Haskell
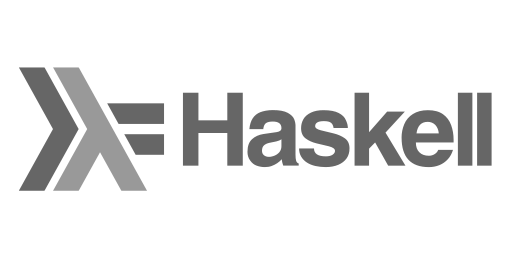
Introduction
Haskell is a purely functional programming language. It is a general-purpose language, meaning it can be used to create anything from small scripts to large enterprise applications. Haskell gained popularity in the early 2000s and has since seen an increase in its adoption in the industry, in academia, and by hobbyists. Haskell is a standardized, general-purpose, polymorphically statically typed, purely functional language, with non-strict semantics and strong static typing. It is named after the logician Haskell Curry. Haskell is widely used in academia and industry.
History
The development of Haskell started in the late 1980s. The first version of the language, named Haskell 1.0, was released in 1990. This was followed by successive language releases of Haskell 98 in 1998, Haskell 2010 in 2010, and most recently Haskell 2018 in 2018. In 2003, an open source implementation of the language, the Glasgow Haskell Compiler (GHC), was released. Since then, GHC has become the de facto standard for Haskell implementations, being actively developed and widely used in both industry and academia. In 2007, the Haskell community began to create the Haskell Platform for easier distribution and deployment of the language. This platform allows users to easily install the compiler, language libraries and other associated tools. Haskell was first released in 1990 by Paul Hudak and Simon Peyton Jones at Yale University. It was designed with the goal of providing a purely functional language that could be used for both research and practical purposes. The language was initially called “Goedel2”, but was later renamed Haskell after the logician Haskell Curry. Since its initial release, Haskell has gone through multiple iterations and releases, as well as numerous libraries. It also has a thriving open source community that contributes to the development of the language.
The current version of Haskell is Haskell 2018. This version includes several features, such as separate compilation, advanced pattern matching, and support for new language extensions. In addition, several other features such as generalized algebraic data types, extensible records, and implicit parameters were added.
Features
One of the major characteristics of Haskell is that it is a purely functional language. This means that all functions are pure mathematical functions, meaning they have no side effects and always produce the same result when given the same inputs. Another feature of Haskell is its laziness. Haskell is a lazy language, meaning that it will only evaluate expressions when they are needed. This lazy evaluation allows for more efficient memory management and enables developers to write code that is more modular and maintainable. Haskell also has a strong static type system. This means that the types of variables and functions are checked at compilation time and any errors are caught early on in the development process. This makes it easier to refactor code and prevents many bugs from being introduced into the program. Lastly, Haskell has a robust suite of libraries available. These libraries provide a wide range of functionality including graphics, web development, databases, data structures, and more.
Haskell has a number of features that make it unique and attractive to programmers. These features include:
- A Lazy Evaluation Model: In Haskell, computations are not evaluated until their result is needed. This makes the language particularly well suited for situations where some data may never be used. This also leads to faster program execution times and reduced memory usage.
- Purely Functional Design: Unlike other programming languages, Haskell is designed to be purely functional. This means that the code is free of side-effects and is composed of functions that take one input and return one output. As a result, programs written in the language are predictable and reliable.
- Rich Type System: Haskell has a rich type system. This enables programs to have strong guarantees about the types of data they are dealing with. This reduces the chances of errors occurring due to incompatible data types.
- Concurrent and Parallel Computing Support: Haskell makes it easy to write programs that can take advantage of multiple cores on a computer. The language features a range of abstractions and libraries that allow developers to easily write code that can run in parallel or concurrently.
- Compiler & Interpreter Support: Haskell comes with both a compiler and an interpreter. This allows programmers to prototype their ideas quickly using the interpreter, and then use the compiler to produce optimized code for deploying applications.
Libraries
Haskell has a wide variety of libraries and tools available. Some popular libraries include:
base
: the standard library that comes with the compiler,containers
: a library providing data structures,parsec
: a library providing parsing combinators,text
: a library for manipulating Unicode text,vector
: a high-performance library for vectors,lens
: a library for immutable data structures,hmatrix
: a library providing matrix operations,conduit
: a library for streaming data,yesod
: a web framework.
