Ada
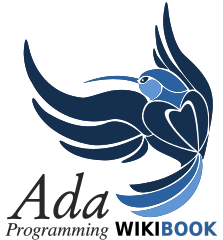
Ada
Ada is a programming language designed by the US Department of Defense and standardized by the American National Standards Institute (ANSI). Its purpose was to increase software reliability and maintainability through the use of robust language features and an emphasis on code readability. Ada is a general-purpose, block-structured language that supports both object-oriented and imperative programming paradigms. It is suitable for a range of tasks from embedded systems programming to large-scale software engineering. The Ada language has been around for over 30 years, and its popularity has continued to grow in recent years. Many organizations are turning to Ada for their critical software development projects due to its robust design and extensibility.
History of Ada
The Ada programming language is one of the oldest high-level programming languages in existence. It was created by the United States Department of Defense (DoD) to serve as a standard for all applications developed for military use. It was named after Lady Augusta Ada Byron, Countess of Lovelace who is credited with creating the first algorithm that could be carried out by a machine. The Ada language first appeared in 1975 and was designed to incorporate structured programming concepts into the language design. The language also featured strong typing, array handling, built-in error detection, and tasking ability. Ada was originally designed to provide a uniform language for embedded systems,or systems running on small microprocessors. It was specifically designed to be reliable, maintainable, and independent of hardware. Ada was adopted as the official DoD standard for systems software development in 1982. The year after, Ada was made available to the public. In 1987, Ada was revised and some features were added such as object-oriented programming and support for distributed systems. Starting in 1995, the Ada language has been revised every two years. Since its inception, Ada has been used in various real-world applications. It has been used by NASA, the U.S. Army, and the U.S. Navy. It has also been used in many commercial projects, including telecommunications systems and software engineering tools.
Ada was developed in 1979 as part of the US Department of Defense’s Ada Joint Program Office initiative. The goal was to create a programming language that was reliable, maintainable, and easily adaptable to different computing environments. At the time, there were many programming languages available, but none of them provided the specific capabilities needed by the military. Ada was designed to address these needs while also being expressive and easy to read and understand. In 1983, Ada was officially adopted by the US military and became a standard language for software development in the defense sector. Since then, Ada has become popular for a variety of applications, ranging from embedded systems to large-scale distributed software projects.
Examples of Ada Usage
Ada is widely used in many different industries. Here are just a few examples:
- Aerospace: Ada has been used in the Aerospace industry since the early days of the DoD’s adoption of the language. Major aerospace companies have used Ada in their flight-control systems.
- Telecommunications: Ada has been used in many telecommunications systems, including aircraft control systems, satellites, and radio relays.
- Medical: Ada has been used in medical devices such as pacemakers and blood analyzers.
- Automotive: Ada has been used in many automotive systems, including engine management systems, driver assistance systems, and autonomous vehicles.
Features
Ada is a modern, high-level, general-purpose programming language with many features such as:
- Strong type checking
- Static type bindings
- Dynamic binding
- Polymorphism
- Object orientation
- Concurrency abstraction
- Exception handling
- Parametric generics
- Data abstraction
Ada also has a number of other features, such as:
- Strong modularity
- Portable source code
- Verification tools
- Readability
- Good support for real-time systems
Ada is considered a “high-level” language and is designed to be easy to read and comprehend. Its syntax is based on Algol, a programming language developed in the late 1950s. This helps people with a background in Algol feel comfortable reading and writing Ada code. Ada has a number of features that make it attractive for programming in the real world. It has strong type checking and built-in error detection, which help ensure reliable software. It also supports tasking, which allows developers to write applications that can manage multiple tasks at the same time. Ada also provides built-in debugging features such as dynamic checks, assertions, and contracts that make it easier to debug programs written in Ada. Finally, Ada is designed to be portable, so programs written in Ada can run on different platforms without needing to be rewritten.
Ada is a strongly typed language with a focus on safety and reliability. It has a wide variety of features, including:
- Ada records, which allow for the definition of complex data structures.
- Object-oriented programming support, including classes and inheritance.
- Exception handling for graceful errors recovery.
- Parameterized types for creating generic data structures.
- Assertions for describing expected behavior.
- Tasking for concurrent programming.
- Pointers and memory management.
- Static type checking at compile-time.
