Android
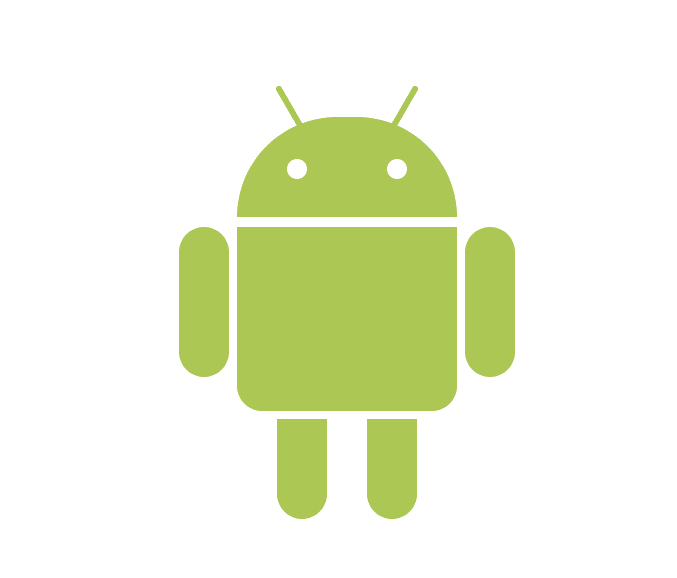
What is Android?
Android is one of the most popular mobile operating systems in the world, with more than 2 billion active users. It was first released in 2008 and has since gone through numerous major versions, each with its own set of features and capabilities. It provides users with a wealth of features, including multitasking and background services, web browsing, media playback, and location-based services. Moreover, it provides developers with a powerful platform for creating apps for a variety of devices and platforms. Android is an open source software stack for mobile devices including smartphones, tablets, and now wearables, televisions, and cars. It consists of an operating system, middleware and key applications. Developed by Google and the Open Handset Alliance, Android offers an open source platform and application framework for mobile devices. Android is a mobile operating system based on the Linux kernel, designed primarily for touchscreen devices such as smartphones and tablets. Google has been the primary driving force behind Android’s development since it was first released in 2007. It is now the world’s most popular mobile operating system, powering more than two billion active devices. As a result, Android is a highly customizable platform and developers can develop apps and services for it with relative ease. This has led to a wide variety of apps and services being available for Android, ranging from enterprise solutions to everyday utility apps.
History
Android was developed in response to Apple’s iOS, which had become increasingly popular in the late 2000s. The Android team was originally formed in 2003 as part of the Open Handset Alliance, which was a consortium of companies that included Google, HTC, Motorola, Samsung, and other technology companies. In 2007, the first Android-powered device was released: the T-Mobile G1, which was the first mass-market smartphone to feature a color display, touch screen, and GPS. Since then, Android has grown exponentially in popularity and is now the most popular mobile operating system in the world. It has also come to power a wide variety of devices, from smart TVs and watches, to cars and even refrigerators. Additionally, it has been adopted by a wide variety of companies, from phone manufacturers to media giants like Sony and Spotify. Android is a mobile operating system developed by Google and based on Linux, first released in 2008. It was initially designed for touchscreen mobile devices such as smartphones and tablets, but has become one of the most widely used mobile operating systems in the world. Nowadays, Android runs on more than 2 billion devices and has the largest installed base of any operating system.
When the original Android source code was released in 2008, the world was a different place. Google had just gone public, the first Android phone (the G1) had yet to be released, and Apple’s iPhone was just starting to make waves. But since then, it’s been a wild ride.
Since its inception, Android has grown significantly and is now the most widely used mobile platform in the world, even surpassing Apple’s iOS. Over the years, Google, along with its partners in the Open Handset Alliance, have worked hard to improve both the core Android platform as well as the applications that run on it. The latest version of Android ( Lollipop) is particularly noteworthy, as it brings a multitude of new features and design elements to the OS.
In addition to the core OS, Google and its partners have also developed a variety of other Android-based projects. These include the Android Wear platform for smartwatches, Android Auto for automobiles, Android TV for televisions, and Brillo, which is intended to bring Android to the Internet of Things.
In 2003, Android Inc., was founded by Andy Rubin, Rich Miner, Nick Sears, and Chris White to develop, in Rubin’s words, “smarter mobile devices that are more aware of its owner’s location and preferences.”
