Redis
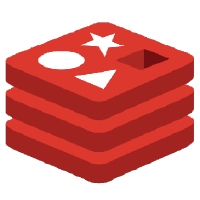
Redis
Redis is an open-source, in-memory data structure store that can be used as a distributed, NoSQL database. It is written in ANSI C and provides an in-memory key-value store as well as various data structures including lists, sets, sorted sets, strings, hashes and bitmaps. It features fast read/write operations, supports replication and clustering, offers automatic failover and master-slave synchronization, and can be used for application caching and session management.
History
Redis was first released in April 2009. It was created by Salvatore Sanfilippo, an Italian software engineer, who was inspired by the need to create an in-memory storage engine for web applications. The development of Redis was further inspired by Salvatore’s experience with creating memcached and researching other databases. Redis quickly gained popularity, becoming one of the most popular NoSQL databases in the world.
Redis History
Redis is a popular open-source in-memory data structure store used as a database, cache, and message broker. It was created by Salvatore Sanfilippo in 2009 and has since been adopted by many developers around the world. Redis supports a range of data structures such as strings, hashes, lists, sets, sorted sets with range queries, and bitmaps.
The Beginnings
When it was first created, Redis was simply an in-memory key-value store. It was designed with simplicity in mind and made use of dictionary-like data structures for its data. This allowed for rapid development and implementation of simple operations on data. The original goal of Redis was to provide a better alternative to traditional databases that had become too complex and slow for basic operations. This was achieved by making use of an in-memory key-value store system that operated without the need for a database.
Enhancements
Since its creation, Redis has evolved into a powerful data structure server. It now supports a range of data structures such as lists, strings, hashes, sets, and sorted sets. It also supports transactions, like ACID compliant transactions, and can be integrated with other applications using a library or API. In addition to its core features, Redis also supports master-slave replication and clustering. This allows for scale-out deployments, allowing you to shard data across multiple nodes for increased performance.
Use Cases
Redis is well-suited for a wide range of applications including real-time analytics, eCommerce, social networking, caching, distributed task queues, and more. It is well-known for its speed and low latency, making it a great choice for applications that require fast read and write operations. It is also able to store large amounts of data in memory, which can be beneficial for applications where data retrieval needs to be quick. Redis is also used in many popular web frameworks such as Ruby on Rails and Django, as well as in various cloud services such as Amazon Web Services, Google Cloud Platform, and Microsoft Azure.
Usages
Redis is often used as a database due to its scalability and high performance. It has been used in numerous applications, such as online games, finance, industrial automation, messaging systems, and more.
- Caching: Redis can be used as a caching layer for websites and services, allowing them to serve requests faster by storing commonly used data in memory. This makes it an excellent choice for speeding up applications.
- Message Queue: Redis can be used as a message queue for processing tasks asynchronously. This makes it a great choice for applications that need to handle large workloads.
- Data Storage: Redis can be used to store data such as user sessions, preferences, or any other type of data.
- Realtime Analytics: Redis can be used for realtime analytics, as it allows for near-instant access to data.
Code Examples
Connecting to Redis
The following example shows how to connect to Redis using the redis-py
library.
import redis
# Connect to Redis server
r = redis.Redis(host='localhost', port=6379, db=0)
# Check the connection
print(r.ping())
Setting and Getting Values
The following example shows how to set a key and then get the value associated with it.
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
# Set a key
r.set('name', 'John')
# Get the value of a key
print(r.get('name')) # John
Working with Lists
The following example shows how to work with lists in Redis.
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
# Create a list
r.rpush('fruits', 'apple')
r.rpush('fruits', 'banana')
r.rpush('fruits', 'orange')
# Get the length of the list
print(r.llen('fruits')) # 3
# Get all the values in the list
print(r.lrange('fruits', 0, -1)) # ['apple', 'banana', 'orange']
# Get the first item in the list
print(r.lindex('fruits', 0)) # apple
# Remove the first item in the list
print(r.lpop('fruits')) # apple
# Get the length of the list again
print(r.llen('fruits')) # 2
Working with Sets
The following example shows how to work with sets in Redis.
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
# Create a set
r.sadd('apples', 'red delicious')
r.sadd('apples', 'granny smith')
r.sadd('apples', 'fuji')
# Get all the values in the set
print(r.smembers('apples')) # {'red delicious', 'fuji', 'granny smith'}
# Check if a value is in the set
print(r.sismember('apples', 'red delicious')) # True
# Remove a value from the set
print(r.srem('apples', 'red delicious')) # True
# Get all the values in the set again
print(r.smembers('apples')) # {'fuji', 'granny smith'}
A basic example of a Redis command would be the GET command. This command is used to retrieve the value of a given key from a Redis database. For example:
127.0.0.1:6379> GET mykey
"myvalue"
This command retrieves the value of the key “mykey” from the Redis database and returns the value “myvalue”.
