Julia
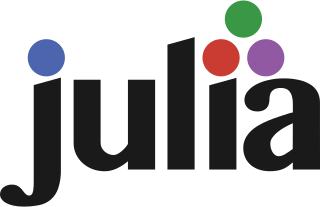
Introduction to Julia
Julia is a high-level, dynamic, general-purpose programming language with features that allow programmers to express their ideas in fewer lines of code than would be possible in other programming languages. Julia was created with the goal of becoming a more powerful and productive language than any other existing language, while still remaining easy to read and understand. Julia uses multiple dispatch as a paradigm, making it easy to express many forms of programming in an expressive yet concise manner. Julia provides a rich system of libraries and packages that support the development of a wide range of applications and libraries.
History
Julia was initially developed in 2009 by Jeff Bezanson, Alan Edelman, Stefan Karpinski, and Viral Shah. In 2012, the Julia project began releasing versions of Julia to the public. Since then, it has experienced a rapid growth in popularity and recognition. As of 2021, Julia is used in many scientific and technological areas, such as economics, finance, data science, machine learning, deep learning, natural language processing, artificial intelligence, robotics and much more. Julia was inspired by several other programming languages such as Lisp, Python, Ruby, Scheme, Lua, FORTRAN, C, APL and MATLAB. The goal of Julia was to combine the speed of compiled languages such as C and FORTRAN with the accessibility of scripting languages such as Python. Julia’s initial development began in August of 2009, when its creators announced it on the Julia Language Google Group. In August 2012, Julia version 0.1 was released with significant improvements to the language’s basic infrastructure. This led to a rapidly growing and dedicated user base over the next several years. In January 2016, Julia version 1.0 was released, introducing language stability and adding many performance improvements. Following the release of version 1.0, more than 1,000 packages were developed for the language. In October 2017, Julia version 1.1 was released, which added support for scientific plotting, parallel computing, interactive computing, data manipulation, and graphics.
Since its creation in 2009, Julia has become popular among developers, data scientists, researchers, investors, and financial analysts.
Features
Julia has several unique features that make it an ideal language for various programming tasks. Some of these features include:
- First-class functions and macros
- Type inference
- Multiple dispatch
- A comprehensive standard library
- Built-in package manager
- A fully featured REPL
- AJIT compiler
- Parallel computing support
- Ambiguous syntax
- Comprehensive documentation
Examples of Usage
One of the most common uses of Julia is machine learning applications. Julia provides a number of libraries and packages that help developers create programs that can quickly and effectively process large amounts of data. For example, the TensorFlow.jl package provides Julia developers with access to powerful deep learning algorithms, such as Convolutional Neural Networks (CNNs).
Another popular usage for Julia is for numerical analysis. Julia has a number of libraries and packages that are tailored for this purpose, such as the DifferentialEquations.jl package, which allows developers to quickly solve complex equations.
Julia is also well suited for web development, with frameworks such as Genie.jl providing the necessary infrastructure to develop websites and web applications with the language.
What You Can Do With Julia
Julia can be used for a wide variety of tasks, ranging from data analysis and scientific computing to web development and machine learning. Here are some examples of what you can do with the language:
-
Data Analysis: Julia has a wide range of libraries, packages, and tools that make it well suited for data analysis. It is especially useful when it comes to analyzing large datasets and visualizing results.
-
Scientific Computing: Julia is used in many scientific and engineering fields, including meteorology, economics, astronomy, and physics. It is also well suited for numerical optimization and machine learning applications.
-
Web Development: Julia can be used for web development. It provides a suite of libraries and packages for building web applications.
-
Machine Learning: Julia is becoming increasingly popular for machine learning applications. It provides a variety of powerful libraries and frameworks such as TensorFlow, Scikit-learn, and MLBase.
Advantages of Julia
Julia has many advantages over other programming languages. First, it is incredibly fast and lightweight. Contrasted with Python, for example, it can run a significant amount of code in less time and with far fewer resources. In addition, Julia was designed to be highly accessible and intuitive, making it very attractive to those new to programming. Its syntax is simple and expressive, which makes it easy to learn and read. Finally, Julia has one of the most comprehensive standard libraries available, making it great for tackling complex tasks.
