PHP
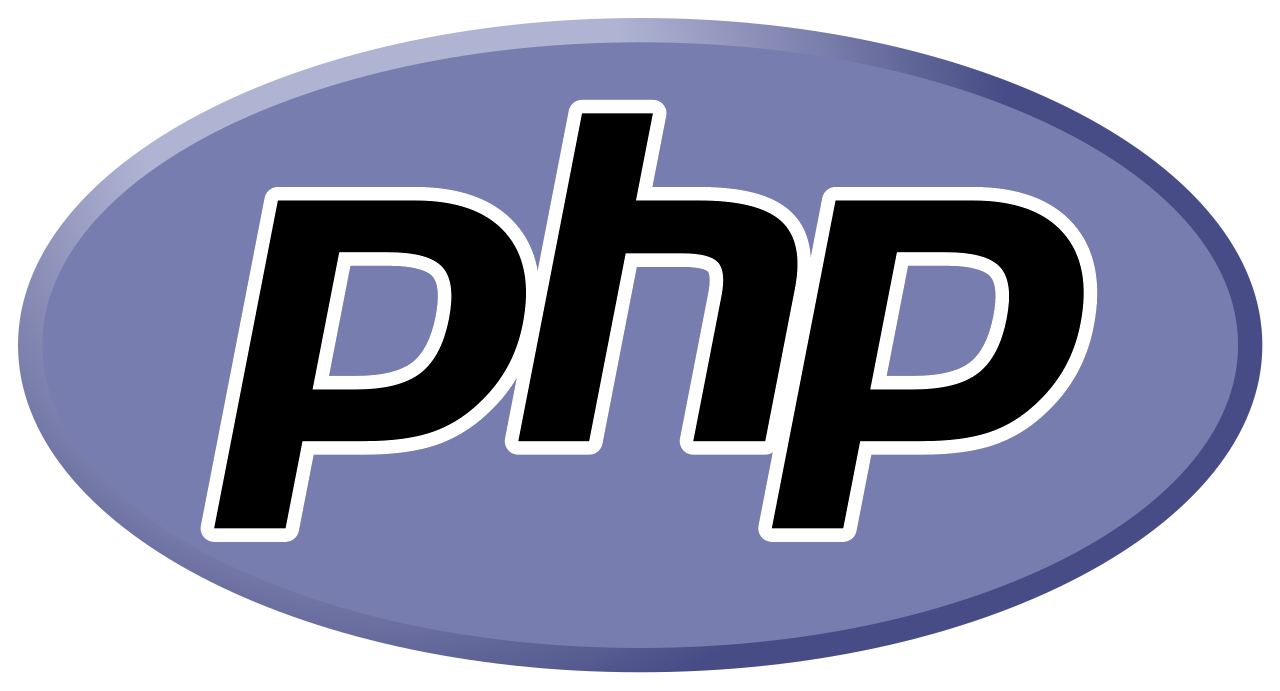
What is PHP
PHP is an open-source language, meaning that it can be freely used and modified without the need for a licence. This makes it an attractive choice for businesses who want to save money while still using a trusted and reliable language. PHP was created in 1995 by Rasmus Lerdorf and has grown to become one of the most popular server-side programming languages in the world. Today, it powers more than 80% of all websites on the internet, including some of the biggest players such as Facebook and Wikipedia.
History of PHP
PHP began life in 1995 as a set of Perl scripts written by Rasmus Lerdorf to track website visitors and manage his online resume. These scripts quickly gained popularity and were released to the public as “Personal Home Pages” or “PHP” . The language continued to grow in popularity and in 1997, Zeev Suraski and Andi Gutmans rewrote the scripting language from scratch, creating what would become known as the “Zend Engine”. This engine allowed for better memory management, improved performance, and support for many new features, making PHP a more powerful and efficient language. In the years since then, the language has continued to evolve, with the release of newer versions adding more powerful features and improved scalability. The latest version, PHP 8, was released in 2020 and includes improved performance, better error handling, and support for modern language features such as typed properties and attributes.
Examples of Usages and Code Examples
There are countless examples of how people are using PHP in their websites and applications. Here are a few of the most common examples:
- Dynamic Websites: PHP is perfect for building dynamic websites as it allows you to quickly create pages based on user input. PHP can be used to store and retrieve information from databases such as MySQL, as well as to perform calculations, generate forms and images, and much more.
