.NET
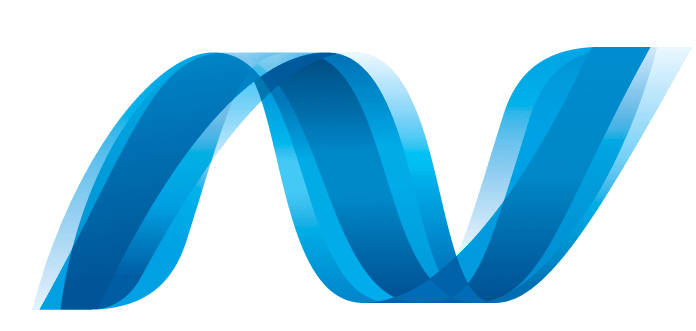
Introduction
.NET (dot-net) is a software framework developed by Microsoft that runs primarily on Microsoft Windows. It was designed to create applications with a common language runtime and components, and it has been used to build a wide variety of computer software, from web services, desktop applications and mobile applications. .NET helps developers build the following types of applications:
- Web applications and web services
- Desktop applications
- Mobile applications
- Console applications
History
The .NET Framework was released in 2002 and was the first development framework from Microsoft that combined the power of the Common Language Runtime (CLR) and the language-independent object-oriented programming model.
Since its first release, the .NET Framework has evolved and undergone several major version changes. The most recent version, version 4.8, was released in April 2019 and contains a number of new features, including support for Windows 10, ARM64, and improved security.
The .NET Framework has been widely adopted by developers, who use it to create a vast array of different types of applications. Applications created with the .NET Framework can be written in any number of languages, including C#, Visual Basic, F#, and C++.
What Is The .NET Framework?
At its core, the .NET Framework is a set of libraries and components intended to help programmers quickly and efficiently build applications. The .NET Framework provides both a runtime environment and a suite of development tools.
The .NET Framework includes a common language runtime (CLR), which manages the execution of code written in any language targeting the .NET Framework. The CLR also provides a rich set of features such as garbage collection, type safety, and exception handling.
The .NET Framework also includes a large library of pre-built components and classes that can be used in applications. This library is referred to as the Base Class Library (BCL). The BCL provides a wide range of functionality including data access, networking, XML parsing, graphics, cryptography, and more.
Advantages of the .NET Framework
The .NET Framework offers developers a number of advantages.
-
Language Interoperability: Developers can use any one of the multiple languages supported by the .NET Framework to develop their applications. This eliminates the need to learn a different language for each platform they target.
-
Simplified Development: The .NET Framework provides a number of useful features and classes that make development easier. This includes features like garbage collection, type safety, and exception handling.
-
Security: The .NET Framework also includes a number of built-in security features that can help protect an application from malicious code.
-
Cross-Platform Support: The .NET Framework can be used to create applications that are able to run on multiple platforms, including Windows, macOS, and Linux.
Code Examples
The following code is an example of a simple application written in C# that displays “Hello World!” to the console.
using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello World!");
}
}
The following code is an example of a simple web application written in C#. This application will return the text “Hello World!” in the browser.
using System;
using System.Web;
public class MyHandler : IHttpHandler
{
public void ProcessRequest(HttpContext context)
{
context.Response.Write("Hello World!");
}
public bool IsReusable
{
get { return false; }
}
}
The following code is an example of a Windows Presentation Foundation (WPF) application written in C#. This application will display a simple window that says “Hello World!”
<Window x:Class="MyProject.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="My Window">
<Grid>
<TextBlock Text="Hello World!" />
</Grid>
</Window>
Examples of usage
The .NET framework is widely used in many different industries, from finance and banking to healthcare, retail and manufacturing. It’s also used for web development, creating mobile apps, machine learning and artificial intelligence, cloud computing and many other types of software.
For example, Microsoft Azure is built using .NET and it powers many large-scale applications and services, such as Office 365 and Dynamics 365. Bank of America also uses .NET for its online banking platform, and Intel uses it to power their data analytics solutions.