Examples
Below are some examples of basic Haskell programs:
Hello World
Here’s an example of a simple Hello World program in Haskell:
main = do
putStrLn "Hello World!"
This program prints out Hello World!
to the console.
Fibonacci Sequence
The Fibonacci sequence is a classic example of recursion in programming, and can easily be implemented in Haskell. Here is an example of a recursive Fibonacci function:
fibonacci 0 = 0
fibonacci 1 = 1
fibonacci n = fibonacci (n-1) + fibonacci (n-2)
This function takes in a single argument, n
, and returns the n
th number in the Fibonacci sequence.
Factorial
Factorial is another classic example of recursive programming, and can be implemented as such in Haskell:
factorial 0 = 1
factorial n = n * factorial (n-1)
This function takes in a single argument, n
, and returns n!
.
Examples
Here is a simple example of a Haskell program:
main = do
putStrLn "Hello, World!"
This program prints the string “Hello, World!” to the console.
Here is a more complex example of a Haskell program:
import Data.List
main = do
let xs = [1,2,3,4]
print $ maximum $ permutations xs
This program finds and prints the maximum permutation of the list xs
.
Syntax
Haskell has a very concise and concise syntax. Here is an example of a simple program:
double x = x * 2
main = do
print $ double 5
This program prints the value 10 to the console.
The following example shows how to create a simple web server in Haskell:
import Network.HTTP.Server
import Network.HTTP.Types
main = do
let s = server { port = 8080 }
-- set up the server
serve s $ \rq ->
-- handle requests
return $
-- return a response
responseLBS status200 [] "Hello World!"
This code will set up a basic web server on port 8080. When a request is received, the code will send back a response with the status code 200 (OK) and the message ‘Hello World!’.
Haskell is used in many areas, including data science, finance, web development, and game development. It’s become popular for computational tasks that require immense processing power, such as deep learning, machine learning, artificial intelligence, image recognition, and more. It’s also been used to develop robust systems such as banking systems, network protocols, and large scale data analysis systems. Additionally, Haskell has been used to develop distributed systems which can handle billions of requests per second.
One popular example of a Haskell application is Cloud Haskell, which is a framework for building distributed systems. Cloud Haskell makes it easy to build distributed applications on multiple nodes that can communicate with one another. Other applications of Haskell include WebRTC, which provides real-time communication between browsers and applications; Quid, an analytics platform for financial markets; and front-end web development frameworks such as Yesod and Happstack.
Here is a simple example of Haskell code that takes a list of numbers and returns a new list with only the even numbers:
-- filter_even takes a list of numbers and returns a list containing only the even numbers
filter_even :: [Int] -> [Int]
filter_even xs = [x | x <- xs, mod x 2 == 0]
Use Cases
Examples of Use:
- Web Development: Haskell is becoming increasingly popular in web development circles. Popular open source web frameworks like Yesod and Snap are written in Haskell, making it possible to quickly and easily build dynamic websites with less code.
- Data Science: Haskell is a great language for working with large datasets. Libraries such as HMatrix and hmatrix-gsl enable developers to quickly perform operations on matrices and numerical data.
- Machine Learning: Many libraries are available for performing machine learning tasks in Haskell. These include hnn, which provides algorithms for neural networks, and hlearn, which provides a range of tools for supervised and unsupervised machine learning.
- Algorithmic Trading: Haskell is an ideal language for developing algorithmic trading systems. Libraries such as BookMap and Noft allow developers to quickly and easily develop complex financial trading strategies.
- Computer Graphics: Haskell is well suited for developing applications that require high performance computer graphics. For example, the library Glome.hs can be used to create 3D graphics.
- System Administration: Haskell is an excellent language for system administration tasks. Popular open source libraries such as Shelly and Turtle provide powerful tools for automating system processes.
Conclusion
Haskell is a powerful and versatile programming language that has found widespread use in many different areas. Its immutable data structures and lazy evaluation make it ideal for tasks that require immense processing power. It’s also been used to create robust systems such as banking systems and distributed applications.