MySQL
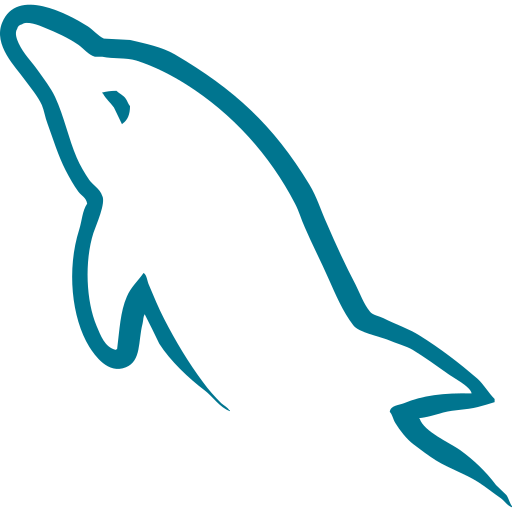
Introduction
MySQL is a powerful and popular open source relational database management system. It is the world’s most widely used open source relational database and its popularity continues to grow. MySQL is used in many industries and has a wide range of applications. It is used by businesses to store and manage their data, by website developers to create fast, reliable websites, and by application developers to develop applications.
MySQL is an attractive choice for web developers because it is easy to use and provides strong data protection and high reliability. It supports multiple storage engines, which allow users to choose the engine that best suits their needs. It also features a variety of options for configuring the database and its performance.
The history of MySQL goes back to the mid-1990s. It was originally developed by Michael Widenius, who founded a company called MySQL AB to develop and support it. When the company was sold to Sun Microsystems in 2008, it became part of the Oracle Corporation. Oracle maintained the support and development of MySQL until January 2019 when Oracle decided to spin off the MySQL business unit into an independent company called MySQL AB, which is now owned by Oracle.
MySQL is used by many large businesses, such as Twitter, Facebook, Google, Etsy, and Yahoo, as well as smaller companies. Additionally, it is used by a number of open source projects, such as WordPress, Joomla, and Drupal.
MySQL is written in C++ and is licensed under the GNU General Public License (GPL). This means that anyone can use and modify the software for free.
MySQL supports numerous storage engines, which provide different functions and capabilities. Some of the more popular storage engines are MyISAM, InnoDB, Memory, and Archive. The storage engine used depends on the specific requirements of each project.
For example, the MyISAM storage engine provides efficient reads and writes from single tables. The InnoDB storage engine provides transactional processing and sophisticated locking capabilities, which make it well suited for mission-critical applications. The Memory storage engine provides quick access to unstructured data and the Archive storage engine provides efficient storage for rarely accessed data.
MySQL also provides a host of tools to help developers manage their database applications, including database administration tools, SQL language tools, and database replication tools. These tools allow developers to easily configure, monitor, and tune their database environment.
In addition to these tools, MySQL also provides access to a number of programming APIs. These APIs allow developers to extend MySQL with custom applications and access data using popular scripting languages such as PHP and Python.
MySQL is highly extensible, allowing developers to customize the database to their exact needs. Developers can add custom functions and stored procedures, as well as create triggers and views.
MySQL also provides a number of performance tuning options, such as memory and query caching, as well as thread pooling. This allows developers to optimize their database environment for maximum performance.
MySQL is an attractive database due to its scalability, ease of use, and robust feature set. It is a popular choice for web development, as well as for applications that require a high degree of performance and reliability. By leveraging its features and tools, developers can create powerful database applications quickly and reliably.
History
The first version of the software was released in 1995. MySQL was originally developed by Swedish developer Michael Widenius and David Axmark in 1995 and further developed by Oracle since 2010.
Features
- Open source software released under the GNU General Public License (GPL)
- Supports multiple storage engines
- Offers full support for transactions
- Has referential integrity constraints
- Supports stored procedures
- Supports replication
- Offers several different replication methods
- Supports views, triggers and cursors
- Create privileges for users
- Support for embedded databases and web-based applications
- Support for programming languages such as Java, Perl, PHP, Python, Ruby and more
Usages
MySQL is a widely used database in many web applications and websites. It is also used in content management systems such as WordPress, Drupal, Joomla, Unbounce and more. Additionally, MySQL can also be used in data science projects. It can be used to store, query and analyze large datasets effectively.