Here are some other examples of .NET usage:
- Adobe Creative Cloud uses .NET for its content management system.
- Google Maps and Apple Maps both use .NET components in their web applications.
- Spotify relies heavily on .NET for its music streaming service.
- Walmart uses the framework for its e-commerce platform.
- Honda’s connected car platform is powered by the .NET framework.
The following code snippets demonstrate how to create a simple “hello world” program using the .NET framework.
The first example is written in C#:
using System;
public class Program
{
public static void Main()
{
Console.WriteLine("Hello World!");
}
}
The second example is written in Visual Basic:
Module HelloWorld
Sub Main()
Console.WriteLine("Hello World!")
End Sub
End Module
The third example is written in F#:
printfn "Hello World!"
There are many different ways that .NET can be used. Here are a few examples:
-
Web Development: .NET can be used to develop web applications, websites, and services. It provides a range of tools and features that make it easy to create interactive, responsive, and secure web applications.
-
Mobile Development: .NET can be used to develop native mobile applications for iOS and Android. The framework includes tools that make it easy to integrate databases, access hardware, and incorporate cloud services.
-
Desktop Applications: .NET can also be used to create desktop applications for Windows, macOS, and Linux. It includes powerful GUI elements and libraries that allow developers to build powerful, feature-rich desktop applications.
-
Games: .NET can also be used to create games, ranging from simple 2D games to complex 3D games. It includes a range of features, such as support for DirectX, OpenGL, OpenAL, and physics engines.
Here is an example of a .NET application written in Visual Basic.NET:
Sub Main()
Dim x as Integer = 10
Dim y As Integer = 15
Console.WriteLine("x + y = " & (x + y))
End Sub
And here is an example of the same application written in C#:
using System;
public class Program
{
public static void Main()
{
int x = 10;
int y = 15;
Console.WriteLine("x + y = " + (x + y));
}
}
Here are some examples of .NET code:
C#
// Hello World program in C#
public class HelloWorld
{
public static void Main()
{
System.Console.WriteLine("Hello World");
}
}
Visual Basic .NET
' Hello World program in VB.NET
Module HelloWorld
Sub Main()
System.Console.WriteLine("Hello World")
End Sub
End Module
F#
// Hello World program in F#
open System
[<EntryPoint>]
let main _ =
printfn "Hello World"
0
IronPython
# Hello World program in IronPython
print("Hello World")
History
The .NET framework was first released in 2002 as part of the Windows XP operating system. It was designed to provide a more efficient programming model than traditional Windows applications, and to make it easier for developers to write secure, reliable code. Over the years, the framework has gone through several major versions, with the latest being .NET 5, which was released in November 2020. This version includes improved performance, better cross-platform support and a unified development experience across Windows and Linux. The main languages used on the framework are C#, F# and Visual Basic. Other languages such as Python, JavaScript, Rust and C++ are also available.
Benefits of .NET
.NET provides many benefits to developers, such as:
- Cross-platform: With .NET, developers can write code that is compatible with any platform. This means that applications can be written for Windows, Mac, Linux, and more, without the need to rewrite code for each platform.
- Robust library of components: .NET includes an extensive library of code components that developers can use to add functionality to their applications. These components are also open source, which means that developers can modify and improve them as needed.
- Scalable and secure: .NET allows developers to scale their applications easily, and the framework includes a robust security system. This ensures that applications built with .NET are secure and reliable.
- Easy to learn: The .NET Framework is designed to be easy to use and understand, and it includes a range of tutorials and documentation that can help developers learn the basics quickly.
Summary
.NET offers a number of benefits, including:
- Increased productivity: With its unified platform and multiple languages, developers can quickly create robust applications and services without needing to learn multiple languages and tools.
- Enhanced security: .NET utilizes code access security, authentication, and authorization to protect applications from malicious attacks.
- Cross-platform support: .NET Core runs on Windows, macOS, and Linux, allowing developers to create applications for multiple platforms.
- Open-source: .NET Core is an open-source project, meaning that developers can contribute to the project, submit bug reports, and access the full source code.