C#
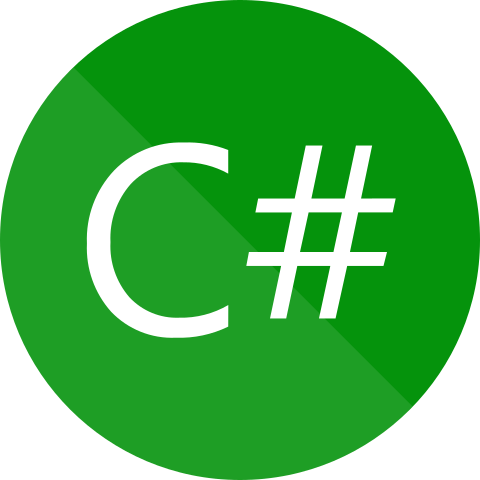
Introduction
C# is a multi-paradigm programming language encompassing strong typing, imperative, declarative, functional, generic, object-oriented (class-based), and component-oriented programming disciplines. It was developed around 2000 by Microsoft within its .NET initiative and later approved as a standard by Ecma (ECMA-334) and ISO (ISO/IEC 23270:2006). C# is one of the programming languages designed for the Common Language Infrastructure. C# is a powerful and versatile programming language used for a variety of tasks. It is well-suited for developing Windows applications, web services, cloud applications, mobile applications, and more. With its vast libraries and support for object-oriented programming, C# is a great choice for developers who want a powerful and flexible language. C# (pronounced “C Sharp”) is a multi-paradigm programming language developed by Microsoft that runs on the .NET Framework. It was designed to provide a simple and unified programming language for different types of applications, including mobile, web, enterprise, and desktop. C# is mostly used for writing business applications, web services, desktop applications, game development and more.
History
C# is a modern, strongly typed programming language that was created in 2000 by Anders Hejlsberg at Microsoft. Hejlsberg wanted to bring the power of C++ to the world of Object Oriented Programming (OOP), while simplifying the syntax and minimizing the complexity of development. The project started as an internal Microsoft project and had many private code names such as Cool, Rotor, and COOL#. The official name of C# was finalized in April 2001, shortly before its public announcement at the Professional Developers Conference (PDC) in July 2001. Since then, the language has been evolving rapidly. In 2002, the first version of C# was released as part of the .NET Framework 1.0. Since then, there have been numerous versions released with new features, improvements, and bug fixes. C# was first developed by Microsoft in 2000 as a part of their .NET initiative. It was designed by Anders Hejlsberg, who also designed Turbo Pascal and Delphi. C# was an evolution from the C-family of programming languages and came out as a simpler and more streamlined option for developers. Over the years, C# has become the most popular language for developing Windows applications.
Basics
C# is a statically-typed language, which means that the type of a variable is known at compile-time and cannot be changed. This can help catch errors early on in the development process, as opposed to a dynamically-typed language like JavaScript, where type errors may only appear at run-time. C# also supports strongly-typed generic collections, which provide better type safety than non-generic collections. C# makes use of the Common Language Runtime (CLR) which allows the language to be platform independent, meaning that C# code can be compiled for a variety of platforms, including Windows, Mac OS X, and Linux. The CLR also provides features such as garbage collection, type safety, and exception handling, which helps make C# development more robust and efficient.
Syntax
C# syntax is based on the C programming language and shares many of the same features, including variables, classes, functions, and control flow statements. It also supports object-oriented concepts such as encapsulation, inheritance, and polymorphism. Below is a basic example of C# code that prints out “Hello, World!”
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
}
The using
directive imports a namespace, in this case the System
namespace. A namespace is used to organize code and
prevent naming conflicts – it allows you to have multiple classes with the same name, but in different namespaces.
The namespace
keyword defines a new namespace, while the class
keyword defines a class. The static
keyword
indicates that a method can be called without creating an instance of the class. The void
keyword indicates that this
method does not return a value. The Main
method is called when a program starts execution. Finally, the Console
class provides a WriteLine
method which prints out the argument passed to it.