MySQL is also commonly used in e-commerce platforms such as Magento and Shopify. It can be used to store data related to customers, products, orders, payments and even inventory. MySQL can also be used in game development platform such as Unity and Unreal Engine.
Code Examples
Below are code examples from a few programming languages using MySQL:
Java
import java.sql.Connection;
import java.sql.DriverManager;
public class DatabaseConnect {
public static void main(String[] args) {
//Create a variable for the connection string
String connectionUrl = "jdbc:mysql://localhost:3306/mydatabase";
//Declare the JDBC objects
Connection con = null;
try {
// Establish the connection
Class.forName("com.mysql.jdbc.Driver");
con = DriverManager.getConnection(connectionUrl, "username", "password");
} catch (Exception e) {
e.printStackTrace();
}
}
}
PHP
<?php
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "mydatabase";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Close the connection
$conn->close();
?>
Python
import MySQLdb
# Open database connection
db = MySQLdb.connect("localhost","username","password","mydatabase" )
# prepare a cursor object using cursor() method
cursor = db.cursor()
# execute SQL query using execute() method.
cursor.execute("SELECT VERSION()")
# Fetch a single row using fetchone() method.
data = cursor.fetchone()
# close the database connection
db.close()
MySQL Library
MySQL is an open-source, relational database management system used by multiple applications. It is primarily used for storing and managing structured data, but its feature set also supports unstructured data, such as documents and media. MySQL is also widely used in web development, as it supports popular web programming languages like PHP and JavaScript.
History of MySQL
MySQL was developed in 1995 by two computer scientists, Michael Widenius and David Axmark, as a fork of the original Unix-based MySQL code. In 2008, Sun Microsystems acquired MySQL AB and later Oracle acquired Sun Microsystems in 2010, becoming the primary owner of MySQL. Since then, Oracle has continued to develop and improve MySQL.
Features of MySQL
MySQL is a popular choice for web development because of its many features and advantages. Here are some of the features that make MySQL so attractive to developers:
-
SQL-based: MySQL uses a SQL-based syntax to control data, making it easy for developers to write complex queries.
-
Robustness: MySQL is extremely reliable and robust, making it suitable for mission-critical applications.
-
Security: MySQL has a built-in security model to protect sensitive data, and provides users with the ability to manage access privileges.
-
Flexibility: MySQL can be set up on many different operating systems and platforms, making it a flexible choice for development.
-
Open-source: MySQL is an open-source system, meaning it is free to use. This makes it an ideal choice for small businesses and hobby projects.
Examples of MySQL Usage
MySQL is used in many application areas, from web applications and data warehousing to online commerce and embedded systems. Here are some of the most common uses of MySQL:
-
Web Applications: MySQL is used to provide persistent storage for dynamic web applications. MySQL stores user data and content, and enables users to interact with websites.
-
Data Warehousing: MySQL is used to store large amounts of data in a structured format, making it easy to query and analyze.
-
Online Retail: MySQL is used to store product information, customer profiles, order information, and other data associated with online stores.
-
Social Networks: MySQL stores user profiles, friend connections, messages, and other social network data.
-
Embedded Systems: MySQL is used in embedded devices, from medical equipment and vehicles to home appliances.
Code Examples
Connecting to a MySQL Database
The first step in using MySQL is to connect to a database. To do this, you must provide a valid username and password. Here’s an example of how to connect to a MySQL database using Java:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DBUtil {
private static final String DB_URL = "jdbc:mysql://localhost/mydatabase?useSSL=true";
private static final String USER = "username";
private static final String PASSWORD = "password";
public static Connection connect() throws SQLException {
return DriverManager.getConnection(DB_URL, USER, PASSWORD);
}
}
Querying a MySQL Database
Once the connection is established, the next step is to execute a query against the database. Here’s an example of how to execute a SELECT query to retrieve data from a MySQL database using Java:
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class QueryUtil {
public static ResultSet query(Connection conn, String sql) throws SQLException {
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(sql);
return rs;
}
}
Inserting Data into a MySQL Database
Once the connection is established, the next step is to execute an INSERT query to add data to a MySQL database using Java:
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.Statement;
public class InsertUtil {
public static void insert(Connection conn, String sql) throws SQLException {
Statement stmt = conn.createStatement();
stmt.executeUpdate(sql);
}
}
Updating Data in a MySQL Database
Once the connection is established, the next step is to execute an UPDATE query to update data in a MySQL database using Java:
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.Statement;
public class UpdateUtil {
public static void update(Connection conn, String sql) throws SQLException {
Statement stmt = conn.createStatement();
stmt.executeUpdate(sql);
}
}
Deleting Data from a MySQL Database
Once the connection is established, the next step is to execute a DELETE query to remove data from a MySQL database using Java:
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.Statement;
public class DeleteUtil {
public static void delete(Connection conn, String sql) throws SQLException {
Statement stmt = conn.createStatement();
stmt.executeUpdate(sql);
}
}
MySQL
MySQL is an open-source, relational database management system (RDBMS) based on Structured Query Language (SQL). The world’s most popular open source database, MySQL has been around since 1995 and is the go-to choice for many organizations seeking a dependable and robust database solution. It is probably best known for its speed and reliability, but it also offers features like scalability and data integrity that ensure it remains a powerful choice for web development and business applications alike.
