C++
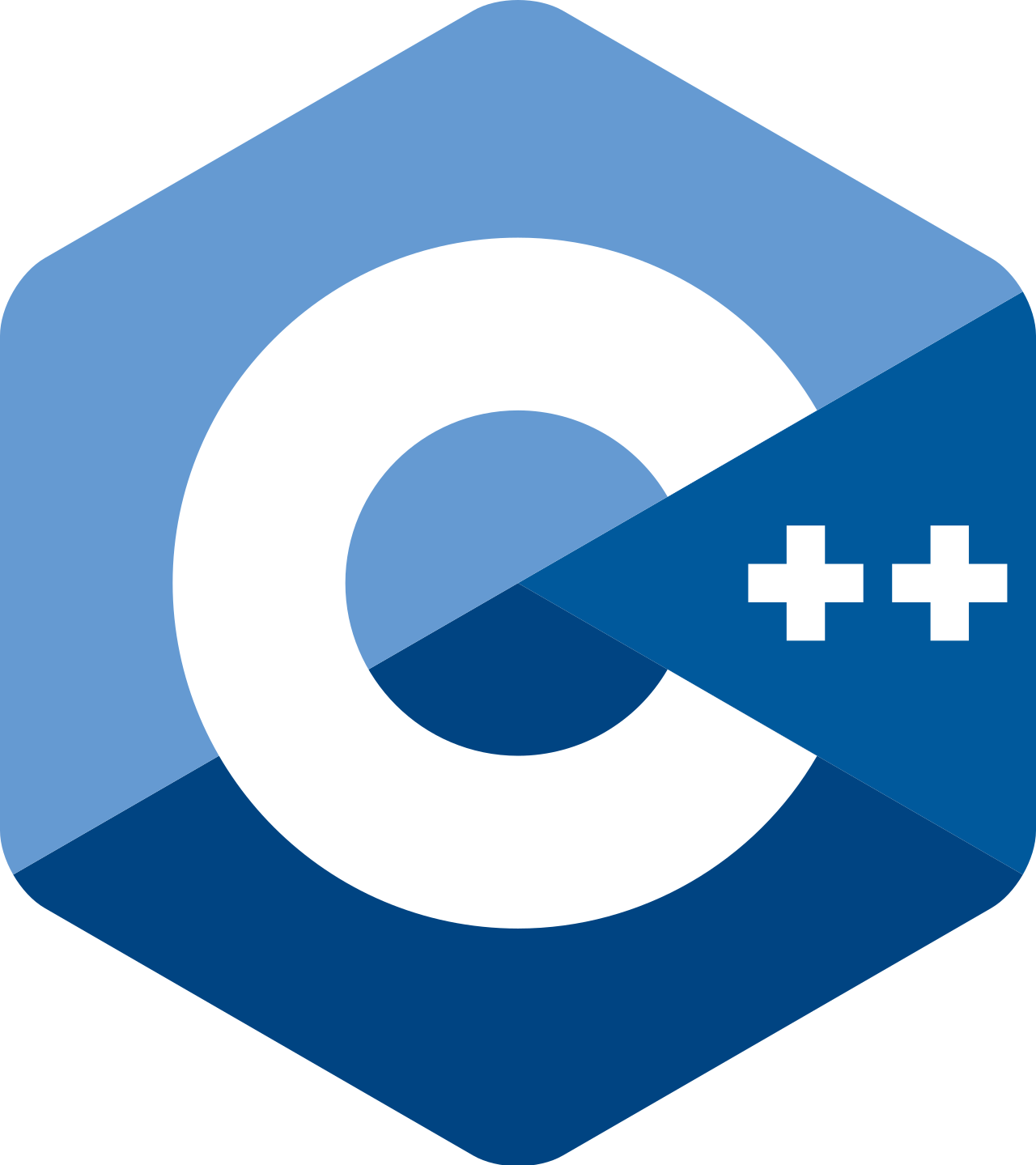
Introduction
C++ is general-purpose programming language, which was developed by Bjarne Stroustrup in 1980 at Bell Labs. It is used to develop a wide range of applications, such as games, microcontrollers, operating systems, visual applications, etc. C++ is an object-oriented programming language, which means it is based on the concept of objects and can be used to create complex data structures and algorithms. C++ is an object-oriented programming language built on the C programming language. It was developed by Bjarne Stroustrup at Bell Labs in 1979 and has since been updated several times. It is widely used in many fields, including software engineering, artificial intelligence, computer graphics, robotics, embedded systems, and other sciences.