- Content Management Systems: PHP is the language of choice for many popular content management systems (CMS) such as WordPress, Drupal, and Joomla. These CMSs allow users to easily create and manage their own websites without having to write code.
- Server-side Scripting: PHP can also be used to create server-side scripts that process data before sending it to the browser. This allows for greater control over user input and can make websites more secure and reliable.
- Command Line Scripting: PHP can also be used to create scripts that run from the command line. This allows for powerful automation tasks to be performed quickly and easily.
Here is an example of a basic PHP script that can be used to print out “Hello World”:
<?php
echo "Hello World";
?>
This code will output the text “Hello World” when run.
History of PHP
PHP is an open-source, server-side scripting language used primarily to create dynamic webpages. Created in 1994 by Rasmus Lerdorf, it has become one of the most widely used web development languages in the world. Originally called Personal Home Page, it was created to help Lerdorf manage his online resume and then gained popularity among web developers as a powerful yet simple way to make interactive web pages. PHP began its life as a set of Perl scripts written by Rasmus Lerdorf in 1994. In 1995, he released a more enhanced version of PHP, dubbed “Personal Home Page/Forms Interpreter.” This early version of PHP was primarily a set of Common Gateway Interface (CGI) programs and provided a basic set of functionality that enabled web developers to access the system environment variables and write HTML output for their web apps. In 1997, Zeev Suraski and Andi Gutmans took over the development of PHP and created a completely rewritten engine. This new engine, which was called the PHP/FI (for Forms Interpreter) 2.0, featured a much larger library of functions, syntax enhancements, and better code organization. In 1998, they released PHP 3.0, which was an almost entirely new product. They designed it with a more modular structure, allowing developers to easily modify, extend, and add new functions. The same year, the team released PHP/FI 2.01, which was a minor update to their 1997 product. Since then, there have been numerous iterations of PHP, with the current stable version being 7.2. PHP has also gained a wide range of third-party libraries that extend its functionality greatly.
Initial Development
When Lerdorf first developed PHP, it was used mainly as a set of tools to help with HTML forms and tracking website visitors. It included functions like form parsing, data gathering from database queries, and sending emails from forms. This language was then improved by Zeev Suraski and Andi Gutmans in 1997, and the new version was released as PHP 3. Later that year, PHP 4 was released with a new engine that allowed for the use of modules and better performance.
Refinement
Since its initial release, PHP has undergone several refinements. Each version came with better features, such as MySQL support and improved performance. PHP 5 was released in 2004, and included many improvements such as better object-oriented programming, better scalability and support for many more databases. Subsequent versions of PHP have made further improvements in the language, including faster execution, better memory management and improved support for modern web standards.
Popularity
As of June 2020, PHP is used by more than 78% of all websites, making it one of the most popular web development languages. It is used to power popular content management systems (such as WordPress and Drupal), e-commerce systems ( such as Magento and Zen Cart), and development frameworks (such as Laravel and Symfony).
Examples
Basic Syntax
The basic syntax of PHP is simple and easy to understand. The following is an example of how to print a string:
<?php
echo "Hello World!";
?>
This code will output the text “Hello World!” on the screen.
Variables
Variables are used to store data. They are denoted by a dollar sign ($). The following example shows how to use variables in PHP:
<?php
$name = "John";
echo "Hello, $name!";
?>
This code will output the text “Hello, John!” on the screen.
Functions
Functions are pieces of code that can be reused multiple times. The following example shows how to create and use a function in PHP:
<?php
function greet($name) {
echo "Hello, $name!";
}
greet("John");
?>
This code will output the text “Hello, John!” on the screen.
Classes
Classes are used to organize related functions and variables into a single unit. The following example shows how to create and use a class in PHP:
<?php
class Person {
public $name;
function greet() {
echo "Hello, $this->name!";
}
}
$person = new Person();
$person->name = "John";
$person->greet();
?>
This code will output the text “Hello, John!” on the screen.
Arrays
Arrays are used to store collections of data. The following example shows how to create and use an array in PHP:
<?php
$names = array("John", "Mary", "Jane");
foreach($names as $name) {
echo "Hello, $name!";
}
?>
This code will output the text “Hello, John! Hello, Mary! Hello, Jane!” on the screen.