History
MySQL was created in Sweden by a man named Michael Widenius – the lead architect of the original project. Widenius developed the project while working at a Swedish IT company called TMC, and soon afterwards released it as an open source project. Since then, MySQL has become one of the most popular databases in the world, with millions of installations across hundreds of thousands of domains.
In 2008, MySQL was acquired by Sun Microsystems, which was subsequently acquired by Oracle Corporation in 2010. This acquisition solidified MySQL’s foothold in the enterprise market, and Oracle has continued to expand and improve upon the software’s capabilities and features. Today, MySQL is available in several versions, from the free and open-source community edition, to the highly scalable and secure Enterprise Edition.
Features
MySQL is renowned for its speed, flexibility and reliability. It is designed to be easy to use and create powerful applications quickly, which makes it a popular choice for web developers. It supports a wide array of platforms, including Linux, Windows, Mac OS X, Solaris, FreeBSD and many other operating systems.
It supports a variety of programming languages, including PHP, Java, C++, Python and Ruby, and can be used with a range of other technologies, such as Apache, Joomla and WordPress. It also supports replication, allowing you to have multiple servers that are automatically synchronized with each other. This ensures your data stays consistent, no matter where it is stored or accessed.
MySQL also offers numerous data storage engines to choose from. These engines provide different levels of customization and performance, allowing you to select the best engine for your particular application or use case. These engines include the InnoDB engine, which is the default engine, and provides excellent transaction support; the MyISAM engine, which is optimized for high-speed read operations; and the Memory engine, which allows for faster operations when storing temporary data.
Finally, MySQL provides comprehensive data security features, such as authentication and authorization. Authentication allows you to control access to the database, while authorization allows you to control what actions users can take. This means that you can easily ensure that only authorized personnel can access sensitive data.
Uses
MySQL is widely used in web development, enterprise applications, data warehousing and analytics. It is used by some of the world’s largest companies, including Google, Facebook, YouTube, Twitter and LinkedIn, and can be found in a wide range of applications, from e-commerce websites and content management systems to mobile games and social networks.
It is also used for data analysis and data warehousing, and can facilitate transactions between different databases and applications. This makes it an ideal choice for businesses who need to store, process and analyze large amounts of data quickly and efficiently.
Example
Here is a simple example of how to connect to a MySQL database in Java:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DBConnection {
public static void main(String[] args) {
Connection con = null;
try {
// Load the MySQL driver
Class.forName("com.mysql.jdbc.Driver");
con = DriverManager.getConnection("jdbc:mysql://localhost/test", "username", "password");
System.out.println("Successfully connected to the MySQL database!");
} catch (ClassNotFoundException ex) {
System.out.println("Could not find the MySQL driver class.");
} catch (SQLException ex) {
System.out.println("An error occurred while connecting to the database.");
} finally {
if (con != null) {
try {
con.close();
} catch (SQLException ex) {
System.out.println("An error occurred while closing the connection.");
}
}
}
}
}
In this example, we use the Class.forName()
method to load the MySQL driver, and then use
the DriverManager.getConnection()
method to establish a connection to the database. Once the connection is
established, we can use standard SQL commands to query and update the database.
Conclusion
MySQL is one of the world’s most popular and powerful relational database management systems. It is renowned for its speed and reliability, but it also provides features like scalability and data integrity that make it suitable for a wide range of applications. With its robust set of features, intuitive APIs and wide range of supported platforms, MySQL is an excellent choice for web development and enterprise applications alike.