Uses of Julia
Julia can be used for many different tasks, but is strongest in scientific and numerical computing. It can be used for data analysis, machine learning, deep learning, natural language processing, image and audio processing, scientific simulation, and much more. For example, Julia can be used for data exploration and visualization. Using Julia’s powerful visualizations library, users can quickly explore data sets to develop insights. Julia can also be used for creating and testing models. Using a combination of its machine learning, deep learning and optimization libraries, models can be created and tested quickly. Julia is also well suited for high-performance computing tasks. It features an efficient garbage collection system and supports parallel computing, allowing users to easily split tasks across multiple processors. This makes it possible to tackle computationally intensive tasks easier and faster. Finally, Julia can be used as a scripting language, making it an ideal choice for automation. With its simple and expressive syntax, users can quickly create scripts to automate routine tasks.
How to Use Julia
Julia can be used either in an interactive shell or a script file. To use Julia in an interactive shell, you first need
to install Julia. Once you have done this, open a terminal window and type julia
. This will launch the Julia
interactive shell. From there, you can start writing Julia code and see the results immediately.
To use Julia in a script file, you first need to create a file with a .jl
extension. This file should be placed in a
directory where you have write permission. Then, you can open this file and start writing your code. To execute the
code, simply run the command julia filename.jl
. This will execute the code in the given file and return any outputs.
Features
Julia is a dynamically typed language, meaning type checking is performed at runtime rather than compile time. This allows for much greater flexibility in code structure and also enables multiple dispatch, or the ability to make a single method work with different arguments. Julia also provides an interactive REPL (read-execute-print loop) environment for rapid development and debugging. The language also offers advanced features such as data streaming and parallelism, allowing users to efficiently process large datasets in a distributed fashion. Julia’s built-in plotting libraries make it easy to visualize data, create interactive maps, and visualize complex equations. Additionally, Julia integrates with existing libraries and packages from other languages, making it easier than ever to extend its functionality.
Examples
Here are some examples of how Julia can be used:
- Numerical Analysis: Solving systems of equations, performing linear algebra operations, solving differential equations, and numerical optimization.
- Data Analysis: Processing large datasets, creating visualizations, building machine learning models, and performing statistical analysis.
- Scientific Computing: Programming robots, creating simulations, optimizing processes, and designing experiments.
Code Examples
Here is an example of basic Julia code that performs elementwise addition on two vectors:
a = [1, 2, 3]
b = [4, 5, 6]
c = a .+ b # c = [5,7,9]
And here is an example of using the @parallel
macro to run a computation on multiple cores:
data = rand(1000) # generate random data
@parallel for i in 1:1000
do_something(data[i]) # perform a task on each data point
end
Here is an example of a basic “Hello World” program written in Julia:
println("Hello World!")
This code will print the message “Hello World!” to the console.
Here is an example of how to define a function in Julia:
function square(x)
return x^2
end
This code defines a new function called “square” which takes one argument and returns the square of that argument. For example, if we call the function with the argument 5, it will return 25.
Below are some examples of programming code written in Julia:
println("Hello World!")
Fibonacci Sequence
function fibonacci(n)
if n <= 2
return 1
else
return fibonacci(n-1)+fibonacci(n-2)
end
end
println(fibonacci(10)) #prints 55
Data Visualization
using Plots
x = linspace(0, 10, 100)
y = sin(x)
plot(x, y)
Below are some examples of basic Julia code:
Printing to the Terminal Window:
println("Hello World")
Creating Variables:
x = 5
y = 10
Using if Statements:
if x > y
println("x is greater than y")
end
Using Loops:
for i=1:10
println(i)
end
Creating Functions:
function myfunc(a, b)
c = a + b
return c
end
Conclusion
Julia is a high-level, dynamically-typed, general purpose programming language, designed specifically for technical computing. It has a rich set of libraries and packages that make it well suited for data analysis, scientific computing, web development, and machine learning applications. There are a wide array of programming examples available for Julia, making it easy to get started with the language. Julia is an efficient, dynamic programming language with a variety of features ideal for rapid development and numerical computing. Its high performance, interactive environment, and integration with existing libraries make it a powerful and versatile solution for a variety of applications.