Syntax
Ada is a declarative language that follows an imperative style of programming. It follows the ALGOL-60 syntax, which allows for easy readability. The main elements of Ada are packages, subprograms, types, and tasks. Packages are like containers that contain all the entities related to a particular concept or domain. The package statement declares a package and defines its scope. Subprograms consist of functions and procedures. They are similar to functions and procedures in other languages. Types define the set of values and operations available to objects. Primitive types such as integers and reals are supported in Ada. Tasks provide a concurrent execution model and are used when a program requires multiple processes to be running at the same time.
Libraries
Ada includes a standard library containing essential functionality for writing applications. It includes:
- Standard I/O
- String manipulation
- Math functions
- Bit manipulation
- Interprocess communication
- Network programming
- Time and date functions
- Standard data types
- Tasking primitives
- Interfacing with other languages
The Ada library also includes additional libraries for more specific tasks, such as:
- Graphics
- Graphical user interfaces
- Database access
- Image processing
- Multimedia
- Web programming
- Embedded system programming
Examples
Here is an example of a simple Ada program that computes the average of two numbers:
-- Compute the average of two numbers
with Ada.Text_IO; use Ada.Text_IO;
procedure Average is
Number1 : Integer;
Number2 : Integer;
begin
Put("Enter first number: ");
Get(Number1);
Put("Enter second number: ");
Get(Number2);
Put_Line("The average of these numbers is: " & Integer'Image((Number1 + Number2) / 2));
end Average;
When compiled and run, this program produces the following output:
Enter first number: 6
Enter second number: 10
The average of these numbers is: 8
We can also use Ada for more complex tasks such as creating a graphical user interface (GUI). Here is an example of a simple GUI program that prints a message when a button is clicked:
with Ada.Text_IO; use Ada.Text_IO;
with Gtk.Handlers; use Gtk.Handlers;
with Gtk.Main;
procedure Hello_World is
Main_Window : Gtk_Window;
Button : Gtk_Button;
begin
Main_Window.Create;
Main_Window.Set_Title("Hello World");
-- Create a button and connect it to a click handler
Button.Create(Main_Window);
Button.Set_Label("Click Me!");
Connect_Clicked_Handler(Button, Click_Handler'Access);
Main_Window.Show_All;
Gtk_Main;
exception
when Gtk.Error =>
Put_Line("Error: " & Gtk.Error'Message);
end Hello_World;
-- Handler called when the button is clicked
procedure Click_Handler is
begin
Put_Line("Button clicked!");
end Click_Handler;
When compiled and run, this program produces a GUI window containing a button. When the button is clicked, it prints the message “Button clicked!” in the console.
Here is an example of Ada code that prints out the words “Hello, world”:
with Ada.Text_IO;
procedure Hello is
use Ada.Text_IO;
begin
Put_Line ("Hello, world");
end Hello;
This code uses the Ada.Text_IO library to print the phrase “Hello, world” to the screen.
Ada is a high-level programming language, so most of the code is highly readable and easy to understand. Here is an example of a simple program written in Ada:
with Ada.Text_IO;
use Ada.Text_IO;
procedure Hello is
begin
Put_Line ("Hello World!");
end Hello;
This program prints out “Hello World!” to the console.
Another example is a function that calculates the greatest common divisor (GCD) of two integers:
with Ada.Integer_Text_IO;
use Ada.Integer_Text_IO;
function GCD(A, B : Integer) return Integer is
Result : Integer := 1;
begin
if A = 0 or B = 0 then
return Result;
end if;
loop
exit when A mod B = 0;
Result := B;
B := A mod B;
A := Result;
end loop;
return B;
end GCD;
This function takes two integers as input and returns their greatest common divisor as output.
Conclusion
Ada is a powerful, reliable programming language that is suitable for a range of applications, from embedded systems to large-scale software engineering projects. It has a wide variety of features that make it well-suited for safety-critical software development.
Ada is still widely used today and is gaining popularity due to its robustness, extensibility, and safety features. Many organizations have adopted Ada for their critical software development projects due to its robust design and extensibility.
Ada is an advanced, open-source language designed for building reliable, efficient and maintainable software. It is a strongly typed language that supports features such as coherent data structures, OOP, exception handling and memory management. Its wide range of usage in various domains from military to banking makes it a valuable asset for any software developing enterprise.