Visual Basic
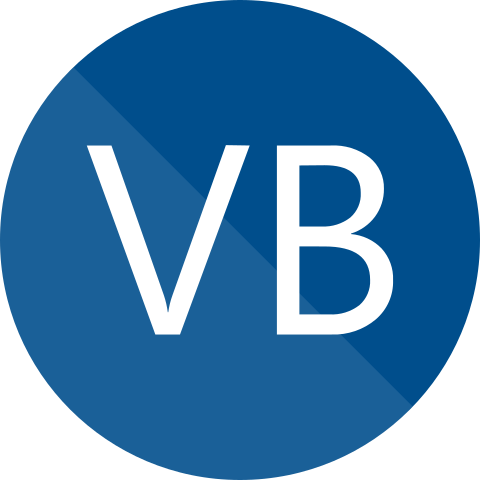
Visual Basic: A Comprehensive Overview
Visual Basic (VB) is a programming language that has been around since the early 1990s. It’s an event-driven language that is used for development of Windows GUI, web applications, and database systems. VB is considered to be one of the easiest programming languages to learn and master.
History of Visual Basic
The first version of Visual Basic was released by Microsoft in 1991, and was built to make writing programs easier than using assembly language or C. It introduced a graphical form designer as well as drag-and-drop functionality, making it much easier for beginners to learn the language and get started with software development. The next version of Visual Basic, version 4.0, was released in 1995 and included the ability to create 32-bit applications and support for component object model (COM). This version of Visual Basic also included Visual Basic Script, which is a scripting language used to automate tasks within Windows. In 1998, Microsoft released Visual Basic 6.0, which included support for ActiveX, Microsoft’s component object model, and improved tools for creating applications that could run on multiple platforms. Visual Basic .NET was released in 2002, and was a significant upgrade from the previous version. The .NET framework allowed for secure web services, better performance, and better scalability. It also included support for the .NET Common Language Runtime (CLR). The latest version of Visual Basic is Visual Basic 2015, which was released in 2015. This version of Visual Basic was designed to take advantage of the .NET framework and includes features such as LINQ, strongly typed data types, and asynchronous programming.
Adoption
Visual Basic is used for many different types of applications, from simple desktop applications to enterprise-level software. Here are some examples of how Visual Basic is commonly used:
Desktop Applications – Visual Basic can be used to create applications for Windows and other operating systems. Common examples include utilities, educational programs, and entertainment applications. Web Applications – Visual Basic can also be used to create dynamic web applications. This includes web-based databases, e-commerce stores, and online services. Mobile Applications – With the introduction of the Windows Mobile platform, Visual Basic can now be used to create mobile applications. These applications can be downloaded from the Windows Marketplace and used on smartphones and tablets. Games – Visual Basic can be used to create both 2D and 3D games. Some popular titles that have been created with Visual Basic include the original Age of Empires, Microsoft Flight Simulator, and World of Warcraft.
Benefits of Using Visual Basic
There are many benefits to using Visual Basic. One of the main benefits is that it is very easy to learn and use. It also has a large library of pre-built components, making it easy to create complex applications without having to code everything from scratch. The tooling that comes with VB is also very powerful and makes developing applications faster and easier. Another benefit is that Visual Basic is cross-platform compatible. This means that its applications can run on different operating systems, such as Windows and Mac OS X. This makes Visual Basic a great choice for creating applications that can be used on any platform. Visual Basic also has great debugging tools, making it easy to identify and fix bugs. It also has strong type checking, which helps to catch errors before they become problems.
Applications of Visual Basic
Visual Basic is used for a variety of applications, including web development, database systems, desktop applications, and graphical user interfaces. One of the most popular uses of Visual Basic is Windows GUI development. VB is ideal for creating applications that have a professional look and feel. It can also be used to develop games and other interactive applications. Visual Basic is also commonly used for database systems. It can be used to create database-driven applications and reports, as well as to interact with databases such as Oracle and SQL Server. Web applications are another popular use of Visual Basic. It can be used to create dynamic websites and web services. It’s often used for developing ASP.NET web applications, which are web applications written in the .NET framework. Finally, Visual Basic can be used to create graphical user interfaces. It has a powerful form designer that allows developers to quickly create interfaces for their applications.
