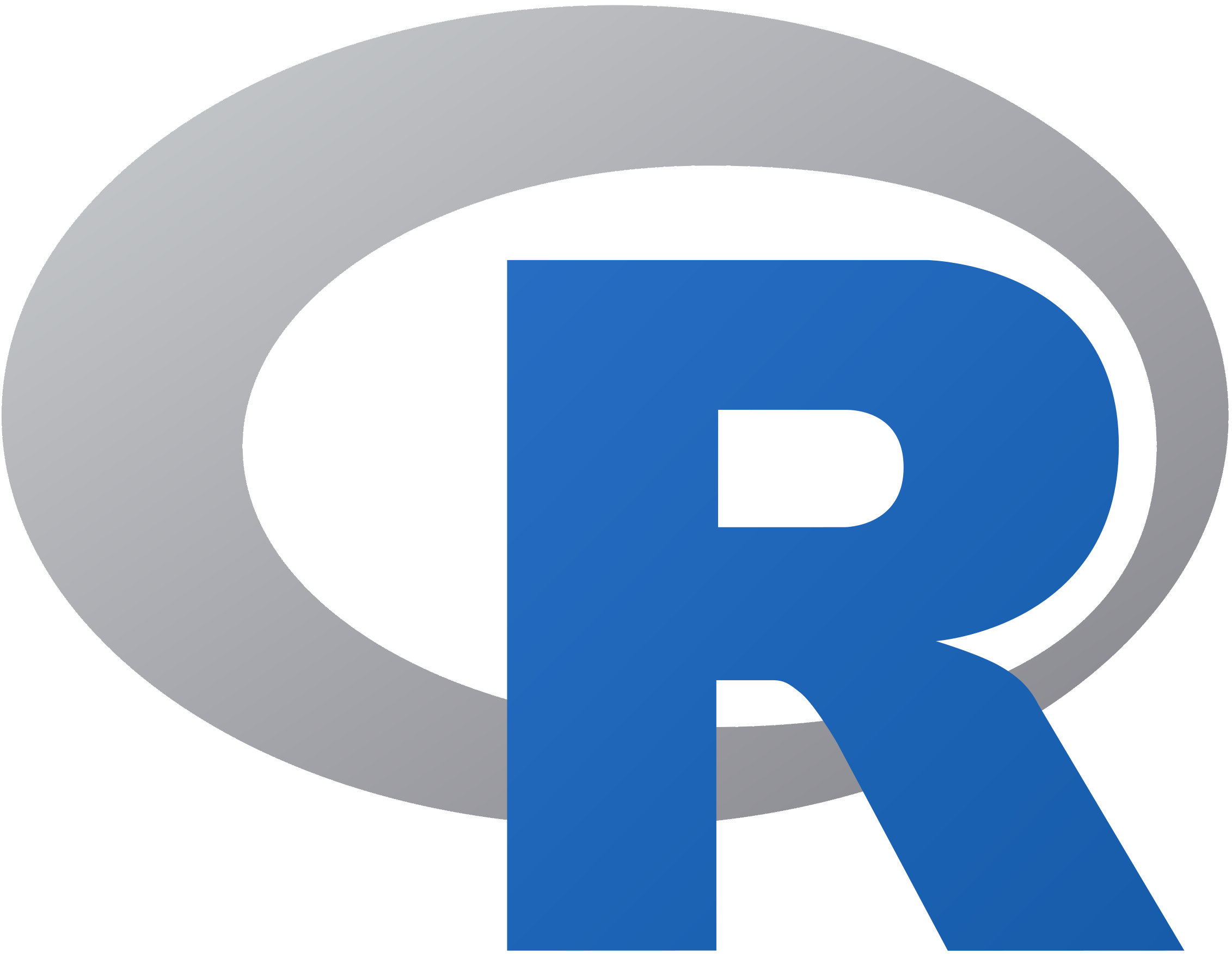
Introduction to R
R is a powerful, open-source programming language and environment for statistical computing and graphics. It is a popular choice for data analysis and statistical applications, as it is easy to learn and has a large library of statistical and graphical algorithms. It is often used by researchers in fields such as statistics, finance, and biology, and by software developers in a variety of industries.
