J#
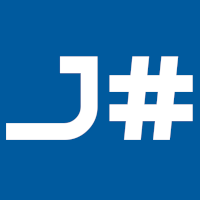
J#
J# is a general-purpose, object-oriented programming language developed by Microsoft. It is a .NET implementation of Java, intended to be a better replacement for the native C# language. Its syntax is similar to that of Java, with some features borrowed from C# and other .NET languages.
History
J# was introduced in 2002 as part of Microsoft’s .NET framework. It was an attempt to bridge the gap between Java, which had been gaining traction in enterprise development, and the popular C# language. The idea was to make it easier for developers to move from Java to the .NET platform, while also taking advantage of the new features that were available in .NET.
The language was included in the Visual Studio .NET 2003 and Visual Studio 2005 releases. Later versions of Visual Studio did not include J#, and Microsoft announced that it would no longer be supported in 2007. Despite this, many developers continue to use the language due to its stability and familiarity.
Features
J# offers many features that are similar to other languages such as Java, C#, and Visual Basic.NET. These include:
- Object-oriented programming – J# uses a class-based object-oriented programming model, just like Java and C#.
- Reflection – J# supports reflection, which allows programs to examine or modify themselves at runtime.
- Garbage collection – J# uses the .NET garbage collector for automatic memory management.
- Generic types – J# supports generic types, allowing type-safe exchange of information between components.
- Delegates – J# supports delegates, which are objects that can be used to call methods asynchronously.
- Events – J# supports events, which allow objects to communicate with each other.
In addition, J# also provides some features that are not found in other languages. These include:
- Windows Forms – J# provides built-in support for developing Windows Forms applications.
- XML and web services – J# provides support for creating and consuming XML documents and web services.
- COM Interoperability – J# supports interoperability with COM components as well as .NET components.
Usages
J# is mainly used for developing desktop applications, especially those that need to be cross-platform compatible. It can also be used to create web services, mobile apps, and even games. One example of a desktop application built with J# is a port scanner. This tool scans for open ports on a network and can be used for security purposes. Another example is a simple web service that returns a list of books. This could be used by a library management system to fetch book titles and authors from a database. Another example is a game development library that allows developers to create 2D games with ease. Examples include the popular Asteroids game or the classic Space Invaders.
Code Examples
Hello World
Here is a simple “Hello World” program written in J#:
public class HelloWorld
{
public static void Main()
{
System.Console.WriteLine("Hello World!");
}
}
This program prints the message “Hello World!” to the console.
Port Scanner
Here is an example of a port scanner written in J#:
public class PortScanner
{
public static void Main(string[] args)
{
// Parse the target IP address
IPAddress ipAddress = IPAddress.Parse(args[0]);
// Create a TCP client to connect to the target
TcpClient tcpClient = new TcpClient();
// Scan all ports
for (int port = 1; port <= 65535; port++)
{
try
{
// Try to connect to the current port
tcpClient.Connect(ipAddress, port);
// If we reached this point, the port is open
System.Console.WriteLine($"Port {port} is open.");
// Disconnect from the current port
tcpClient.Close();
}
catch (Exception ex)
{
// If we reached this point, the port is closed
//System.Console.WriteLine($"Port {port} is closed.");
}
}
}
}
This program takes an IP address as an argument and scans all ports from 1 to 65535. If a port is open, it prints a message to the console. J# can be used to create a wide variety of applications, including Windows Forms applications, console applications, web services, and web sites. Here is a simple example of a J# Windows application:
Conclusion
J# is an extension of the Java language that was created by Microsoft to help developers who had experience with Java to move their applications onto the .NET Framework. It has been used in many popular applications and offers developers the ability to reuse their existing code while still taking advantage of the features of the .NET Framework.