History of C++
C++ was originally designed as an extension of the C language, with additional features specifically designed to make it easier to program object-oriented applications. Stroustrup’s goal was to create a language that “combines the power and efficiency of C with the flexibility and extensibility of object-oriented programming”. The first version of C++, known as “C with Classes”, was released in 1983. This initial version of the language was focused on providing a more powerful way to program compared to the existing C language. In 1985, the second version was released, called “C++”. This version added features such as templates, classes, and virtual functions, making it much easier to write object-oriented programs. By the 1990s, C++ had become one of the most popular programming languages in the world. While some objected to its reliance on object-oriented programming systems, many saw it as an improvement over traditional procedural languages like C. As the language continued to evolve, it became more powerful, adding features like exception handling and namespaces.
In the late 1970s, Bjarne Stroustrup created C++ at Bell Labs. In 1983, he released the first version of the language.
It incorporated some of the best features of the language C, a popular language for system programming at the time. In
addition to incorporating the useful features of C, C++ also added object-oriented concepts to the language, which
allowed users to define and manipulate objects.
In 1985, C++ was adopted by the American National Standards Institute (ANSI), making it the official standard for the
language. This made C++ the most widely used language for software development. Over the years, C++ has evolved and its
capabilities have grown exponentially. Today, C++ is the most popular language for developing a variety of applications,
from operating systems to embedded systems and web-based applications.
The C++ language was first released in 1985. The language was initially known as C with classes
and was eventually
standardized in 1998 by the International Organization for Standardization. Since then, it has become one of the most
popular and widely used programming languages in the world.
C++ is derived from the C language and is influenced by many other languages such as Simula 67 and ALGOL 68. The
development of C++ was driven by the need for performance and efficiency. The language was designed to provide
high-level abstractions while still being able to access the hardware directly.
It has evolved over time with the introduction of new features such as object-oriented programming, templates,
exceptions, and the standard template library. These features allow developers to create robust and powerful
applications with greater ease.
C++ was originally called C with Classes, as it began its life as an extension of the C language developed by Dennis
Ritchie in 1972. The modern version of C++ is still a departure from its predecessor, with the additions of
object-oriented programming, generic programming, and several other features that are essential in developing modern
software applications.
Despite its name, C++ does not directly involve C, but rather builds upon the syntax, design philosophy, and the
underlying concepts of the language. While the two can be used together, C++ does not rely on C for its syntax or
general usage, and is a complete language of its own.
Example of C++ Code
The following example is a simple program written in C++. This program will print the words “Hello World” to the console.
#include <iostream>
int main() {
std::cout << "Hello World" << std::endl;
return 0;
}
This example illustrates some of the basic features of the language. The first line of the program includes
the <iostream>
header, which contains the definitions for the std::cout
and std::endl
functions. These functions
are used to print data to the console.
The next line of the program defines the main()
function. This is the entry point for all C++ programs, and it must
always return an integer.
The code inside of the main()
block is what does the actual work. The code prints the text “Hello World” to the
console using the std::cout
and std::endl
functions. Finally, the program returns 0 to indicate that it ran
successfully.
##Conclusion
C++ is an incredibly powerful and versatile programming language. It has many features that make it well-suited for both large and small projects, and its object-oriented programming capabilities make it an excellent choice for developing complex applications. Its strong support for generic programming also makes it easier to write code that is both efficient and flexible.
C++ is used in various areas such as embedded systems and game development. Embedded systems use C++ for their low-level functions and game development requires it to create high-performance graphics and animation. Additionally, C++ is often used in scientific computing, artificial intelligence, machine learning, and image processing. The language is also used in web development, particularly for server-side development and large applications. Many web frameworks such as Django and Ruby on Rails are written in C++.
