Groovy
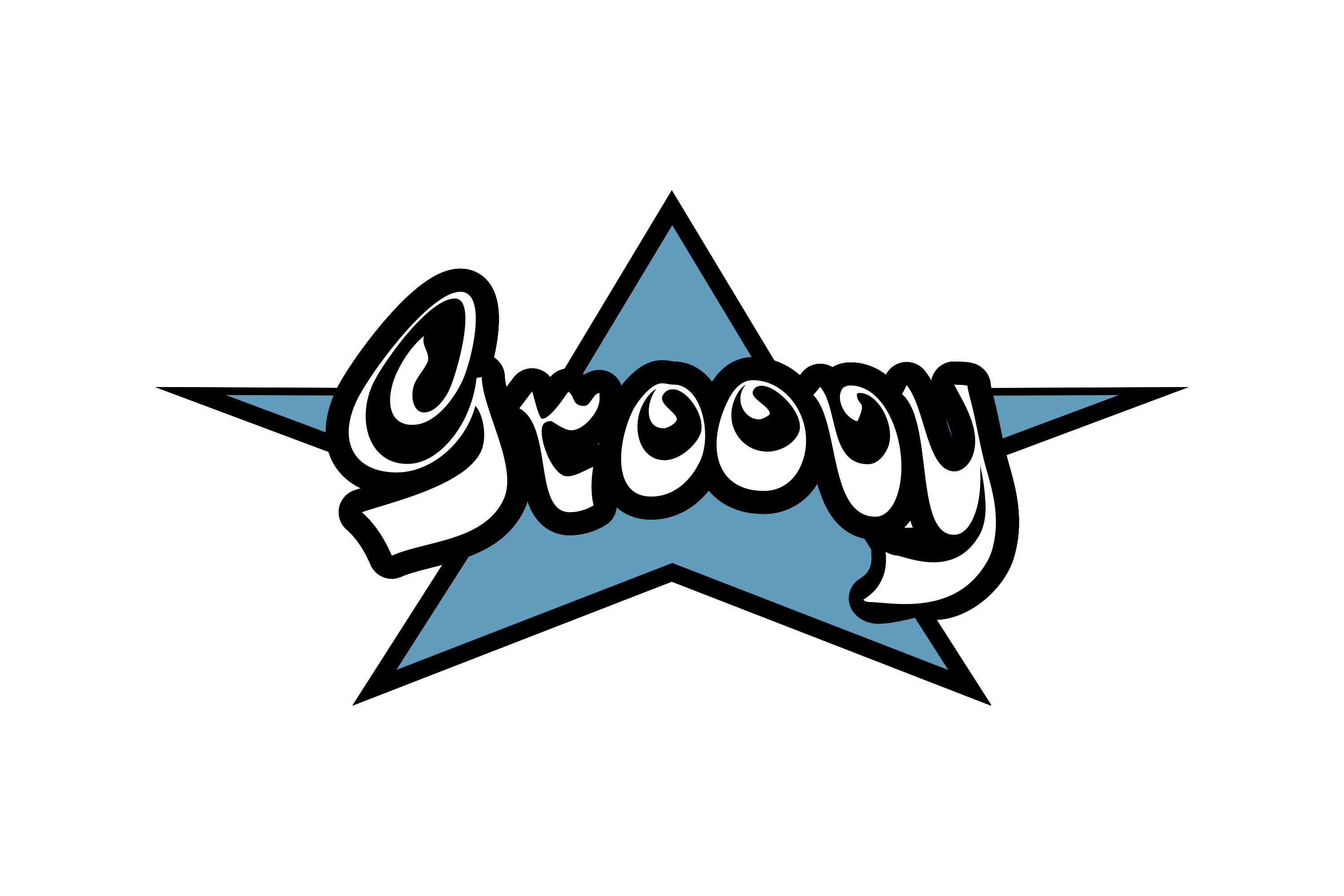
Introduction to Groovy
Groovy is an open source, dynamic programming language for the Java platform that was first released in January 2007. It is a scripting language and runs on the Java virtual machine. Designed to be easy to learn and use, it seeks to simplify Java development by offering features such as dynamic typing, closures, meta-programming, SQL and XML support, and more.
History of Groovy
Groovy was first created by Guillaume Laforge and Jochen Theodorou in 2003 as a static scripting language for the Java platform. Its development has been spearheaded by the Apache Foundation since late 2006 and its evolution to a modern dynamic language has been supported by a dedicated team of developers, as well as its vibrant community of users.
Today, Groovy is used in multiple past and future projects, from simple scripts to large scale web applications. Its flexibility, broad support for most popular programming languages, and its ability to easily integrate with existing code make it perfect for a number of contexts, including enterprise development.
Features of Groovy
At the core of Groovy is a collection of features that make it unique in the programming world. These features include:
- Dynamic typing: Groovy allows you to write code without explicitly declaring types, making it much easier to write and read.
- Closures: Closures are special blocks of code that can be used to improve code structure and readability.
- Meta-programming: Groovy provides powerful metaprogramming capabilities, allowing you to write code that writes code.
