MongoDB
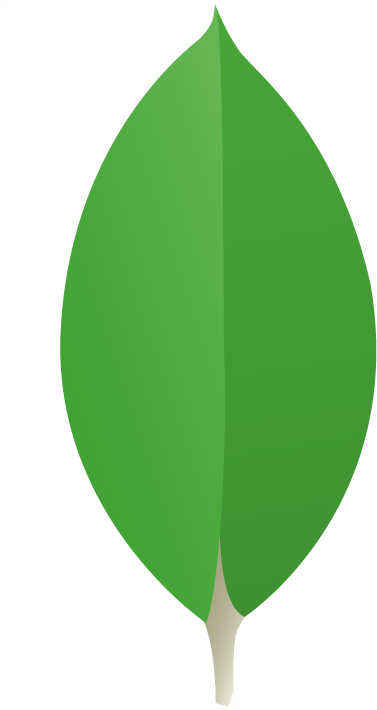
MongoDB
MongoDB is a cross-platform, open-source, non-relational database management system (DBMS) written in the C++ programming language. The most popular type of NoSQL database, MongoDB is designed for scalability and high availability. It allows for storing data using dynamic schemas, meaning documents within the same collection may store different sets of data. MongoDB is a popular choice among developers and data scientists due to its flexible and fast features.
History of MongoDB
MongoDB was developed by the company 10gen in 2007. Its first version, MongoDB 0.9.7, was released in 2009. In 2010, 10gen released an open source version of MongoDB and later in the same year launched a public cloud-based hosting service for the database. In October of 2012, 10gen officially changed their name to MongoDB Inc. Since then, MongoDB has been in active development and continues to be embraced by developers worldwide.
Origins of MongoDB
MongoDB was originally developed as a part of a web-scale platform at DoubleClick, the digital advertising company, where Merriman and Horowitz served as engineers. The project was meant to simplify their data processing jobs, and was soon expanded to include other use cases beyond ad-serving.
In 2007, Merriman and Horowitz founded 10gen, now MongoDB Inc., to bring MongoDB to the open-source world. 10gen launched MongoDB in 2009, and has since become the leading NoSQL database.
Adoption
MongoDB can be used for a variety of applications such as content management systems, web applications, real-time analytics, and big data. It is well-suited for use cases that require rapid development cycles, high scalability, and efficient query performance. Some popular uses of MongoDB include user authentication, geospatial queries, real-time analytics, recommendation systems, and full-text search.
Examples of how MongoDB can be used in a variety of applications include:
- User Authentication: MongoDB can be used to store and retrieve user authentication information such as usernames, passwords, and roles. This allows developers to create secure authentication systems quickly and easily.
- Geospatial Queries: MongoDB’s geospatial indexing feature makes it easy to query geographic data such as zip codes, cities, and states. This makes it useful for applications that require geographical data analysis.
- Real-Time Analytics: MongoDB’s fast read/write capabilities make it well-suited for applications that require real-time analytics. For example, it can be used to track user behavior in web and mobile applications.
- Recommendation Systems: MongoDB can be used to store and query user data to generate personalized recommendations. This can be useful for e-commerce applications that seek to increase customer engagement and sales.
- Full-Text Search: MongoDB’s text search feature allows users to quickly query text-based data such as blog posts, articles, and product descriptions. This can be used to power powerful search functions in applications.
Advantages of MongoDB
MongoDB has become very popular over the years due to its advantages. Here are some of the advantages of MongoDB:
- Scalability: MongoDB can scale horizontally and vertically when needed, meaning it can cope with large volumes of data and large numbers of users.
- Flexibility: MongoDB can store any type of data in any format, and the structure of the data can be changed on the fly.
- Performance: MongoDB is a very fast database that can handle millions of reads and writes per second.
- Cost: MongoDB is an open-source platform, so there are no license or usage fees associated with using it.
Disadvantages of MongoDB
Although MongoDB is a great solution for many projects, there are some disadvantages to consider as well. Here are some of the drawbacks of using MongoDB:
- Lack of Transactions: MongoDB does not support multi-document transactions, meaning it is not suitable for applications that require complex forms of data integrity.
- Limited SQL Support: MongoDB does not support the full range of SQL queries, meaning it cannot be used for applications that rely heavily on SQL.
- Complexity: MongoDB requires experienced developers to make the best use of its features, and its more complex syntax and data models can be difficult to understand.
MongoDB Features
MongoDB has many features that make it appealing to developers and businesses. The most prominent of these features include:
- Replication – MongoDB supports master-slave and master-master replication, allowing multiple copies of the same data to be stored across multiple servers for reliability and scalability.
- Sharding – MongoDB supports sharding, which allows a large dataset to be partitioned across multiple machines in order to improve performance and scalability.
- Indexing – MongoDB supports indexing, which allows users to quickly locate data within a collection.
- Aggregation – MongoDB supports aggregation functions, which allow users to quickly calculate results based on data stored in the database.
