Dart
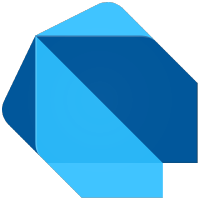
Introduction
Since its introduction in 2012, Dart has become a popular choice among developers for its powerful features and ease of use. With its support for multiple paradigms, fast compilation times, and easy interoperability with JavaScript, Dart is well suited for both web and mobile development. Originally developed by Google in 2011, Dart was designed to provide a better development experience than Java or JavaScript. It has since evolved into its own language with a platform independent compiler and a standard library. Dart is an object-oriented, class-based, garbage-collected programming language with C-style syntax developed by Google and used to create mobile, desktop, server, and web applications. It is open-source, and can be used for both client-side and server-side development. Dart was created by Lars Bak and Kasper Lund in October 2011, released to the public in October 2012, and announced as a production language in November 2013. The initial version supported both the virtual machine and JavaScript, but the dual-mode feature was removed in version 2. Dart is a programming language developed by Google and used to build web, server, mobile, and desktop applications. It is an open-source client-optimized language with a focus on simplicity and performance. Unlike many other languages, Dart compiles to both JavaScript and native code.
History
Dart was first announced at the GOTO conference in October 2011, and its first version was released in 2013, 2 years later. It was designed to create more structured, scalable, and faster web apps than ever before. The idea behind the language was to make it easier to develop web apps and to make it faster to compile the code. Since its initial release, Dart has undergone several updates, including the addition of new features and bug fixes. In May 2017, the release of Dart 2.0 brought additional language improvements such as improved type inference, modernized syntax, and support for generic types. One of the language’s main goals is to replace JavaScript as the language of choice for web and mobile development. The idea is that Dart will be faster, easier to write, and more reliable than JavaScript.
Usage
Dart is used to build web apps and mobile apps, as well as server-side applications. It is popular among developers because of its ease of use and the fact that it is compiled to both JavaScript and native code. Some of the most popular apps that use Dart are Adobe Creative Suite, Google Ads, and Flipkart. The language is especially suitable for developing complex web applications, as it allows developers to use object-oriented programming principles. Dart also supports several programming paradigms, including functional programming and reactive programming.
Features
Dart provides several features that make it well suited for web development. First, Dart is strongly typed, which means that it checks for errors at compile time rather than waiting for run time. This ensures that the app will not crash due to an invalid operation. Second, Dart has an excellent garbage collection system. This system helps manage memory usage and helps prevent memory leaks. Third, Dart’s native code compilation allows it to be used to create high-performance web apps and mobile apps. Additionally, the language supports asynchronous programming, which makes it easier to write concurrent programs. Finally, Dart is an open-source language and is supported by a large community of developers. This allows developers to get help when they need it and to contribute their own solutions.
Dart’s most notable features include:
- Support for multiple paradigms (object-oriented and functional)
- Fast compilation times
- A rich, standard library
- Strong type safety and code optimization
- Easy interoperability with JavaScript
Dart has several features designed to make it fast and easy to use. It has an Ahead-of-Time (AOT) compiler that compiles code into native machine code for both the client and server-side. The client-side code can run on both mobile and desktop platforms, while the server-side code can run on both Windows and Linux. The language also supports strongly typed, class-based, object-oriented programming, with support for generics, abstract classes, and interfaces. It has a unified type system, with support for both primitive and class types, and a rich library of core and third-party packages. Finally, it supports several modern language features such as async/await and mixins. Dart offers some features that are not available in other languages. These features include native support for asynchronous programming, a structural type system, an optional type inference system, garbage collection, and an integrated package manager. Additionally, Dart has many popular libraries and frameworks, such as Flutter and AngularDart.
Examples of Using Dart
The following are examples of using Dart in various ways:
Client-Side Development
Dart can be used to create web applications or mobile apps using Flutter framework. Flutter is a UI toolkit built with the Dart language, which can be used to build beautiful, high-performance mobile applications for both iOS and Android.
Server-Side Development
Dart can also be used for server-side development. It can be used to create web servers, APIs, and other server-side applications. It can also be used to write command-line tools and scripts.
Desktop Applications
Dart can be used to create graphical user interfaces (GUIs) for both desktop and mobile applications. It can be used to create cross-platform applications which can run on Windows, macOS, and Linux.
Libraries and Frameworks
Dart has a rich library of core and third-party packages, designed to make development faster and easier. These packages can be used to create server-side applications using such frameworks as Aqueduct and Angel, create desktop applications using Electron, or create mobile apps using Flutter.
Code Examples
The following is a simple “Hello World!” example in Dart:
void main() {
print('Hello, World!');
}
The following is an example of using the Flutter framework to create a simple, Hello World mobile application.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Welcome to Flutter',
home: Scaffold(
appBar: AppBar(
title: Text('Welcome to Flutter'),
),
body: Center(
child: Text('Hello World!'),
),
),
);
}
}
Dart can be used to create a wide variety of applications, ranging from web servers to mobile and desktop applications. Popular apps built with Dart include Google Ads Developer, Google Ad Manager, and Discord.
Dart is also well suited for building server-side applications, as it allows developers to create high-performance, cross-platform applications with fewer resources.
Here are some code examples to demonstrate how to use Dart.
Hello World Program:
void main() {
print('Hello World');
}
Creating a Function:
// Defining the function
int addTwoNumbers(int number1, int number2) {
return number1 + number2;
}
// Calling the function
int result = addTwoNumbers(2, 4);
print(result); // 6
Creating a Class:
// Defining the class
class Person {
String name;
int age;
Person(this.name, this.age);
void sayHello() {
print('Hello, my name is $name and I am $age years old.');
}
}
// Creating an instance of the class
Person person = Person('John', 30);
person.sayHello(); // Hello, my name is John and I am 30 years old.
Below is a simple example of a Dart program that prints “Hello World”:
void main() {
print('Hello World!');
}
The snippet above shows how easy it is to create a basic program in Dart. The program prints “Hello World” to the console.
Below is an example of a function written in Dart. This function takes two numbers as parameters and returns the sum of the two numbers:
int sum(int num1, int num2) {
return num1 + num2;
}
In this example, the function sum
takes two numbers as parameters and returns the sum of the two numbers.
Below is an example of an asynchronous function written in Dart. This function fetches data from an API and prints the result to the console:
Future<void> fetchData() async {
var response = await http.get('https://example.com/api');
if (response.statusCode == 200) {
print(response.body);
} else {
print('Error!');
}
}
This example demonstrates how easy it is to use asynchronous programming in Dart. The fetchData
function makes an HTTP GET request to the given URL and prints the response body to the console.
Here is an example of a basic “Hello World” program written in Dart:
void main() {
print("Hello World!");
}
And here is an example of an application that prints the square root of a number:
import 'dart:math';
void main() {
// Get a number from the user
var input = stdin.readLineSync();
int number = int.parse(input);
// Calculate the square root
var result = sqrt(number);
// Print the result
print("The square root of $number is $result");
}
Conclusion
In conclusion, Dart is a powerful language with versatile capabilities. It can be used to create a wide variety of applications, from server-side solutions to mobile and desktop apps. With its features, libraries, and frameworks, it has become a popular choice for developers looking to build modern applications.