Google acquired Android Inc. in 2005 and they released the first version of the Android OS, Alpha, later that year. The first commercial version, Android 1.0, was released on September 23, 2008. Since then, the Android operating system has gone through multiple major releases and updates, with the current version being Android 9.0 ‘Pie’, which was released on August 6, 2018.
Examples of Usage
Android is used in a wide variety of scenarios, from consumer electronics to enterprise solutions. Some of the most popular uses of Android include:
- Media streaming: Android is used by companies like Netflix, Hulu, and Spotify to power their media streaming services. Additionally, it is used by TV manufacturers to create streaming capabilities for their devices.
- Mobile apps: A large majority of all the apps available on Google Play are created for Android devices. These include everything from games to utility and productivity apps.
- Automotive solutions: Many car manufacturers use Android to power their in-car infotainment systems. This allows them to offer features such as maps, music streaming, and advanced voice control.
- Home automation: Android is used by smart home devices such as thermostats, security systems, and lightbulbs, allowing users to control them using their phones.
- Enterprise solutions: Companies such as Salesforce, Oracle, and SAP use Android to power their enterprise solutions. This allows businesses to create custom solutions that run on Android devices.
Code Example
Android applications are written primarily in the Java programming language. Here is a simple example of an Android application that displays a “Hello World” message:
import android.app.Activity;
import android.os.Bundle;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
This code will create an app with a single Activity (the main screen) and display a “Hello World” message. To take full advantage of Android’s features, developers can use additional APIs, such as the Android Support Library or Google Play Services.
Here is some sample code which demonstrates how to create an Android application:
public class MyActivity extends Activity {
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my);
// Get a handle to the button in the layout
Button myButton = (Button)findViewById(R.id.my_button);
// Set an OnClickListener on the button
myButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Do something when the button is clicked
}
});
}
}
And here is some sample code for an Android Wear application:
public class MyWatchActivity extends WearableActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my_watch);
// Get a handle to the button in the layout
Button myButton = (Button)findViewById(R.id.my_button);
// Set an OnClickListener on the button
myButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Do something when the button is clicked
}
});
}
}
The following code example shows how to create a simple application for Android using the Android SDK.
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme">
<activity
android:name=".MainActivity"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
</activity>
</application>
This example creates an application called ‘MainActivity’ which will be launched when the application is opened. It also defines the application’s icon, label, and theme, which will be used throughout the application.
Origins
The development of Android began in 2003, when Google acquired Android Inc., a small startup led by Andy Rubin, who had previously been involved in the development of Danger Inc.’s T-Mobile Sidekick smartphone. The company was developing a technology platform that would allow mobile devices to run multiple applications at once, something that was unheard of at the time. In 2005, Google released the Android Developer Challenge, a competition to help develop innovative applications for the platform. The challenge saw a huge response and Google eventually awarded $1 million to the winning developers. This helped spur further development of the Android platform and its associated tools and libraries.
Components
The Android operating system is composed of several distinct components, each with its own purpose and function. The main components are the Linux kernel, which provides the core functions of the operating system; the application framework, which provides the basic structure for creating apps; and the Dalvik virtual machine, which provides a runtime environment for running Android apps.
The Android SDK (Software Development Kit) is a set of tools and libraries that enable developers to create Android applications. The SDK contains a range of APIs (Application Programming Interfaces) that allow developers to access the underlying hardware and software features of an Android device.
Versions
Over the years, Google has released a number of major versions of Android, each with its own distinct features and capabilities. The first major version, released in 2008, was Android 1.5 (Cupcake). This version introduced a number of new features, such as support for third-party application markets and customizable home screens.
In the following years, Google released a number of new versions of Android, including Android 2.2 (Froyo); Android 3.0 (Honeycomb); Android 4.0 (Ice Cream Sandwich); Android 4.1 (Jelly Bean); Android 4.4 (KitKat); Android 5.0 ( Lollipop); Android 6.0 (Marshmallow); and Android 7.0 (Nougat). Android 8.0 (Oreo) was released in 2018, with Android 9.0 (Pie) following in 2019.
Features
Android provides a variety of features to users, some of which are built into the operating system itself and some of which are available through third-party application markets. Some of the most commonly used features include support for multitasking and background services, web browsing, media playback, location-based services, voice input and output, and notifications.
