C
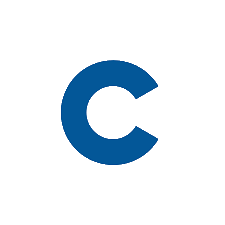
C Language
C language is one of the most popular and widely used programming languages of all time. It was created by Dennis Ritchie in 1972 at AT&T Bell Labs.
The language combines the features of high-level programming languages such as C++ and Java, but retains the low-level abilities associated with assembly code. This makes it ideal for systems programming tasks, such as creating operating systems, device drivers, and embedded applications.
History
C was initially designed by Dennis Ritchie as a systems programming language for the Unix operating system. In 1978, Brian Kernighan and Dennis Ritchie published the first edition of the “C Programming Language”, which became the definitive reference for the language. Since then, it has been revised several times and is still widely used today.
The initial version of C was developed by Dennis Ritchie at AT&T Bell Labs as a general-purpose language. The first version was based on an earlier language called “B,” which had been developed at Bell Labs around 1970.
Over time, the language evolved to become more powerful and robust, with the addition of features such as the ability to pass arguments to functions and support for structures and unions. A standard for the language, known as the ANSI C standard, was completed in 1989.
Since then, the language has been extended with object-oriented capabilities and additional libraries. The most popular version of C today is the C99 standard, which was released in 1999.
Features
C is a powerful and versatile language that is simple and efficient. It is highly portable and platform-independent, making it ideal for cross-platform development. It is also an incredibly powerful language with powerful features such as pointers and dynamic memory allocation, allowing developers to make very efficient programs.
Usage
C is one of the most popular programming languages and is used in a variety of applications. It is often used in embedded systems, operating systems, compilers, interpreters, text editors and web servers. It is also used to create graphical user interface (GUI) applications, games, device drivers and other software.
Syntax
C is known for its terse and precise syntax, making it easy to read and understand. A basic C program consists of functions, variables, control structures, preprocessor directives and comments. Here is an example of a simple “Hello World” program written in C:
#include <stdio.h>
int main()
{
printf("Hello, World!");
return 0;
}
Types
C supports a variety of data types, including primitive types such as int, float, char, bool and more complex types such as struct, union and enum.
Libraries
C has a rich standard library, providing a number of useful functions and macros. Some of the most popular libraries include the Standard C Library, the GNU C Library and the Microsoft C/C++ Run-Time Library.
Compilation
In order to compile and execute a C program, it must be compiled first. This is done using an external compiler such as gcc or clang. Once the program is compiled successfully, it can be executed from the command line.
Syntax and Structure of C
The syntax of C is fairly simple and consists of a set of basic keywords such as if
, for
, and while
, as well as
specific built-in operators.
Structures in C are composed of collections of individual variables, which can be either primitive data types such
as int
or char
, or user-defined types such as structures or unions.
C also supports functions, which are blocks of code that can accept input and return output. The output from a function can be used as the input to another function, allowing for complex operations.
Examples of C Code
Hello World
This is a simple example of the classic hello world program in C:
#include <stdio.h>
int main() {
printf("Hello, world!\n");
return 0;
}
The #include
directive is used to include a library file, in this case the standard input/output library which
provides the printf
function.
The int main()
line defines a function named main and declares it to return an integer value. The body of the program
is contained within the curly braces.
The printf
function prints the string passed to it on the screen. The \n
character indicates a line break.
Finally, the return 0
statement tells the program to return the value 0 to the operating system, indicating that the
program has executed successfully.
Data Types
This program uses basic C data types to declare and initialize variables:
#include <stdio.h>
int main() {
int i = 10;
char c = 'a';
float f = 3.14;
printf("i = %d, c = %c, f = %f\n", i, c, f);
return 0;
}
The int
data type is used to declare the integer variable i
, char
is used for the character variable c
,
and float
is used for the floating point number f
.
The printf
function is used to print the values of the variables on the screen. The %d
indicates that an integer
should be printed, %c
indicates that a character should be printed, and %f
indicates that a floating point number
should be printed.
Conclusion
C is an incredibly powerful and versatile language that has been used in countless applications over the last several decades. Its simple syntax and robust libraries make it a favorite of programmers around the world.
C is an immensely popular and powerful language that is used in many different applications. Its concise syntax makes it easy to read and understand, while its powerful features and libraries make it a great choice for many types of projects. If you are looking for a language that is fast, efficient and flexible, then C is the perfect choice.