- Security – MongoDB supports and enforces user authentication, authorization, encryption, and access control.
Examples of MongoDB Usage
MongoDB is used in a variety of applications, ranging from small personal projects to large-scale enterprise platforms. Here are some examples of how MongoDB is used in the real world:
- Content Management Systems: MongoDB is used by some content management systems (CMS) such as WordPress, Drupal, and Joomla to store the content of websites.
- Social Networks: MongoDB is used by social networking sites such as Facebook and Twitter to store user profiles, posts, and comments.
- Analytics Platforms: MongoDB is used by analytics platforms such as Google Analytics and Adobe Analytics to store and analyze user data.
- eCommerce Platforms: MongoDB is used by ecommerce platforms such as Amazon and eBay to manage product data and store user information.
MongoDB Code Example
The following example shows how to connect to a MongoDB server and insert a document into a collection. This code is written in JavaScript and uses the MongoDB Node.js Driver.
// Connect to the MongoDB server
const MongoClient = require('mongodb').MongoClient;
const uri = "mongodb+srv://username:password@sample-cluster.mongodb.net/test?retryWrites=true&w=majority";
const client = new MongoClient(uri, {useNewUrlParser: true});
// Connect to the server
client.connect(err => {
// Create a collection
const collection = client.db("test").collection("books");
// Insert a document
collection.insertOne({
title: "Alice in Wonderland",
author: "Lewis Carroll"
}, (err, result) => {
if (err) {
console.log(err);
} else {
console.log("Inserted document into the books collection.");
}
// Close the connection
client.close();
});
});
This code connects to the MongoDB server, creates a collection called “books” and then inserts a new document into the collection.
Here is an example of how to use MongoDB in your code.
First, you need to create a MongoClient instance:
var mongoClient = new MongoClient(url);
Then, you need to connect to the MongoDB server using the connect() method:
mongoClient.connect(function(err) {
if (err) throw err;
console.log("Connected successfully to server");
});
Once connected, you can start working with a specific database. Let’s say we want to use the “database_name” database:
var db = mongoClient.db("database_name");
Next, we can start writing code to interact with the database. For example, we can insert a new document into a collection:
db.collection("collection_name").insertOne({name: "John Doe"}, function(err, res) {
if (err) throw err;
console.log("Document inserted");
});
This code creates a new document with the name “John Doe” in the “collection_name” collection.
We could also query the database for documents matching a certain criteria. For example, we could query the database for all documents with the name “John Doe”:
db.collection("collection_name").find({name: "John Doe"}).toArray(function(err, result) {
if (err) throw err;
console.log(result);
});
This code will return an array of all documents with the name “John Doe”.
These are just a few examples of how to use MongoDB in your code. There are endless possibilities for how you can use MongoDB in your applications.
Setting up a Database
To setup a MongoDB database, first install the MongoDB server. Once it is installed, create a “db” folder in the root directory of your project. This will be the directory where your database will be stored. To start the server, go to the command line and type the following command:
mongod --dbpath [path_to_db_folder]
Once the server is running, you can connect to it using the mongo shell. The shell is used to issue commands to the database, such as creating databases and collections, inserting data, updating data, and querying data.
Inserting Data
Now that the database is set up, we can insert data into it. To do so, we use the insert()
function, which takes an
object of values to be inserted into the collection. For example, if we had the following collection called users
:
{
"name": "John Doe",
"age": 30,
"address": "123 Main Street"
}
We can insert this data into the users collection using the following code:
db.users.insert({
"name": "John Doe",
"age": 30,
"address": "123 Main Street"
})
Once the data is inserted, it can be queried and updated using the find()
and update()
functions.
Querying Data
Once data is inserted into the database, it can be queried using the find()
function. The find()
function takes a
query object as an argument, which specifies what data should be returned. For example, if we want to find all users
whose age is greater than 25, we can use the following code:
db.users.find({
"age": {$gt: 25}
});
This query will return all documents that have an age value greater than 25.
Updating Data
Data in MongoDB can also be updated with the update()
function. This function takes a query object and an update
object as arguments, which specify the data to be updated. For example, if we want to update the address of the user we
inserted earlier, we could use the following code:
db.users.update({
"name": "John Doe"
}, {
$set: {
"address": "456 Pine Street"
}
});
This code will update the address of “John Doe” to “456 Pine Street”.
Deleting Data
Data can be deleted from the database using the remove()
function. This function takes a query object as an argument,
which specifies which documents should be deleted. For example, if we want to delete the user we inserted earlier:
db.users.remove({
"name": "John Doe"
});
This code will delete the document that contains the name “John Doe”.
Conclusion
MongoDB is a powerful tool that can be used to store, query, update, and delete data. With its ease of use and flexibility, it is a popular choice for many applications. MongoDB is a powerful and flexible database system that is well-suited for a variety of applications. It is designed for scalability and agility and allows developers to quickly store, query, and index data without the need for complex schemas. MongoDB can be used for user authentication, geospatial queries, real-time analytics, recommendation systems, and full-text search.