Apache Cassandra
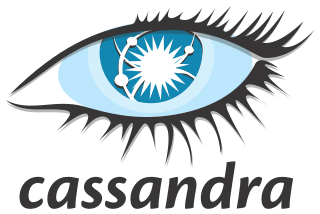
Apache Cassandra
Apache Cassandra is an open-source, distributed NoSQL database management system designed to handle large amounts of data across multiple commodity servers, providing high availability with no single point of failure. It was originally developed by Facebook and is now maintained by the Apache Software Foundation.
Cassandra was originally developed at Facebook in 2008. The project was then open-sourced and donated to the Apache Software Foundation in 2009, where it has become the most successful NoSQL system in the market today.
At its core, Cassandra is a masterless, shared-nothing, distributed database that can be deployed on multiple machines. It is a column-oriented database with no single point of failure and allows for massive horizontal scalability. This makes it ideal for applications with very large data sets that need to be highly available.
Cassandra also uses a replication strategy called “eventual consistency” which allows it to provide strong consistency in read/write operations while still remaining highly available. It is optimized for write-intensive applications and can handle up to tens of thousands of writes per second.
Cassandra is also highly flexible and supports a wide range of data types. It supports both relational and non-relational databases allowing for a hybrid approach to data storage.
History
Cassandra was originally developed at Facebook by Avinash Lakshman, Prashant Malik and Facebook engineer and former University of Toronto professor Eric Evans in 2007. Initially released as an open source project, Cassandra was developed using Facebook’s infrastructure for its inbox search feature, eventually becoming production-ready by 2008.
In 2009, Cassandra was made available to external users as a part of the Apache Incubator Program, which is a way for new open source projects to be hosted and formally recognized by the Apache Software Foundation.
In 2011, Cassandra graduated from the incubator program and became an official project of the Apache Software Foundation. Since then, Apache Cassandra has become increasingly popular as a distributed, robust, and fault-tolerant database management system.
The development of Apache Cassandra began in the late 2000s when the team at Facebook had a problem dealing with large volumes of data. They wanted a distributed storage system which could scale up or down based on the data volumes and store their data across commodity servers. The solution they created was called Cassandra, and it was released as an open source project in 2008.
Since then, Cassandra has been adopted by some of the biggest companies in the world including Netflix, Facebook, Twitter, Apple, eBay, and many others. Its popularity has only grown over the years, and it is now one of the most popular NoSQL databases in the world.
Features
Apache Cassandra has some unique features that set it apart from other NoSQL databases:
-
Scalable and Highly Available: Cassandra has the ability to scale outwards for increased storage capacity or performance by adding or removing nodes from the cluster. By design, it allows for replication of data to multiple nodes, thus offering high availability for the data.
-
Tunable Consistency: Cassandra offers a variety of consistency settings to suit different needs. The user can choose from strong, eventual, and quorum consistency models. On top of that, Cassandra also supports read and write repair algorithms that allow the user to configure higher levels of protection against data loss.
-
Flexible Data Model: Cassandra employs a flexible schema-free data model that supports different types of data. This allows the user to store structured, semi-structured, and unstructured data in the same cluster with minimal overhead.
-
High Performance: Cassandra is designed for high performance and low latency. It makes use of a data replication architecture that is based on a log-structured merge-tree (LSM-Tree). This architecture provides fast writes, compaction, and fast reads.
Usages
Apache Cassandra is widely used for a variety of applications. Some common use cases include:
- Internet of Things (IoT): Cassandra is used to store and analyze large amounts of data generated by connected devices.
- Real-time analytics: Cassandra is used to store and analyze streaming data in real-time.
- Messaging systems: Cassandra is used to power messaging platforms such as WhatsApp and Instagram.
- Payment processing: Cassandra is used for secure payment processing for companies such as PayPal.
- Ad targeting: Cassandra is used to gather and store data on user behavior and interests for more effective ad targeting.
Code Examples
The following code examples demonstrate how to connect to and query a Cassandra database. The examples use the Java DataStax driver.
Establishing a Connection
The following example demonstrates how to create a Cluster
instance and establish a connection to a Cassandra cluster.
Cluster cluster=Cluster.builder()
.addContactPoint("127.0.0.1")
.build();
Session session=cluster.connect();
Querying a Table
The following example demonstrates how to query a table called users
and print out the results.