C# is a powerful language used to create a wide variety of applications, ranging from web and mobile applications to desktop applications and games. It is used by many companies, including Microsoft, Amazon, Adobe, Accenture, and Oracle. It is particularly well-suited for the development of Windows applications, as it has access to the full set of Windows APIs, and it supports native Windows components such as user interfaces, multimedia, web services, databases, and more. C# is also used to create applications for Android and iOS, using frameworks such as Xamarin. With Xamarin, developers can share the same codebase across platforms, allowing them to quickly and easily create apps for multiple platforms. C# is also commonly used to develop backend systems, such as web services, cloud applications, and more. It is easy to integrate with various data sources, and it offers support for asynchronous programming, making it well-suited for developing large-scale applications.
Below is an example of code that calculates the mean and standard deviation of a set of numbers.
using System;
public class Mean
{
static void Main()
{
double[] numbers = new double[5] { 1.0, 2.0, 3.0, 4.0, 5.0 };
double sum = 0.0;
foreach (double number in numbers)
sum += number;
double mean = sum / numbers.Length;
double sumOfSquares = 0.0;
foreach (double number in numbers)
sumOfSquares += Math.Pow(number - mean, 2);
double standardDeviation = Math.Sqrt(sumOfSquares / (numbers.Length - 1));
Console.WriteLine("Mean = {0}", mean);
Console.WriteLine("Standard Deviation = {0}", standardDeviation);
Console.ReadLine();
}
}
In this example, we use the Math
class to perform mathematical operations, such as calculating the square root of a
number. We then calculate the mean and standard deviation of the numbers. Finally, the results are printed out to the
console.
A Short History of C#
C# is a powerful, general-purpose programming language created by Microsoft as part of the .NET initiative in 2000. Originally designed for developing applications on Windows, C# has since become a popular language for building applications and services for the web, mobile, desktop, and cloud.
C# was designed with the goal of making developers more productive, enabling them to quickly and easily create robust and maintainable apps for any platform and environment. It combines the best features of object-oriented programming ( OOP) such as type safety, inheritance, and polymorphism with modern language innovations such as generics, lambda expressions, and asynchronous programming.
Pre-C#
C# evolved from a programming language called C–, which was released in 1985 as an extension of the original C language. The design of C– was based on the language constructs of C and Simula, an object-oriented language from 1967. C– introduced many of the features that are now present in C#, including classes, inheritance, and virtual methods.
In the late 1990s, Microsoft began work on a new platform called ActiveX, which allowed developers to create interactive applications and distribute them over the Internet. While ActiveX primarily used Visual Basic and JavaScript, Microsoft was looking for a new, more powerful language they could use to develop their own applications.
Introducing C#
In 2000, Microsoft released C# (pronounced “See Sharp”) as part of the .NET framework. This new version of the language was designed to be a better and more effective language for developing Windows applications. It featured a simplified syntax, OOP techniques, garbage collection, and support for modern language features such as generics.
The release of C# 1.0 included a set of libraries for accessing the Windows API and networking protocols. This library, called the .NET Framework Class Library (FCL), became the foundation for the .NET ecosystem and provided developers with a comprehensive set of data structures, collections, and more.
Since its initial release, C# has gone through several versions, each introducing new language features and enhancements to improve performance and make development easier.
C# 2.0
Released in 2005, C# 2.0 introduced several features that helped to simplify development and make it easier to debug code. It introduced generics, which allow developers to create types that can work with any type of data; iterators, which enable developers to easily create custom iterator classes; and anonymous methods, which allow developers to quickly create small methods without needing to define a separate class.
C# 3.0
In 2007, C# 3.0 was released with a focus on language-level support for LINQ (Language Integrated Query). LINQ
introduces a set of query operators such as Where()
and Select()
that make it easy to filter and transform data. It
also adds support for lambda expressions, which allow developers to write code using a functional-style syntax.
C# 4.0
In 2010, C# 4.0 introduced several features that made it easier to interact with dynamic languages such as Python, Ruby, and JavaScript. It also added support for covariance and contravariance, which enables developers to write more flexible code when working with generics.
C# 5.0
C# 5.0 was released in 2012 with the major feature being support for asynchronous programming. This allows developers to
write code that responds to events asynchronously, without blocking the main thread. C# 5.0 also introduced the await
keyword, which makes it easier to write asynchronous code.
