Swift release cycle
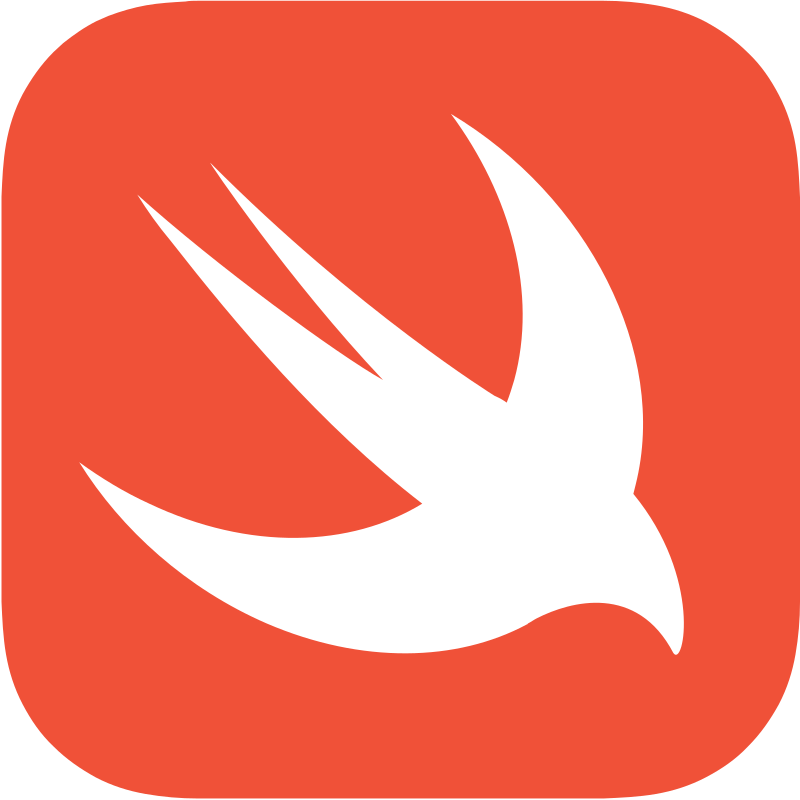
Swift Language Release Cycle
The Swift release cycle is the process of releasing new versions of Swift. Each version brings new features, bug fixes, and performance improvements. Every year Apple releases a new version of Swift and this process of releasing new versions is known as the Swift release cycle.
History
In June of 2014, Apple announced Swift, a replacement for the aging Objective-C language. Swift is designed to be more concise and modern than Objective-C, allowing developers to write code that is easier to read and maintain. Swift code also runs faster than its predecessor, as it uses a modern compiler and runtime.
Since then, Swift has gone through a number of releases and updates. Apple released Swift 1 in 2014, followed by Swift 2 in 2015, Swift 3 in 2016, and the latest version, Swift 4.5, was released in 2020. Every major release introduces new features and improvements, and Apple continues to update Swift to generally make it better.
Overview
The Swift release cycle typically follows a certain pattern. First, Apple releases a major version of Swift, which may include significant new features and changes. This is followed by a few minor revisions and bug fixes. Finally, a “ point” release is issued that includes only a small set of changes or bug fixes.
The primary goal of the Swift release cycle is to keep the language up to date and stable. The releases aim to introduce new features and improvements while maintaining backward compatibility. Additionally, Apple takes feedback from the developer community into account when creating new releases.
Major Versions
Major versions of Swift are released on a regular basis, usually once each year. Major versions introduce new features as well as improvements and bug fixes. The most recent major version was Swift 4.5, which was released in 2020.
Major versions are intended to add new features to the language and improve existing features. Some of the new features introduced in Swift 4.5 include support for dynamic callable types, library evolution, thread-safe APIs, source compatibility with Swift 5, new diagnostic capability, and more.
Minor Releases
Minor releases are typically released shortly after major versions. Minor releases do not introduce any new features, but instead focus on improving existing features and fixing bugs.
Minor releases are important because they help ensure that the language is stable and reliable. Minor releases are also important for ensuring backward compatibility.
Point Releases
Point releases are typically small updates that include bug fixes and minor improvements. These releases are intended to be less disruptive than major releases. Point releases are typically released every few months.
Example Usage
Let’s look at an example of how to use Swift’s release cycle. Suppose you have an app that you want to update to use the latest features of the language.
First, you should check the official Apple website to see what the latest version of Swift is. You can then switch your project to that version and start using the new features.
If you find a bug in your code due to a change in the language, you can look for a minor or point release that fixes the issue. If there is no available release that fixes the issue, you can submit the bug to the official Apple bug tracker.
You can also use the Swift release cycle to stay up to date with the latest language features. As new versions are released, you can upgrade your project to that version and immediately start taking advantage of the new features.
Code Examples
Here are some examples of code written in Swift 4.5.
Dynamic Callable Types
Dynamic callable types allow developers to create types that can be called like functions. This allows developers to create custom APIs and closures that can be called like functions.
// Create a simple type that can be called like a function.
struct Sum {
let adder: (Int, Int) -> Int
func callAsFunction(a: Int, b: Int) -> Int {
return adder(a, b)
}
}
let adder = Sum { a, b in
return a + b
}
let result = adder(1, 2)
// result == 3
Library Evolution
Library evolution allows developers to add new APIs to existing types without breaking existing code. This allows developers to add features and improvements to existing types while ensuring backward compatibility.
// Define a basic type.
struct Person {
let name: String
let age: Int
}
// Add a new initializer without breaking existing code.
extension Person {
init(name: String) {
self.name = name
self.age = 0
}
}
let person = Person(name: "John Smith")
// person.age == 0
Conclusion
The Swift release cycle is an important part of the language, as it helps keep the language up to date and stable. Major versions introduce new features, minor releases fix bugs and improve existing features, and point releases are small updates with bug fixes and minor improvements. By understanding and using the Swift release cycle, developers can ensure that their apps are always up to date and stable.