History of R
R was originally developed in the early 1990s as an implementation of the S programming language by Ross Ihaka and Robert Gentleman at the University of Auckland in New Zealand. Initially, R was primarily used for statistical analysis, but over time it has grown to become a general-purpose language for data manipulation, analysis, and visualization. With its open source license, R is now widely available on various platforms, including Windows, Linux, and macOS. Since its creation, R has become an increasingly popular choice among statisticians, data scientists, and other data analysts. The language is regularly updated with new features, and a vibrant community of developers has created a wide array of third-party packages and libraries to complement the core language.
Features of R
R provides a wide range of features that make it useful for data analysis and visualization. It is an interpreted language, meaning that code is compiled and executed at run time rather than being precompiled. This makes it easier to debug and modify code, and also allows users to interactively explore data by writing short snippets of code. R is vectorized, meaning that most operations are performed on entire vectors of data rather than on single values. This makes it easy to perform bulk operations on large datasets quickly and efficiently. R is also a functional programming language, providing built-in support for functions, which can be passed as arguments to other functions and composed together to create more complex operations. This makes it easier to write reusable and extensible code. Finally, R has a wide range of built-in graphical features for data visualization, as well as a number of third-party packages for creating interactive web-based visualizations. This makes it easy to explore data and build interactive visualizations in a web browser.
Using R
R is typically used interactively in a command-line environment. This provides an intuitive way to try out code without having to compile and execute the code each time. Users typically write commands in the command-line environment and view the output in the terminal window. In addition to the command-line environment, there are several graphical user interfaces (GUIs) available for working with R. These GUIs provide a graphical editor for writing code, as well as tools for exploring data and creating visualizations. Popular GUIs for R include RStudio and R Commander.
R Packages
R includes a wide range of built-in functions and data structures, but its power comes from the large number of third-party packages available. These packages extend the core language with additional functions, algorithms, and data structures, as well as providing access to specialized databases and other resources. Popular packages for data analysis include dplyr for manipulating data frames, tidyr for reshaping data, and ggplot2 for creating powerful and interactive data visualizations. There are also packages for machine learning, such as caret for supervised learning, and keras for deep learning.
Adoption
R is a language and environment for statistical computing and graphics. It is widely used among data miners, statisticians, and researchers due to its diverse set of tools and powerful graphical capabilities. R is open-source and free, and allows users to easily extend its functionality with packages from the Comprehensive R Archive Network (CRAN). The use of R software in data analysis is becoming increasingly popular, as evidenced by the growing number of available packages. These packages provide powerful tools for manipulating data and creating sophisticated graphics. In this article, we will examine some of the most popular R libraries and discuss their key features.
Data Manipulation
The dplyr
package provides a suite of tools for manipulation of datasets. It includes the lapply()
function for quickly transforming data, select()
and filter()
functions for selecting specific rows and columns, and group_by()
and summarise()
functions for grouping and summarising data. dplyr
also provides built-in support for data frames, so that operations can be performed across multiple columns in one command.
The tidyr
library is designed to help tidy up messy datasets. It provides a series of functions that allow users to reshape, merge, and split data. For example, the gather()
function converts wide data into long data, and the spread()
function performs the opposite transformation. The spread()
function is especially useful when working with tables that contain multiple variables per row.
Graphics
The ggplot2
package is a popular library for creating compelling visualisations of data. It provides a range of plotting functions, including scatterplots, bar charts, line graphs, histograms, and density plots. ggplot2
also offers rich customization of elements such as labels, colours, themes, and faceting.
The lattice
library is another powerful plotting library, particularly well-suited to making array plots and 3D plots. lattice
offers several functions for producing Trellis plots, which are useful for visualising complex relationships between different variables.