C# 6.0
C# 6.0, released in 2015, included a number of new language features that make it easier to write code. This includes
auto-property initializers, string interpolation, and expression-bodied members. C# 6.0 also adds support for
the async
and await
keywords, which make it simpler to write asynchronous code.
C# 7.0
C# 7.0 adds several new language features to make coding faster and simpler. This includes pattern matching, tuples,
local functions, and more. It also adds support for the is
operator, which allows developers to check if an object is
of a specific type.
C# 8.0
Released in 2019, C# 8.0 adds a number of new language features, including nullable references, async streams, records,
and ranges. It also adds support for the switch
expression, which allows developers to match multiple values without
needing to use multiple if/else
statements.
History of C#
C# was first introduced in 2000 as part of Microsoft’s .NET initiative. It was created by Anders Hejlsberg, who is also the designer and architect of the programming language Delphi. The language was built upon the success of existing languages such as Java and C++, offering a higher level of abstraction with more powerful features.
C# quickly became popular for its flexibility and ease of use, offering developers a faster and more productive development environment than traditional compiled languages. This popularity has only increased over the years, with the language now being used to develop a vast number of applications across many different platforms.
Features of C#
C# includes a range of powerful features that make it an ideal choice for developing a variety of applications. These include:
-Strongly Typed: C# is a strongly typed language, meaning that all variables must be declared before they are used and all operations must be performed on compatible types. This helps to prevent errors and ensures that code is consistent and reliable.
-Object Oriented: C# is an object-oriented language, meaning that it is designed around the principles of object-oriented programming. This makes it easier to manage and maintain large amounts of code, as objects can be reused and extended to create new functionality.
-Memory Management: C# has an efficient memory management system, which allows developers to manage how data is stored and accessed. This can help to ensure that resources are used efficiently and performance is improved.
-Language Interoperability: C# can interoperate with other .NET languages, such as Visual Basic and C++. This allows developers to easily share code between projects and make use of any existing code libraries.
Examples of Usage
C# can be used to create a wide range of applications, including desktop applications, web applications, mobile applications, games, and more. Here are some examples of the types of applications that can be created with C#:
- Windows Applications: C# can be used to create traditional desktop applications that run on the Windows operating system. These applications can access the full range of Windows APIs, allowing developers to create powerful, feature-rich applications.
- Web Applications: C# can be used to create dynamic websites using the ASP.NET framework. This allows developers to create fast, interactive web applications with features such as user authentication, databases, and more.
- Mobile Applications: C# can be used to create native mobile apps for iOS and Android using the Xamarin platform. Xamarin allows developers to create apps that look and feel native on both platforms, and can even share code between the two for increased efficiency.
- Games: C# can be used to create 2D and 3D games using the Unity game engine. Unity provides a powerful game engine, along with a wide range of tools and plugins, making it easy to create stunning 3D games.
Code Examples
Here are some simple examples of C# code that demonstrate some of the language’s features:
Hello World Program
This is a basic example of a “Hello World” program written in C#:
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
}
}
}
This program will print the text “Hello World!” to the console.
Class Declaration
Here is a simple example of a class declaration in C#:
class Person
{
public string Name { get; set; }
public int Age { get; set; }
public Person(string name, int age)
{
Name = name;
Age = age;
}
}
This example shows a basic class declaration, which includes a constructor that sets the name and age of the person.
Lambda Expression
This is an example of a lambda expression in C#, which can be used to define an inline function:
Func<int, int, int> addNumbers = (x, y) => x + y;
Console.WriteLine(addNumbers(3, 4)); // Outputs 7
In this example, we define a lambda expression to add two numbers together and then call the function with the values 3 and 4. This will print the result 7 to the console.
Here is a simple example of a C# program which prints the text “Hello World!” to the console:
using System;
public class Program
{
public static void Main()
{
Console.WriteLine("Hello World!");
}
}
When this code is executed, it will print the text “Hello World!” to the console.
Conclusion
C# is a versatile programming language that is used for a variety of applications. It is easy to learn, has a robust library of functionality, and is tightly integrated with the .NET framework. It provides powerful tools for debugging and testing, and it is object-oriented which allows developers to use the same patterns and practices across different platforms. C# is used for developing Windows applications, web services, games, databases, and more.