Fortran
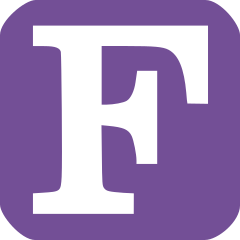
What is Fortran?
Fortran (short for “Formula Translating System”) is a general-purpose, procedural, imperative programming language that is especially suited to numeric computation and scientific computing. Developed by John Backus at IBM in 1957, Fortran is still widely used today in engineering, science and economics. Fortran features include structured programming, array programming, generic and object-oriented programming, and high-level data abstraction.
Fortran is the oldest programming language still in use for scientific, engineering and numerical computations. It is also one of the earliest languages to be compiled into machine code as opposed to being interpreted by a software program. As a result, Fortran is typically faster than languages such as BASIC or C/C++ when performing numerical computations.
History of Fortran
The original version of Fortran was released in 1957, making it the first widely used language for numerical computing. The language has grown and changed over the years, with Fortran 77 and Fortran 90 (the most commonly used versions) being released in 1978 and 1991, respectively. Since its initial release, Fortran has become the language of choice for many scientific, engineering and numerical computing applications. Its popularity was helped by the efficiency and clarity of its code, as well as its ability to perform complex calculations quickly. In addition to being used in a wide range of scientific and engineering fields, Fortran is also used in economics (e.g., economic modelling) and in some video games.
Origins and Early Years
Fortran was developed by John Backus at IBM in 1957. Backus had previously worked on the IBM 701, the first computer to use magnetic tape for storage, and the FORTRAN programming language would be the first high-level language to be used for scientific computing.
With FORTRAN, instead of having to write low-level assembly code, users could write programs using mathematical formulas that were then translated into assembly code. This made it much easier for scientists to write their own programs and allowed for more complex mathematics to be included in the programs.
Developments and Extensions
Over the years, Fortran has undergone many changes to keep up with the evolving needs of programmers. In 1966, the A version of Fortran was released, which added support for complex numbers and static data types, as well as some additional features. This version of Fortran, also known as “Forth” or “Standard Fortran,” went on to become the most widely used version of Fortran.
The next major milestone in the evolution of Fortran was the release of Fortran 77 in 1977, which introduced extended precision, the ability to pass functions as arguments, and other features that increased the expressiveness of the language.
In 1991, Fortran 90 was released, adding features such as dynamic memory allocation, object-oriented programming, and an array syntax based on linear algebra notation. Fortran then underwent several additional revisions over the next decade, culminating in the release of Fortran 2003 in 2004.
Current Usage
Today, Fortran is still widely used in scientific and numerical computing. It is particularly popular in fields such as nuclear physics and astronomy, due to its efficient execution of large calculations and its ability to handle very large datasets.
Recently, Fortran has been gaining interest from the computing industry for its ability to quickly process large amounts of data. In particular, Fortran is well suited for applications such as machine learning and deep learning, where large datasets need to be processed in a very short amount of time.
The language is also gaining traction as it becomes easier to use, with new libraries and tools that make it easier for developers to use the language. For example, Fortran can now be used with popular IDEs such as Visual Studio Code and Eclipse, making it easier for developers to use the language.
Features of Fortran
Fortran is a powerful language, offering advanced features for scientific and numerical computing. Some of the key features include:
- Complex number handling: numeric functions for real and complex numbers, subroutine for complex arithmetic operations, support for vector and matrix operations
- Dynamic memory allocation and automatic garbage collection
- User defined data types, allowing for the creation of structured data objects
- High performance matrix processing, with array syntax and intrinsic functions
- Parallelization capabilities through OpenMP and MPI
Adoption
Fortran has been used in a wide range of applications over the years. Some common examples include:
- Aerospace engineering: Fortran is often used for aerodynamic simulation codes and computational fluid dynamics programs.
- Financial analytics: Fortran is used to develop financial models for investments and portfolio management.
- Climate modeling: Fortran is used in global climate models to simulate and predict global weather patterns.
- Computational chemistry: Fortran is used to develop programs to simulate molecules, reactions, and materials.
- Image processing: Fortran is used to create programs for image analysis, such as facial recognition and medical imaging.
Code Examples
Below is an example of a simple program written in Fortran:
program hello print *, “Hello, World!” end program hello
This program simply prints the message “Hello, World!” to the screen. To make this program executable, the code must be compiled using a Fortran compiler.
Below is an example of a more complex program written in Fortran:
program complex implicit none real :: x = 0.0 do while (x <= 5) x = x + 0.1 print *, “x = “, x end do end program complex
This program calculates and prints out the value of x from 0.0 to 5.0 in increments of 0.1. This example demonstrates the use of looping constructs, as well as variables and functions in Fortran.
program hello_world
print *, "Hello World!"
end program
This is a simple program that prints “Hello World!” when executed.
program matrix_multiplication
implicit none
integer :: rows1, cols1, rows2, cols2
real :: a(rows1, cols1), b(rows2, cols2)
real :: c(rows1, cols2)
! Get dimensions of matrices
! Assume a and b are already populated
rows1 = size(a, 1)
cols1 = size(a, 2)
rows2 = size(b, 1)
cols2 = size(b, 2)
! Check if matrices are compatible
if (cols1 /= rows2) then
print *, 'Matrices not compatible.'
stop
else
! Compute matrix multiplication
do i = 1, rows1
do j = 1, cols2
c(i, j) = 0.0
do k = 1, cols1
c(i, j) = c(i, j) + a(i, k) * b(k, j)
end do
end do
end do
end if
end program
This program computes the matrix multiplication of two matrices, a
and b
, and stores the result in c
.
Here are some simple code examples to show how to get started with Fortran:
Printing a string:
program example
print *, 'Hello World!'
end program example
Declaring a variable and assigning it a value:
integer :: x
x = 5
Performing some mathematical operations :
a = 3.0
b = 2.0
c = a * b + 1
d = sqrt(c)
Creating a loop:
do i=1,10
print *, "i = ", i
end do
Defining a function:
real function square(x)
square = x**2
end function square
The following is a simple example of Fortran code:
program hello_world
print *, "Hello world!"
end program hello_world
This code simply prints “Hello world!” to the screen.
Conclusion
Fortran is a reliable and efficient programming language that is still in use today. It is suitable for both scientific and engineering applications, as well as a range of commercial applications. Despite its age, Fortran remains a popular language due to its speed and wide range of available tools.