For data visualisation, the ggvis
library provides an interactive environment for producing interactive visuals such as scatter plots, bar charts, and line graphs. It also integrates seamlessly with ggplot2
and lattice
, allowing users to combine the power of both libraries.
Statistics
The stats
package is one of the most comprehensive libraries for statistical analysis. It contains functions for a variety of tasks, such as linear and nonlinear modelling, time series analysis, and statistical tests.
The multcomp
library provides functions for conducting multiple comparisons and hypothesis testing. It includes a range of classical tests, such as Tukey’s test and Fisher’s Exact test, as well as modern methods such as bootstrap and permutation tests.
The car
library is designed to simplify common regression tasks, such as dealing with multicollinearity and calculating predicted values. It also provides a framework for conducting Diagnostic tests, such as Breusch–Pagan and White tests.
The mgcv
library provides a range of tools for fitting Generalised Additive Models (GAMs), which are used to describe relationships between response and predictor variables. GAMs allow for more flexible modelling by incorporating non-linear components into the model.
Examples
Here are a few examples of using R to analyze and visualize data:
Finding the mean of a dataset
The mean of a dataset can be computed using the built-in mean() function:
> dataset <- c(1,2,3,4,5)
> mean(dataset)
[1] 3
Plotting a histogram
A histogram of a dataset can be created using the built-in hist() function:
> hist(dataset)
Summarizing a dataset
The built-in summary() function can be used to generate a summary of a dataset:
> summary(dataset)
Min. 1st Qu. Median Mean 3rd Qu. Max.
1 2 3 3 4 5
Using a machine learning algorithm
The caret package can be used to train a machine learning algorithm on a dataset. For example, the following code fits a support vector machine (SVM) model to a dataset:
> library(caret)
> model <- svm(x = dataset, y = labels)
R is used for a wide variety of tasks, ranging from basic data analysis to advanced predictive modeling. Here are some examples of R’s use:
- Statistical Computing - R is used for a variety of statistical techniques such as linear and non-linear regression, time series analysis and statistical inference.
- Data Mining - R can be used to mine large datasets and uncover valuable insights that can be used to inform decisions.
- Machine Learning - R has a range of powerful machine learning and deep learning libraries, enabling users to develop complex models such as neural networks and decision trees.
- Visualization - R offers a range of packages for creating beautiful graphics and visualizations, enabling users to explore their data and find meaningful patterns.
Code example
Let’s look at an example of how you can use R to create a simple histogram using the ggplot2
library.
#install ggplot2
install.packages("ggplot2")
#load ggplot2
library(ggplot2)
#create some dummy data
data <- rnorm(1000)
#create a histogram
ggplot(data=data, aes(x=data)) +
geom_histogram()
The following code snippet shows how to use the dplyr
library to manipulate a dataset:
library(dplyr)
# Load a dataset
dat <- read.csv("dataset.csv")
# Select specific columns
dat <- select(dat, id, age, gender)
# Filter out rows based on a condition
dat <- filter(dat, age > 18)
# Group data by gender
dat <- group_by(dat, gender)
# Summarise data
summary <- summarise(dat, mean_age = mean(age))
# Print summary
print(summary)
And here is an example of how to use the ggplot2
library to create a scatter plot:
library(ggplot2)
# Load a dataset
dat <- read.csv("dataset.csv")
# Create a scatter plot
p <- ggplot(dat, aes(x=age, y=income)) +
geom_point() +
xlab("Age") +
ylab("Income")
# Print the chart
print(p)
Below are some examples of code in R:
# print a message
print("Hello World!")
# sum two numbers
x <- 1
y <- 2
z <- x + y
print(z)
# plot a scatterplot
x <- c(1,2,3,4,5)
y <- c(1,4,9,16,25)
plot(x, y)
Conclusion
R is an extremely versatile language that is widely used in many fields, from finance to sports analytics. Its popularity stems from its easy-to-use syntax, powerful visualizations, and extensive library of packages. With the help of R, data scientists, researchers, and analysts are able to make sense of their data in powerful and meaningful ways.