- Static and dynamic typing: Groovy supports both static and dynamic typing, allowing for greater flexibility in coding.
- Syntax variations: Groovy also offers a variety of syntax variations, allowing you to code in ways that may be more familiar to you.
- SQL and XML support: Groovy offers powerful SQL and XML support with minimal changes needed to existing code.
Usage
Groovy is commonly used for scripting, server-side web development, automation, building Domain Specific Languages (DSLs), and writing unit tests. For scripting, Groovy can be used to quickly write scripts to automate tasks, such as creating databases or setting up web servers. For server-side development, Groovy can be used to build web applications using the Grails framework or the Ratpack framework. Groovy can also be used to quickly create domain specific languages (DSLs) to define complex functionality in a concise and readable format. It is also used for writing unit tests with the Spock and JUnit frameworks.
Examples of Groovy Usages
Web Development
Groovy can be used in web development to speed up development time of web applications and to add features not available in standard web technologies. With Groovy’s powerful meta-programming and closure support, developers can create dynamic web applications using a fraction of the code needed for traditional web development.
Mobile Development
Groovy can also be used for mobile development, allowing for the development of native Android and iOS apps with minimal effort and maximum power. By taking advantage of the scripting language’s dynamic nature, developers can quickly create sophisticated native apps.
Enterprise Programming
Groovy is often used in enterprise programming to greatly reduce development time. Thanks to its integration with Java, Groovy can access existing code and libraries with minimal effort, making it the perfect choice for enterprise development.
Testing
Groovy can be used for automated testing, allowing testers to quickly write and execute tests in a fraction of the time. With its powerful integration with Java, Groovy allows testers to quickly create complex tests and compare the results.
Code Example
Here is a simple example of a Hello World program written in Groovy:
println "Hello World!"
This code will output the message “Hello World!” when executed.
Hello World
A common example of the usage of Groovy is the “Hello World” program. This program prints out the string “Hello World” when executed.
Here is an example of the code:
// Hello World in Groovy
println "Hello World"
Closures
Closures are important concepts in Groovy. Here is a simple example of how to use closures in Groovy:
def numbers = [1,2,3,4,5]
numbers.each {
println "Number: ${it}"
}
In this example, the each
method is used to iterate over each item in the list numbers
. The closure represented by { println "Number: ${it}" }
is then called on each item. The it
variable references the item in the list being processed.
##Spock Framework
The Spock framework is a popular testing framework for Groovy and is used to write concise and maintainable tests. Here is an example of a unit test written with the Spock framework:
class MathTest extends Specification {
def "should add two numbers"() {
expect:
Math.add(2, 3) == 5
where:
Math | function
}
}
import spock.lang.*
class Math {
static int add(int x, int y) {
return x + y
}
}
In this example, the MathTest
class is a Spock specification and it contains a single test that tests the functionality of the add
method of the Math
class. This test will pass if the add
method returns the expected value of 5
.
Working with Collections
Groovy’s collection classes make it easy to work with lists and maps. Here is an example of iterating over a list of strings and printing each one to the console:
def words = ['hello', 'world', 'Welcome']
words.each { word ->
println word
}
This code will print each word in the list to the console.
Database Access
Using Groovy SQL (GSQL), developers can access databases and execute queries. Here is an example of connecting to a database and querying the users table:
def db = Sql.newInstance('jdbc:postgresql://localhost:5432/my_database')
db.eachRow('SELECT * FROM users') {
println "$it.name ($it.email)"
}
This code will connect to the database and print out each row from the users table.
Metaprogramming
Metaprogramming enables developers to dynamically modify classes and methods at runtime. Here is an example of dynamically adding a method to the String class:
String.metaClass.reverse = {
delegate.reverse()
}
println 'hello world'.reverse() // prints 'dlrow olleh'
This code adds a reverse()
method to the String class, which can then be used to reverse strings.
Writing HTML & JavaScript Code
Groovy makes it easy to write HTML and JavaScript code without needing to switch contexts. Here is an example of generating HTML using Groovy’s MarkupBuilder:
def builder = new groovy.xml.MarkupBuilder()
builder.html {
head {
title 'My Page Title'
}
body {
p 'Welcome to my page!'
}
}
println builder
This code will generate the following HTML output:
<html>
<head>
<title>My Page Title</title>
</head>
<body>
<p>Welcome to my page!</p>
</body>
</html>
Below are examples of simple Groovy scripts. The first script creates a new class called HelloWorld and prints out a message:
class HelloWorld {
static void main(String[] args) {
println "Hello World!"
}
}
The next script displays the sum of two numbers:
def number1 = 10
def number2 = 5
def sum = number1 + number2
println "The sum is $sum"
Finally, the following script iterates over a list of strings and prints them out:
def list = ["Hello", "World", "Groovy"]
list.each { println it }
Conclusion
As you can see, the Groovy library offers a lot of powerful features that make it easy to quickly create complex, dynamic applications. From dynamic typing and AST transformations to closures and metaprogramming, Groovy is a powerful language that enables developers to quickly and easily write powerful applications.
For more information on Groovy, please visit the official website at https://groovy-lang.org.