Developing Websites with Database Access
PHP can be used to create websites with powerful database-driven features, such as user authentication, database queries and database updates. Furthermore, PHP can be used to create shopping carts and provide a secure environment for users to pay for products online.
Creating Web Services
PHP can be used to create web services that can be used by other applications to interact with a website. For example, a web service could allow users to search for specific information within a website’s database.
File Uploads
PHP can be used to handle file uploads from web browsers. This allows users to upload files such as images, documents and other files to a website.
Generating Dynamic Images
PHP can be used to generate dynamic images on the fly. This can be used to create images that change based on user input or other dynamic data.
Creating Complex Graphics
PHP can be used to generate complex graphics, such as charts and diagrams. This is useful for displaying data or presenting information in a visually appealing manner.
Code Examples
Here are some code examples of how to use PHP:
Database Access
This example shows how to connect to a MySQL database and perform a query:
$db = mysqli_connect("localhost", "user", "password");
if (!$db) {
die('Could not connect: ' . mysqli_error());
}
$query = "SELECT * FROM table";
$result = mysqli_query($db, $query);
while ($row = mysqli_fetch_array($result)) {
echo $row['field'];
}
Generating a Dynamic Image
This example shows how to generate a dynamic image with PHP:
<?php
$image = imagecreatetruecolor(500,500);
$white = imagecolorallocate($image, 255, 255, 255);
$blue = imagecolorallocate($image, 0, 0, 255);
imagestring($image, 5, 50, 50, "Hello World!", $blue);
header("Content-type: image/png");
imagepng($image);
imagedestroy($image);
?>
File Uploads
This example shows how to handle file uploads with PHP:
<?php
if (isset($_FILES['uploadedfile'])) {
$target_path = "uploads/";
$target_path = $target_path . basename($_FILES['uploadedfile']['name']);
if (move_uploaded_file($_FILES['uploadedfile']['tmp_name'], $target_path)) {
echo "The file " . basename($_FILES['uploadedfile']['name']) .
" has been uploaded";
} else {
echo "There was an error uploading the file, please try again!";
}
}
?>
PHP is widely used for creating dynamic websites and web applications. It can do anything from process HTML forms, build entire e-commerce systems, provide RSS feeds, generate dynamic PDF files, and interact with databases like MySQL.
Form Processing
Form processing is one of the most common uses of PHP. It enables web developers to quickly process and validate input from a user. For example, let’s say we have an HTML form with a field that takes in a username. We could use the following PHP code to validate the input and make sure it is unique.
<?php
// Get form data
$username = $_POST['username'];
// Check if username already exists in database
// (assume database connection and query have already been established)
$result = mysql_query("SELECT * FROM users WHERE username = '$username'");
if (mysql_num_rows($result) > 0) {
echo "Username already exists!";
} else {
// Process form data
}
?>
Database Interaction
PHP provides a variety of functions and extensions for interacting with databases, such as MySQL and Oracle. Here is an example of some code that can be used to retrieve a list of records from a database table.
<?php
// Establish connection to the database
$db = mysql_connect("hostname","username","password");
mysql_select_db("database_name",$db);
// Run a query to get all records from "products" table
$result = mysql_query("SELECT * FROM products");
// Loop through results and print out a list
while ($row = mysql_fetch_array($result)) {
echo "<li>" . $row['name'] . "</li>";
}
?>
E-Commerce Systems
PHP is also heavily used to build entire e-commerce systems. It provides the power and flexibility to handle customer data, manage inventory and orders, integrate payment gateways, and much more. Here is a simple example of a function that can be used to calculate the total cost for an order:
<?php
// Calculate the total cost of an order
function getTotal($products) {
$total = 0;
foreach ($products as $product) {
$total += $product['price'] * $product['quantity'];
}
return $total;
}
?>
Conclusion
PHP is a versatile, open-source scripting language that can be used to create dynamic websites and web applications quickly and efficiently. It has grown tremendously over the years and now boasts a huge library of third-party packages and libraries to extend its functionality. With its robust capabilities, simplicity and popularity, PHP is likely to remain a mainstay of the web development world for years to come.