Another commonly used Redis command is the SET command. This command is used to set the value of a given key in a Redis database. For example:
127.0.0.1:6379> SET mykey "myvalue"
OK
This command sets the value of the key “mykey” to “myvalue”.
Redis can also be used to query data stored in sorted sets. For example, the following command will retrieve all elements of a given sorted set with scores between 0 and 1:
127.0.0.1:6379> ZRANGEBYSCORE myset 0 1
1) "element1"
2) "element2"
3) "element3"
This command returns the elements “element1”, “element2”, and “element3” with scores between 0 and 1 from the sorted set “myset”.
Setting and Getting
The following code example shows how to set and get a key:
// Set a key
redis> SET mykey "Hello Redis"
OK
// Get the value of the key
redis> GET mykey
"Hello Redis"
Setting an Expiration Time
The following code example shows how to set an expiration time for a key:
// Set the expiration time to 10 seconds
redis> EXPIRE mykey 10
(integer) 1
// Check if the key exists
redis> EXISTS mykey
(integer) 1
// Wait 10 seconds...
// Check if the key still exists
redis> EXISTS mykey
(integer) 0
Incrementing and Decrementing
The following code example shows how to increment and decrement the value of a key:
// Set the value of a key to 1
redis> SET count 1
OK
// Increment the value
redis> INCR count
(integer) 2
// Decrement the value
redis> DECR count
(integer) 1
Working with Sets
The following code example shows how to add, remove and retrieve elements from a set:
// Add elements to a set
redis> SADD myset "One"
(integer) 1
redis> SADD myset "Two"
(integer) 1
redis> SADD myset "Three"
(integer) 1
// Remove an element from the set
redis> SREM myset "Two"
(integer) 1
// Retrieve the elements in the set
redis> SMEMBERS myset
1) "One"
2) "Three"
Working with Sorted Sets
The following code example shows how to add, remove, and retrieve elements from a sorted set:
// Add elements to a sorted set
redis> ZADD myzset 1 "One"
(integer) 1
redis> ZADD myzset 2 "Two"
(integer) 1
redis> ZADD myzset 3 "Three"
(integer) 1
// Remove an element from the sorted set
redis> ZREM myzset "Two"
(integer) 1
// Retrieve the elements in the sorted set
redis> ZRANGE myzset 0 -1
1) "One"
2) "Three"
Working with Hashes
The following code example shows how to set, get, and delete fields from a hash:
// Set multiple fields with values in a hash
redis> HMSET myhash field1 "one" field2 "two" field3 "three"
OK
// Get the value of a field
redis> HGET myhash field2
"two"
// Delete a field from the hash
redis> HDEL myhash field2
(integer) 1
Connecting to Redis
To use Redis, you must first connect to a Redis server. The following example connects to a local instance of Redis using the Python client.
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
Setting a Key-Value Pair
Once connected to Redis, you can set a key-value pair using the set()
method.
r.set('name', 'John')
Retrieving a Value
You can retrieve a value from Redis using the get()
method.
name = r.get('name')
print(name) # 'John'
Storing Complex Data Types
Redis also supports storing complex data types such as lists, sets, and hashes. For example, the following code stores a list of numbers in Redis.
r.rpush('numbers', 1, 2, 3, 4, 5)
You can then retrieve the list with the lrange()
method.
numbers = r.lrange('numbers', 0, -1)
print(numbers) # [1, 2, 3, 4, 5]
Basic Commands
The following are some of the most commonly used commands in Redis:
-
SET
: Sets a key with a value. -
GET
: Retrieves a value from the key. -
DEL
: Deletes a key and its associated value. -
EXPIRE
: Sets a timeout on key expiration. -
EXISTS
: Checks if a key exists. -
INCR
: Increments the value of a key. -
DECR
: Decrements the value of a key. -
SADD
: Adds an element to a set. -
SMEMBERS
: Retrieves the members of a set. -
SREM
: Removes an element from a set. -
SINTER
: Calculates the intersection of two sets. -
ZADD
: Adds an element to a sorted set. -
ZRANGE
: Retrieves elements from a sorted set. -
ZREM
: Removes an element from a sorted set. -
HMSET
: Sets multiple fields with values in a hash. -
HGETALL
: Retrieves all fields and values from a hash.
Conclusion
Redis is an open source, in-memory data structure store first developed by Salvatore Sanfilippo in 2009. It is widely used as a database, cache, and message broker by organizations such as Twitter, GitHub, Craigslist, Instagram, and Amazon. Redis supports data structures such as strings, hashes, lists, sets, sorted sets with range queries, and bitmaps. It also has support for complex data types such as lists and hashes.