ResultSet results=session.execute("SELECT * FROM users");
for(Row row:results){
System.out.println("name: "+row.getString("name"));
System.out.println("age: "+row.getInt("age"));
System.out.println("city: "+row.getString("city"));
}
Inserting a Row
The following example demonstrates how to insert a row into the users
table.
session.execute("INSERT INTO users(name, age, city) VALUES ('John', 25, 'New York')");
Updating a Row
The following example demonstrates how to update a row in the users
table.
session.execute("UPDATE users SET city = 'Boston' WHERE name = 'John'");
Deleting a Row
The following example demonstrates how to delete a row from the users
table.
session.execute("DELETE FROM users WHERE name = 'John'");
Apache Cassandra is used by many large organizations because of its ability to handle large amounts of data. Here are some examples of how Apache Cassandra is being used:
- Twitter uses Cassandra for its core data store, storing users’ tweets, followers, and other user-generated data.
- Netflix uses Cassandra to store metadata about its movies and TV shows, allowing customers to quickly find titles they are interested in watching.
- eBay uses Cassandra to store its product catalog, allowing customers to quickly search for items they are interested in buying.
Features
Apache Cassandra offers a number of features that make it an attractive option for organizations dealing with big data.
The most attractive feature is its scalability. Cassandra can easily scale up or down depending on the amount of data being stored. This means that it can easily accommodate large datasets and can also handle sudden influxes of data.
Another important feature is its high availability. Cassandra ensures that there is no single point of failure and all nodes in the cluster are always available. This ensures that the data is always safe and accessible.
Cassandra also offers support for various programming languages such as Java, Python, and C++. This makes it easy for developers to work with Cassandra.
Finally, Cassandra provides powerful tools for managing the data such as backup and restore, replication, and data compression. These features help make sure that the data is always up-to-date and secure.
Setting Up Cassandra
First, you need to install Cassandra on your machine. You can do this using a package manager like apt-get or yum. Once it is installed, you can start the Cassandra service by running the following command:
sudo service cassandra start
Creating a Keyspace
A keyspace is a namespace used to group related tables in Cassandra. To create a keyspace, we use the CREATE KEYSPACE
command. For example, if we want to create a keyspace called ‘my_keyspace’, we can use the following command:
CREATE KEYSPACE my_keyspace WITH REPLICATION = { 'class' : 'SimpleStrategy', 'replication_factor' : 1 };
Creating Tables
Now that we have a keyspace, we can create tables within it. To create a table, we use the CREATE TABLE
command. For
example, if we want to create a table called ‘employees’ in our ‘my_keyspace’ keyspace, we can use the following
command:
CREATE TABLE my_keyspace.employees (name text PRIMARY KEY, age int, address text);
Inserting Data
Once we have our table, we can insert data into it. To insert data, we use the INSERT INTO
command. For example, if we
want to insert a new employee into our ‘employees’ table, we can use the following command:
INSERT INTO my_keyspace.employees (name, age, address) VALUES ('John Doe', 25, '123 Main St.');
Usage
Cassandra is used by a number of companies for a variety of use cases. Some of the most common include:
- Real-time analytics - Cassandra is used to store and analyze real-time data from web, mobile, IoT devices and more. Companies like Netflix, Expedia, Facebook, and eBay are using Cassandra for real-time analytics.
- Content delivery and caching – Cassandra is used in content delivery networks (CDNs) to cache static or dynamic web content. Companies like Akamai and Limelight Networks use Cassandra to deliver content quickly and reliably.
- Online gaming – Cassandra is used to store and serve player data with low latency. Companies like Zynga, EA, and Kabam rely on Cassandra to ensure their players have the best experience possible.
- Ecommerce – Cassandra is used to store and process high volumes of orders and customer data. Companies like Etsy and eBay have adopted Cassandra to power their online stores.
- Logging and auditing – Cassandra is used for storing and auditing user activity data and system logs. Companies like Twitter and Yahoo use Cassandra for log management.
Code Example
This is an example of setting up a basic Cassandra cluster using Docker.
