iOS
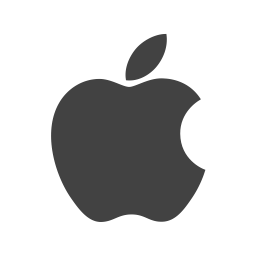
iOS
“iOS” is an operating system created and developed by Apple Inc. It is used primarily on devices such as Apple’s iPhone, iPod Touch and iPad. It is the second most popular mobile operating system after Android.
History
The initial version of iOS was announced in January 2007, with the release of the original iPhone. Since then, it has undergone numerous updates and upgrades, with the latest being iOS 14. iOS was primarily designed for touchscreen user interface devices. The iPhone was first unveiled by Steve Jobs at the Macworld conference on January 9, 2007. Initial development of the iPhone OS (as it was then known) began in 2005, and was based on the core of the Mac OS X operating system. The original version of iOS included features such as multi-touch gestures, an email client, a web browser, an address book, a media player, support for third-party applications, and more. With the release of the iPhone 3G in 2008, Apple also released the App Store, which allowed users to download and install third-party applications from the iTunes Store. Since then, the App Store has grown to become one of the largest mobile application stores in the world, with over two million apps available. Since its introduction, iOS has undergone several major updates, including the introduction of support for new devices, such as the iPad, Apple Watch, and Apple TV, as well as improvements in the user interface and performance. In 2010, iOS 4 was released, introducing multitasking, and the Notification Center. iOS 5 introduced iCloud, and Siri in 2011. iOS 6 introduced Apple Maps, and Passbook in 2012. iOS 7 saw the redesign of the user interface, and the introduction of Control Center and AirDrop. iOS 8 introduced Health, Apple Pay, and the ability to use third-party messaging services. iOS 9 introduced a revamped Notes app, Proactive, and split-screen multitasking.
Features
iOS has many features that make it an attractive platform. One of these features is its intuitive interface and user-friendly design. It is also capable of multitasking, which makes it efficient for usage. Additionally, it offers powerful applications such as the App Store, where users can download third-party apps and games. iOS is secure and has integrated hardware encryption and other security measures.
iOS Development
iOS is an open platform, meaning any developer can create and distribute apps for the platform. There are several ways to develop apps for iOS, including using the native iOS SDK, using a cross-platform development framework, or using an HTML5-based web app. The iOS SDK is the official development tool provided by Apple to create apps for the platform. The SDK includes all of the tools and needed to create apps, as well as a simulator so developers can test their apps without having to deploy them to an actual device. Cross-platform frameworks make it possible to develop apps that will run on multiple platforms, including iOS. These frameworks provide an abstract layer that makes it easier to develop apps, as well as tools for deploying and debugging apps. Popular frameworks include React Native, Flutter, and Ionic. Finally, web apps can be developed using HTML5 and JavaScript. This is a great way to create apps that are optimized for mobile devices, or to create cross-platform apps that can run on many different platforms.
iOS SDK
The iOS SDK (Software Development Kit) is a set of tools used to develop native apps for the iOS platform. It provides , header files and sample code for developers. It also includes a graphical user interface (GUI) to help developers design and implement their apps.
RoboVM
RoboVM is an open-source toolchain for the iOS platform. It allows developers to write in Java and compile it into an iOS executable. It supports a wide range of and frameworks and has excellent performance.
Google Guice
Google Guice is an open-source for the iOS platform, allowing developers to write dependency-injection code in Java. It simplifies the development process by removing the need to write complex boilerplate code.
Apache Cordova
Cordova is an open-source mobile application framework used to create native apps using HTML, CSS and JavaScript. It is based on the popular Apache Cordova technology and supports various features such as device access, storage, media and more.
Swift Programmers
Swift Programmers is a for developing iOS apps in Swift, Apple’s modern programming language. It includes functionality for design, testing and debugging. It also contains extensions for making it easier to create custom UI elements.
iOS apps are written using the Objective-C programming language. This code example illustrates how to create a simple iOS app:
#import <UIKit/UIKit.h>
@interface AppDelegate : UIResponder <UIApplicationDelegate>
@property (strong, nonatomic) UIWindow *window;
@end
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Override point for customization after application launch.
return YES;
}
@end
This basic app will display a blank window when launched. To add content to the window, you can add code to the didFinishLaunchingWithOptions
method.
// Create a view controller
UIViewController *viewController = [[UIViewController alloc] init];
// Assign it as the window's root view controller
self.window.rootViewController = viewController;
// Add a label to the view
UILabel *lbl = [[UILabel alloc] initWithFrame:CGRectMake(100, 100, 200, 30)];
[lbl setText:@"Hello World!"];
[viewController.view addSubview:lbl];
This code will create a view controller, assign it to the window’s root view controller, and then add a label with the text “Hello World!” to the view.
AFNetworking
AFNetworking is a popular iOS used for making network requests. It is designed to simplify the task of working with requests and responses, and it handles common tasks such as caching and progress monitoring.
The following example shows how to use AFNetworking to make a GET request:
NSURL *URL = [NSURL URLWithString:@"https://example.com/api/v1/endpoint"];
NSURLSessionConfiguration *config = [NSURLSessionConfiguration defaultSessionConfiguration];
AFURLSessionManager *manager = [[AFURLSessionManager alloc] initWithSessionConfiguration:config];
NSURLRequest *request = [NSURLRequest requestWithURL:URL];
NSURLSessionDataTask *dataTask = [manager dataTaskWithRequest:request completionHandler:^(NSURLResponse *response, id responseObject, NSError *error) {
if (error) {
NSLog(@"Error: %@", error);
} else {
NSLog(@"%@ %@", response, responseObject);
}
}];
[dataTask resume];
Masonry
Masonry is another popular used for creating auto-layout constraints. It is designed to be a simpler and faster alternative to writing autolayout code manually. The following example shows how to use Masonry to create a constraint between two views:
NSArray *views = @[view1, view2];
[views mas_makeConstraints:^(MASConstraintMaker *make) {
make.equalTo(otherView).with.offset(10);
make.left.equalTo(self.view).offset(20);
make.size.mas_equalTo(CGSizeMake(100, 100));
}];
KeychainSwift
KeychainSwift is a used to securely store data such as user passwords and authentication tokens. It is designed to be secure by default and can help developers protect sensitive information.
The following example shows how to use this to save a password:
KeychainSwift.standard.set("password", forKey: "passwordKey")
Here is a simple example of an iOS app written in Swift:
import UIKit
class MyViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
}
And here is an example of an iOS app written with React Native:
import React, { Component } from 'react';
import { View, Text } from 'react-native';
class MyApp extends Component {
render() {
return (
<View>
<Text>Hello World!</Text>
</View>
);
}
}
Conclusion
iOS is a powerful and popular mobile operating system that powers millions of devices around the world. It has an intuitive user interface, numerous security features, and is open to developers, allowing them to create their own apps and games for the platform. With its wide range of features and support for multiple languages, iOS is a great choice for anyone looking for a powerful and secure mobile platform.