For developers, Android offers a powerful development platform that includes a wide range of APIs and libraries, allowing them to easily create applications for a variety of devices and platforms. Android also supports a wide range of programming languages, including Java, C++, Kotlin, and HTML5.
Examples of Usage
Android Apps
The core of Android is built around applications, or apps, that allow users to do everything from checking news and weather to playing games and streaming movies and music. As of 2015, there were over 1.5 million apps available in the Google Play store, making it one of the largest app stores in the world.
Developers who wish to create apps for Android can do so using Java or Kotlin, and tools such as the Android Software Development Kit (SDK). The SDK provides all of the necessary tools to build, test, and deploy apps for Android devices.
Android Wear
Android Wear is a version of Android that has been specifically designed to run on wearable devices such as smartwatches. It is based on the same Android OS as mobile devices, but has been modified to fit the form factor and provide a different experience.
The main differences between Android Wear and the regular version of Android are the interface and the types of apps that can be installed. Android Wear is focused on providing quick glances at information and controlling other devices, while regular Android phones are better suited for more in-depth interactions and activities.
Android Auto
Android Auto is an in-car connectivity system based on a modified version of Android. It is designed to make it easy for drivers to access their favorite apps and services while driving, such as phone calls, navigation, music, and messaging.
Android Auto works by connecting an Android device to a compatible car dashboard. When connected, the car’s dashboard will display Android Auto’s user interface, allowing drivers to access apps and services without taking their eyes off the road. Additionally, compatible apps have been optimized for use with Android Auto, allowing for a more seamless experience.
Android TV
Android TV is a version of Android designed specifically for use on television sets. Like with Android Wear, it is based on the same Android OS as phones and tablets, but has been optimized for use on a bigger screen.
Android TV runs on a variety of platforms, including smart TVs, set-top boxes, and game consoles. Users can access popular streaming services such as Netflix, Hulu, and YouTube, as well as a plethora of other apps and games. Additionally, several manufacturers have created Android TV-powered streaming sticks and boxes, allowing users to turn any TV into a Smart TV.
Brillo
Google recently unveiled Brillo, a platform designed to bring Android to the Internet of Things. Brillo is based on a modified version of Android and is intended to make it easier for developers to create applications for connected devices.
Brillo is designed to be lightweight, so that it can be used on devices such as connected light bulbs and door locks. Access to some of Android’s core components, such as the Activity Manager, is not included, but the platform does come with support for Bluetooth Low Energy and Wi-Fi.
Key Components
Android is split into four main components:
-
Linux Kernel - Android relies on the Linux kernel for core system services such as security, memory management, process management, network stack, and driver model.
-
Android Runtime (ART) - ART is the primary runtime which replaces Dalvik Virtual Machine (DVM) which is used in earlier versions of Android. ART is a cross-platform runtime which supports the compilation and execution of multiple programming languages.
-
Libraries - It includes a set of C/C++ core libraries which are exposed to developers through the Android application framework. These libraries provide most of the functionality available in the core libraries of Java language.
-
Application Framework - This layer provides abstractions for core mobile device functionality such as audio, locations, graphics, data storage etc. Application framework enables high-level access to various device capabilities and its services which make it easier for developers to write applications.
Adoption
Android is the world’s most popular mobile operating system with over 2 billion active users worldwide. It is largely used on smartphones, tablets, and other mobile devices. It is also used on televisions, cars, and even wristwatches.
The majority of Android usage falls into the categories of:
-
Communication - Android devices are used as a tool for communication, with applications such as WhatsApp, Viber, Skype and Hangouts.
-
Entertainment - Android devices are used for entertainment purposes, with applications such as YouTube, Netflix, Spotify and Facebook.
-
Productivity - Android devices are also used for productivity, with applications such as Google Drive, Microsoft Office, Dropbox, and Evernote.
-
Banking - Many banks have their own Android applications which allow customers to access their accounts and make payments.
-
Education - Android devices are used in education, with applications such as Khan Academy and DuoLingo providing educational opportunities to users.
Conclusion
Android is a powerful mobile operating system, with a wide range of applications and uses. It has become the most widely used mobile operating system, with over 2 billion active users worldwide. Android is an open source platform which enables developers to create innovative applications and experiences for their users. Additionally, Android can be used on a variety of devices, ranging from smartphones to television sets, cars, and even wristwatches. With its versatile applications and features, Android has revolutionised the way we communicate and experience the world.