Hello World
This is a simple program that prints “Hello World!” to the console:
#include <iostream>
int main()
{
std::cout << "Hello World!";
return 0;
}
Fibonacci Series
This program prints out the first 20 numbers of the Fibonacci series:
#include <iostream>
using namespace std;
int main()
{
int n, first = 0, second = 1, next;
cout << "Fibonacci Series: ";
for (int i = 1; i <= 20; ++i) {
if(i == 1) {
cout << first << " ";
continue;
}
if(i == 2) {
cout << second << " ";
continue;
}
next = first + second;
first = second;
second = next;
cout << next << " ";
}
return 0;
}
Vector
This is an example of creating a vector of integers and adding some elements to it:
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> v;
// Adding elements using push_back()
v.push_back(10);
v.push_back(20);
v.push_back(30);
// Accessing elements using index
cout << "Accessing element at index 0: " << v[0] << endl;
cout << "Accessing element at index 1: " << v[1] << endl;
cout << "Accessing element at index 2: " << v[2] << endl;
return 0;
}
Features of C++
One of the most important features of C++ is its object-oriented programming capabilities. Object-oriented programming is a type of programming that makes it easier to work with large, complex programs. It works by grouping related data and functions into objects, which can then be used throughout the code. This makes it much easier to keep track of and manage different parts of the code. C++ also has strong support for generic programming. Generic programming is a type of programming that allows a programmer to write code that can be used with any type of data. This means that instead of writing code for each specific type of data, a programmer can write a generic function that can be used with any type of data. This makes it much easier to write code that is both efficient and flexible. Other important features of C++ include robust resource management, which allows a programmer to efficiently manage memory and other resources; strong exception handling, which makes it easier to handle errors in the code; and a large library of standard libraries, which provide the tools needed to create powerful programs.
C++ is an object-oriented programming language, which means it is built on the concept of objects that contain data and code. With object-oriented programming, developers can create complex data structures and algorithms to solve specific problems. The language also provides features such as templates and exceptions. The template feature allows programmers to define generic code that can be applied to various types of data, allowing them to write code more quickly and efficiently. The exception handling feature allows developers to handle errors in an orderly fashion, reducing the likelihood of crashing a program. C++ also supports the Standard Template Library (STL). The STL is a collection of powerful algorithms and data structures that can be used by a programmer to simplify their work. It makes it easier for developers to write efficient code and helps reduce the overall development time.
The C++ language has many features that make it an excellent choice for application development, such as:
Object-Oriented Programming
Object-oriented programming (OOP) is a model that helps developers organize their code into logical chunks and separate data from functionality. C++ supports this programming paradigm with the use of classes, objects, encapsulation, polymorphism, inheritance, and abstraction.
Memory Management
C++ provides several tools for controlling memory usage, such as free-store allocations, smart pointers, and manual memory management. This is important since memory leaks can cause unstable programs and, in some cases, system crashes.
Low-Level Operations
C++ enables developers to perform low-level operations, such as manipulating memory addresses, bitwise operations, and hardware manipulation. These low-level operations are often useful when a more specific operation needs to be performed than what is available in higher level languages.
Performance
C++ is a compiled language, which means the code is converted from a human-readable format (source code) into machine language that can be understood by the computer. Compiling the code allows for better performance since the compiler can optimize the code and produce faster results.
Portability
C++ programs can be easily ported to different operating systems since they are platform independent. This makes C++ a great choice for cross-platform development projects, as the code can be easily moved between operating systems without much modification.
Examples of Usages for C++
C++ is used all over the world to create various types of applications, from embedded systems to mobile applications. Here are a few examples of popular uses for C++:
Desktop Applications
Due to its performance, portability, and memory management, C++ can be used to build powerful desktop applications that are both reliable and efficient. Adobe, Microsoft, and Google are some of the well-known tech companies using C++ for building their desktop applications.
Web Browsers
Web browsers are often written in C++, as the language provides fine control over memory and can better utilize resources. Popular web browsers like Chrome and Firefox are written in C++, allowing them to provide a faster and smoother experience for users.
Embedded Systems
C++ is an ideal choice for embedded systems, as the language can be used to write microcode that is optimized for the hardware. This coupled with its low-level operations, memory management, and performance makes C++ the language of choice for embedded systems.
Video Games
Due to its speed and performance, C++ is commonly used to create video games. Many popular game engines, such as Unreal Engine, are written in C++, allowing developers to create high-performance games with lower overhead.
AI and Machine Learning
AI and machine learning algorithms rely heavily on performance and scalability for their success. C++ enables developers to write code that can take full advantage of the system’s resources and has proven to be an excellent choice for large-scale AI and ML projects.
Code Examples
Here is a simple code example in C++ that prints “Hello World” to the console:
#include <iostream>
int main() {
std::cout << "Hello World!" << std::endl;
return 0;
}
Output:
Hello World!
As you can see, C++ is an easy to understand and powerful language that can be used for a wide range of applications. From gaming to embedded systems, C++ is a versatile language that can help developers create efficient, solid applications.
Here is a basic “Hello World” program written in C++.
#include <iostream>
int main() {
std::cout << "Hello World!" << std::endl;
return 0;
}
Conclusion
C++ is an incredibly powerful language that is used to develop a variety of applications. Its versatility and efficiency make it the most popular language for many software developers. C++ is a great choice for developing both simple and complex applications, and it can be used to easily create highly optimized code.