Writing Code in Visual Basic
Visual Basic code is written in a text editor, such as Microsoft Visual Studio or Notepad++. It’s typically written in a language very similar to plain English, making it easy to read.
Here is an example of a simple program written in Visual Basic:
Public Class HelloWorld
Sub Main()
Console.WriteLine("Hello, World!")
End Sub
End Class
This code will print out “Hello, World!” in the console window when it is run.
Here is another example of a more complex program written in Visual Basic:
Public Class Calculator
Private Sub Calculate(ByVal num1 As Double, ByVal num2 As Double)
Dim result As Double = num1 + num2
Console.WriteLine(result)
End Sub
Shared Sub Main()
Dim calculator As New Calculator()
calculator.Calculate(4, 2)
End Sub
End Class
This code will create a basic calculator program that adds two numbers together and prints out the result.
Visual Basic makes it easy to create powerful applications with minimal effort. Below is an example of a basic “Hello World” program in Visual Basic:
Public Class MainForm
Private Sub MainForm_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
MessageBox.Show("Hello World!")
End Sub
End Class
This program displays a message box with “Hello World!” when the application is launched.
Working with Objects
In Visual Basic, everything is an object. This means that each object has its own set of methods and properties. To work with an object, you need to create an instance of the object and then access its properties and methods. For example, if you want to create a window on the screen, you could create an instance of the Form object
and then you could set its Text
property to change the text displayed on the window.
The following example creates a window on the screen with the text “Hello World”:
Dim myForm As New Form
myForm.Text = "Hello World"
myForm.Show()
You can also create custom objects in Visual Basic and add methods and properties to them. This makes it easy to create powerful applications that are tailored to the specific needs of the developer.
Working with Data
Visual Basic makes it easy to store and manipulate data, especially when using the integrated database capabilities. For example, you can create a database to store customers’ information and then use Visual Basic to query the database and display the results. This makes it easy to create applications that can access large amounts of data quickly and easily.
Creating GUI
Visual Basic includes a number of tools for creating graphical user interfaces (GUIs). These tools allow you to create professional-looking applications with ease. You can create forms, buttons, menus, and other controls and then customize their appearance and behavior to suit your needs.
Working with Libraries
Visual Basic includes a number of libraries that contain functions and classes that can be used to simplify common tasks. For example, the Windows API library contains functions for interacting with the Windows operating system and other software components. You can also include third-party libraries in your project to add additional functionality.
Debugging and Testing
Visual Basic includes a number of tools for debugging and testing your application. You can set breakpoints to pause the execution of your code and view the current state of the program. You can also use the integrated test runner to automatically execute tests and find bugs.
More examples
Here are some examples of Visual Basic code. The first example shows how to display a message box.
MsgBox "Hello, World!"
The second example shows how to create a simple GUI window.
Dim frmMain As New Form
frmMain.Text = "My Program"
frmMain.Show
The third example shows how to create a button and add it to a form.
Dim btnOK As New Button
btnOK.Text = "OK"
frmMain.Controls.Add(btnOK)
Finally, the fourth example shows how to connect to a database and retrieve data.
Dim conn As New ADODB.Connection
conn.Open "Provider=SQLOLEDB;Data Source=localhost;Initial Catalog=myDatabase;User Id=myUsername;Password=myPassword"
Dim rs As New ADODB.Recordset
rs.Open "SELECT * FROM Customers", conn
Do While Not rs.EOF
Debug.Print rs("Name")
rs.MoveNext
Loop
conn.Close
rs.Close
Conclusion
Visual Basic is a powerful programming language and development system for creating applications for Microsoft Windows. It has been around since 1991 and has been continually updated and improved since then. Visual Basic is mainly used to create GUI applications and data-driven applications, but it is also used to create custom ActiveX controls and objects. Furthermore, its syntax makes it easy to understand and work with. If you are looking for a language to develop Windows applications, Visual Basic is a great choice.