First, you will need to install Docker on your machine. Once installed, run the following command to pull the Cassandra image from Docker Hub:
docker pull cassandra
Once the image has been pulled, you can now start the Cassandra container using the following command:
docker run --name my_cassandra -d cassandra
The Docker Cassandra container will now be running in the background. You can check the status of the container by running the following command:
docker ps
You can also use the Docker CLI to connect to the Cassandra shell:
docker exec -it my_cassandra cqlsh
Once connected to the Cassandra shell, you can now start creating keyspaces and tables. To create a keyspace, run the following command:
CREATE KEYSPACE my_keyspace WITH REPLICATION = {'class': 'SimpleStrategy', 'replication_factor' : 3};
This will create a keyspace called “my_keyspace” with a replication factor of 3. To create a table, run the following command:
CREATE TABLE my_table (id int PRIMARY KEY, name text);
This will create a table called “my_table” with two columns: “id” and “name”.
Finally, you can insert some records into the table by running the following command:
INSERT INTO my_table (id, name) VALUES (1, 'John Doe');
This will insert a record into the table with an ID of 1 and a name of “John Doe”.
Once your data is in the table, you can query it by running the following command:
SELECT * FROM my_table;
This will return all of the records in the table. You can also query specific records by adding a WHERE clause:
SELECT * FROM my_table WHERE id = 1;
This will return the record with an ID of 1.
Cassandra is used in a variety of applications, ranging from social networks to large enterprise applications. Some common use cases include:
-
Fraud Detection: Cassandra can store large amounts of data and provide fast access to records required for fraud detection.
-
Real-time Analytics: Cassandra can handle large amounts of data and provide low-latency access to analytics.
-
Customer Relationship Management (CRM): Cassandra can store customer records and quickly access them when needed.
-
Content Delivery Network (CDN): Cassandra can quickly deliver content to users around the world.
Cassandra is written in Java, so most code examples will use this language. Here is an example of a simple table creation using Cassandra’s CQL syntax:
CREATE TABLE test
(
id int PRIMARY KEY,
name varchar
);
And here is an example of inserting data into the table:
INSERT INTO test (id, name)
VALUES (1, 'John Doe');
You can also use Cassandra’s Java API to query and manipulate data. Here is an example of how to retrieve a row from a table:
ResultSet rs=session.execute("SELECT * FROM test WHERE id = 1");
Row row=rs.one();
String name=row.getString("name");
Features
-
High Availability: Cassandra is designed to be highly available and durable, so that if a node fails or the cluster loses connectivity, data continues to be available and transactions stay consistent.
-
Scalability: Cassandra scales linearly and can handle large amounts of data. It’s also easy to add new nodes to the cluster as needed.
-
Flexible Data Model: The Cassandra data model can handle a variety of data structures, including key/value pairs, wide columns, and documents.
-
Low Latency: Cassandra is designed for low-latency operations.
Architecture
Cassandra has a unique architecture that allows it to offer many of its features. At the highest level, Cassandra is composed of nodes, clusters, and keyspaces.
Nodes are the individual machines that make up the cluster. They can exist in different physical locations, but together form the cluster.
A keyspace is a logical container for the data, similar to a database. It can contain multiple tables which store the actual data.
The data is split into a series of partitions, and each node holds a replica of each partition. This allows the data to be distributed across the cluster and makes it more resilient to failures.
When a query is run, the coordinator node sends it to the replicas responsible for the correct partitions. The replicas then respond with the results, and the coordinator merges them into a single response.
Conclusion
Apache Cassandra is an open source distributed database management system that is designed to handle large amounts of data across many commodity servers. It provides scalability, high availability, support for multiple programming languages, and powerful data management tools. Organizations such as Twitter, Netflix, and eBay are among some of the largest users of Apache Cassandra, and it is continuing to be adopted by more and more businesses.
Apache Cassandra is an open source, distributed database system designed for scalability and high-availability. It’s a NoSQL solution that supports a wide range of applications, from social networks to large enterprise applications. Its features include its ability to handle large amounts of data, its high availability, and its scalability. It also has a unique architecture that allows it to offer many of its features, such as its data model and low-latency operations. Examples of uses for Cassandra range from fraud detection to customer relationship management. And code examples in Java demonstrate how to use Cassandra’s CQL syntax and Java APIs to query and manipulate data.